previous episode
Octave programming, making choices, next episode.
Overview Teaching: 30 min Exercises: 0 min Questions How can programs do different things for different data values? Objectives Construct a conditional statement using if, elseif, and else Test for equality within a conditional statement Combine conditional tests using AND and OR Build a nested loop
Our previous lessons have shown us how to manipulate data and repeat things. However, the programs we have written so far always do the same things, regardless of what data they’re given. We want programs to make choices based on the values they are manipulating.
The tool that Octave gives us for doing this is called a conditional statement , and it looks like this:
The second line of this code uses the keyword if to tell Octave that we want to make a choice. If the test that follows is true, the body of the if (i.e., the lines between if and else ) are executed. If the test is false, the body of the else (i.e., the lines between else and end ) are executed instead. Only one or the other is ever executed.
Conditional statements don’t have to have an else block. If there isn’t one, Octave simply doesn’t do anything if the test is false:
We can also chain several tests together using elseif . This makes it simple to write a script that gives the sign of a number:
One important thing to notice in the code above is that we use a double equals sign == to test for equality rather than a single equals sign. This is because the latter is used to mean assignment. In our test, we want to check for the equality of num and 0 , not assign 0 to num . This convention was inherited from C, and it does take a bit of getting used to…
We can also combine tests, using && (and) and || (or). && is true if both tests are true:
|| is true if either test is true:
In this case, “either” means “either or both”, not “either one or the other but not both”.
True and False Statements 1 and 0 aren’t the only values in Octave that are true or false. In fact, any value can be used in an if or elseif . After reading and running the code below, explain what the rule is for which values that are considered true and which are considered false. a. if '' disp('empty string is true') end b. if 'foo' disp('non empty string is true') end c. if [] disp ('empty array is true') end d. if [22.5, 1.0] disp ('non empty array is true') end e. if [0, 0] disp ('array of zeros is true') end f. if true disp('true is true') end
Close Enough Write a script called near that performs a test on two variables, and displays 1 when the first variable is within 10% of the other and 0 otherwise. Compare your implementation with your partner’s: do you get the same answer for all possible pairs of numbers?
Another thing to realize is that if statements can be also combined with loops. For example, if we want to sum the positive numbers in a list, we can write this:
With a little extra effort, we can calculate the positive and negative sums in a loop:
We can even put one loop inside another:
Nesting Will changing the order of nesting in the above loop change the output? Why? Write down the output you might expect from changing the order of the loops, then rewrite the code to test your hypothesis. Octave (and most other languges in the C family) provides in-place operators that work like this: x = 1; x += 1; x *= 3; Rewrite the code that sums the positive and negative values in an array using these in-place operators. Do you think that the result is more or less readable than the original?
Currently, our script analyze.m reads in data, analyzes it, and saves plots of the results. If we would rather display the plots interactively, we would have to remove (or comment out ) the following code:
And, we’d also have to change this line of code, from:
This is not a lot of code to change every time, but it’s still work that’s easily avoided using conditionals. Here’s our script re-written to use conditionals to switch between saving plots as images and plotting them interactively:
Key Points Use if and else to make choices based on values in your program.
Technology eXplorer
- Octave:2> Conditional Execution
- April 19, 2020
Purnendu Kumar
To control the flow operations in an algorithm, one must be able to verify some conditions and act accordingly. Other-times it might be required to perform same task repeatedly until certain conditions are met. Conditional execution are the backbone of any algorithm. In this article we will look through common conditional and looping expressions. We encourage readers planning to learn Octave/Matlab to try the exercises marked as “ Ex “ along with the examples used in this article.

if Statement
“ if ” statement is the most common conditional execution block in any algorithm. It executes the part of block only the boolean expression provided after “ if ” is true. Boolean expression after “ if “is known as expression. To end the loop, “ end ” statement is used.
“ if ” statement can also be used with an “ else ” statement and thus some action can be performed in both cases either the condition is true or false.
It is often the case algorithm night require to verify more than one condition and then do accordingly. in such case “ elseif ” condition can be used as many time required.
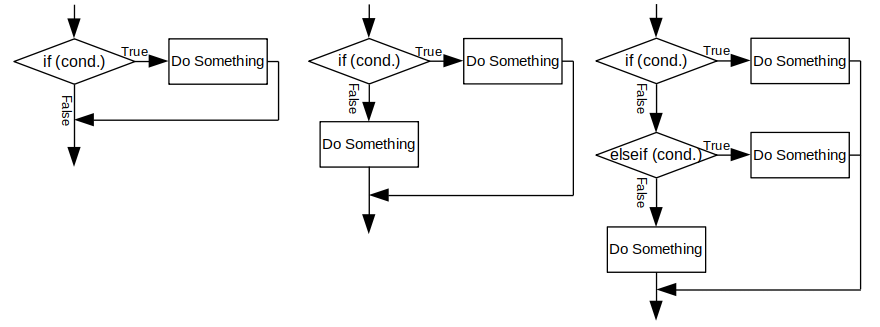
switch Statement
A “ switch ” is a series of checks on a variable. outcome of this statement is comparable to “ if .. elseif .. else “, but execution is not. In “ switch case ” execution happens as per variable, multiple condition checks are not done.
while Statement
“ while ” is a looping statement with boolean condition. “ while ” loop keeps on executing the same block of code until the condition is true. while loop is preferable when the number of iteration is not known.
Loop can be terminated in mid also using “ break ” statement.

for Statement
When number of iteration is known and steps are equidistant, for loop is preferable for algorithm to work efficiently.
Colon operator is preferred choice in for loop, but not the only way to use a for loop.
After learning the conditional execution along with scripting, try to create a solution for any known problem and verify your script. keep learning, keep growing. Let us know your query and questions in comment section.

- GNU Octave – Scientific Programming Language
- Octave:1> Getting Started
- Octave:1> Variables and Constants
- Octave:1> Expressions and operators
- Octave:1> Built-In Functions, Commands, and Package
- Octave:2> Vectors and Matrices Basics
- Octave:2> Scripts and Algorithm
- Octave:2> Functions
- Octave:3> File Operations
- Octave:3> Data Visualization
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Facebook (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to email a link to a friend (Opens in new window)
- Click to print (Opens in new window)
- Click to share on Tumblr (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Pocket (Opens in new window)
- Click to share on Telegram (Opens in new window)
Tags: GNU Octave Matlab Conditional Execution Loop if while switch
Purnendu is currently working as Senior Project Engineer at QuNu Labs, Indias only Quantum security company. He has submitted his thesis for Masters (MS by research 2014-17) in electrical engineering at IIT Madras for doing his research on “constant fraction discriminator” and “amplitude and rise-time compensated discriminator” for precise time stamping of Resistive Plate Chamber detector signals. In collaboration with India Based Neutrino observatory project, he has participated in design and upgrade of FPGA-based data acquisition system, test-jig development, and discrete front-end design. After completion of his bachelors in Electrical Engineering (Power and Electronics), he was awarded Junior Research Fellowship at Department of Physics and Astro-physics, University of Delhi under same (INO) project. His current interest is in high-speed circuit design, embedded systems, IoT, FPGA implementation and optimization of complex algorithms, experimental high-energy physics, and quantum mechanics.
You may also like...
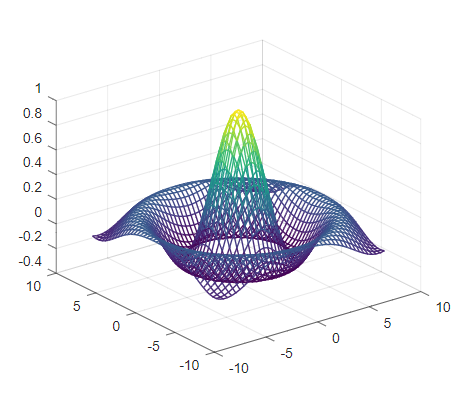
Engineered to doom – Red flags for PCB design
- Next Octave:3> File Operations
- Previous Octave:2> Scripts and Algorithm
- Git- The ultimate version control (1)
- Image Profiler (1)
- DIY Electronics (1)
- GNU Octave (11)
- PCB Design (10)

- February 2020
- October 2019
- February 2018
10.1 The if Statement
The if statement is Octave’s decision-making statement. There are three basic forms of an if statement. In its simplest form, it looks like this:
condition is an expression that controls what the rest of the statement will do. The then-body is executed only if condition is true.
The condition in an if statement is considered true if its value is nonzero, and false if its value is zero. If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if it is non-empty and all of the elements are nonzero. The conceptually equivalent code when condition is a matrix is shown below.
The second form of an if statement looks like this:
If condition is true, then-body is executed; otherwise, else-body is executed.
Here is an example:
In this example, if the expression rem (x, 2) == 0 is true (that is, the value of x is divisible by 2), then the first printf statement is evaluated, otherwise the second printf statement is evaluated.
The third and most general form of the if statement allows multiple decisions to be combined in a single statement. It looks like this:
Any number of elseif clauses may appear. Each condition is tested in turn, and if one is found to be true, its corresponding body is executed. If none of the conditions are true and the else clause is present, its body is executed. Only one else clause may appear, and it must be the last part of the statement.
In the following example, if the first condition is true (that is, the value of x is divisible by 2), then the first printf statement is executed. If it is false, then the second condition is tested, and if it is true (that is, the value of x is divisible by 3), then the second printf statement is executed. Otherwise, the third printf statement is performed.
Note that the elseif keyword must not be spelled else if , as is allowed in Fortran. If it is, the space between the else and if will tell Octave to treat this as a new if statement within another if statement’s else clause. For example, if you write
Octave will expect additional input to complete the first if statement. If you are using Octave interactively, it will continue to prompt you for additional input. If Octave is reading this input from a file, it may complain about missing or mismatched end statements, or, if you have not used the more specific end statements ( endif , endfor , etc.), it may simply produce incorrect results, without producing any warning messages.
It is much easier to see the error if we rewrite the statements above like this,
using the indentation to show how Octave groups the statements. See Functions and Scripts .
© 1996–2018 John W. Eaton Permission is granted to make and distribute verbatim copies of this manual provided the copyright notice and this permission notice are preserved on all copies. Permission is granted to copy and distribute modified versions of this manual under the conditions for verbatim copying, provided that the entire resulting derived work is distributed under the terms of a permission notice identical to this one.Permission is granted to copy and distribute translations of this manual into another language, under the above conditions for modified versions. https://octave.org/doc/interpreter/The-if-Statement.html

Assignment 8: Conditionals
Assignment 8, if statements, color slider, picture chooser.
Next: Increment Ops , Previous: Boolean Expressions , Up: Expressions [ Contents ][ Index ]
8.6 Assignment Expressions
An assignment is an expression that stores a new value into a variable. For example, the following expression assigns the value 1 to the variable z :
After this expression is executed, the variable z has the value 1. Whatever old value z had before the assignment is forgotten. The ‘ = ’ sign is called an assignment operator .
Assignments can store string values also. For example, the following expression would store the value "this food is good" in the variable message :
(This also illustrates concatenation of strings.)
Most operators (addition, concatenation, and so on) have no effect except to compute a value. If you ignore the value, you might as well not use the operator. An assignment operator is different. It does produce a value, but even if you ignore the value, the assignment still makes itself felt through the alteration of the variable. We call this a side effect .
The left-hand operand of an assignment need not be a variable (see Variables ). It can also be an element of a matrix (see Index Expressions ) or a list of return values (see Calling Functions ). These are all called lvalues , which means they can appear on the left-hand side of an assignment operator. The right-hand operand may be any expression. It produces the new value which the assignment stores in the specified variable, matrix element, or list of return values.
It is important to note that variables do not have permanent types. The type of a variable is simply the type of whatever value it happens to hold at the moment. In the following program fragment, the variable foo has a numeric value at first, and a string value later on:
When the second assignment gives foo a string value, the fact that it previously had a numeric value is forgotten.
Assignment of a scalar to an indexed matrix sets all of the elements that are referenced by the indices to the scalar value. For example, if a is a matrix with at least two columns,
sets all the elements in the second column of a to 5.
Assigning an empty matrix ‘ [] ’ works in most cases to allow you to delete rows or columns of matrices and vectors. See Empty Matrices . For example, given a 4 by 5 matrix A , the assignment
deletes the third row of A , and the assignment
deletes the first, third, and fifth columns.
An assignment is an expression, so it has a value. Thus, z = 1 as an expression has the value 1. One consequence of this is that you can write multiple assignments together:
stores the value 0 in all three variables. It does this because the value of z = 0 , which is 0, is stored into y , and then the value of y = z = 0 , which is 0, is stored into x .
This is also true of assignments to lists of values, so the following is a valid expression
that is exactly equivalent to
In expressions like this, the number of values in each part of the expression need not match. For example, the expression
is equivalent to
The number of values on the left side of the expression can, however, not exceed the number of values on the right side. For example, the following will produce an error.
The symbol ~ may be used as a placeholder in the list of lvalues, indicating that the corresponding return value should be ignored and not stored anywhere:
This is cleaner and more memory efficient than using a dummy variable. The nargout value for the right-hand side expression is not affected. If the assignment is used as an expression, the return value is a comma-separated list with the ignored values dropped.
A very common programming pattern is to increment an existing variable with a given value, like this
This can be written in a clearer and more condensed form using the += operator
Similar operators also exist for subtraction ( -= ), multiplication ( *= ), and division ( /= ). An expression of the form
is evaluated as
where op can be either + , - , * , or / , as long as expr2 is a simple expression with no side effects. If expr2 also contains an assignment operator, then this expression is evaluated as
where temp is a placeholder temporary value storing the computed result of evaluating expr2 . So, the expression
You can use an assignment anywhere an expression is called for. For example, it is valid to write x != (y = 1) to set y to 1 and then test whether x equals 1. But this style tends to make programs hard to read. Except in a one-shot program, you should rewrite it to get rid of such nesting of assignments. This is never very hard.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Octave: How to correctly make string as a condition?
I was trying the if test for the first time, well actually so does with function.
Here's the script:
There are 2 things that I don't understand:
- Why do I have to write the condition with quotation marks? (i.e "yes" not yes or "no" not no ).
- Why the 'no' condition ran the input command when it should've been randi ?
Can someone show me how it should be done?
- if-statement

I = "yes" assigns the string "yes" to the variable I . To make a comparison, use == like this: I == "yes" . But this comparison will only work if the two strings are the same length, and will return an array, not a single equality value. Use strcmp to compare strings: if strcmp(I, "yes") .
The input function parses what the user types, so that typing 3 will result in the number 3 , not the string "3" . If you add a second input argument like this: input("prompt","s") then it will not parse the input, and return a string. The user will then be able to type no . See the docs .
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged function if-statement octave octave-gui or ask your own question .
- The Overflow Blog
- Where developers feel AI coding tools are working—and where they’re missing...
- He sold his first company for billions. Now he’s building a better developer...
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Announcing the new Staging Ground Reviewer Stats Widget
- Should low-scoring meta questions no longer be hidden on the Meta.SO home...
Hot Network Questions
- Does Newton's third law violate the law of energy conservation?
- A time-travel short story where the using a time-travel device, its inventor provided an alibi for his future murderer (his wife)
- Figure out which of your friends reveals confidential information to the media!
- Why doesn't SpaceX use a normal blast trench like Saturn V?
- Ideas for high school research project related to probability
- Folding a 40º coffee filter cone
- Is it even possible to build a beacon to announce we exist?
- Why aren't some "conditions" officially a condition? (Burning, Bloodied)
- What is the equivalent of mechanical AND gate?
- Do "always prepared" Spells cost spell slots?
- Is there any reason _not_ to add a flyback diode?
- Can I stack deck floor frames on top of each other?
- What is a Locus?
- Why would an escrow/title company not accept ACH payments?
- Does "DNS provider" refer to an authoritative DNS server or a DNS resolver?
- Is there a way to have my iPhone register my car which doesn't have carplay, only for the "Car is parked at"-feature?
- Can Inductors be thought of as storing voltage?
- Is p→p a theorem in intuitionistic logic?
- Can we divide the story points across team members?
- Firefox isn't upgraded on Debian: its ESR has 1.5 years old, ensuring it being discarded. How to ask a global upgrade from Debian or Mozilla team?
- Are there individual protons and neutrons in a nucleus?
- Why should an attacker perform a clickjacking attack when they can simulate the click with JavaScript?
- Starter circuit for small 12 V solar panel/submersible water pump
- Does AI use lots of water?

IMAGES
VIDEO
COMMENTS
However, using conditionals with matrices only seems to let you assign a scalar to some elements, not a full matrix. I tried using conditionals to determine which elements are iterated, but using conditionals changes the dimensions of the matrix to a one dimensional array. Zn = Zn.^2+Z; Iter(Iter==0 & (abs(Zn)>10))=m; Here's my full program.
condition is an expression that controls what the rest of the statement will do. The then-body is executed only if condition is true.. The condition in an if statement is considered true if its value is nonzero, and false if its value is zero. If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if it is non-empty and all of the ...
If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if all of the elements are non-zero. Octave's while ... The assignment expression in the for statement works a bit differently than Octave's normal assignment statement. Instead of assigning the complete result of the ...
The tool that Octave gives us for doing this is called a conditional statement, and it looks like this: ... Conditional statements don't have to have an else block. If there isn't one, Octave simply doesn't do anything if the test is false: ... This is because the latter is used to mean assignment.
Matlab/Octave tutorial for conditional statements for absolute beginners.Please feel free to make any comments, and subscribe and thumbs up if you like the v...
Note that in conditional contexts (like the test clause of if and while statements) Octave treats the test as if you had typed all (all (condition)). Function File: z = xor (x, y) Function File: z = xor (x1, x2, …) Return the exclusive or of x and y. For boolean expressions x and y, xor (x, y) is true if and only if one of x or y is true.
In this tutorial if elseif and else conditional statements are explained in GNU Octave run in a Windows 11 Pro environment.
Expressions are the basic building block of statements in Octave. An expression evaluates to a value, which you can print, test, store in a variable, pass to a function, or assign a new value to a variable with an assignment operator. An expression can serve as a statement on its own.
This page titled 7.5: Working with Conditions is shared under a CC BY-NC-SA 4.0 license and was authored, remixed, and/or curated by Allen B. Downey (Green Tea Press) via source content that was edited to the style and standards of the LibreTexts platform. Shows how to use conditions to test for valid variable values.
Expressions are the basic building block of statements in Octave. It can be created using values, variables that have already been created, operators, functions, and parentheses. An expression evaluates to value and can serve as a statement on its own. Generally, statements contain one or more expressions that specify data to be operated on.
the value of the variable b is incremented even if the variable a is zero.. This behavior is necessary for the boolean operators to work as described for matrix-valued operands. Built-in Function: z = and (x, y) Built-in Function: z = and (x1, x2, …) Return the logical AND of x and y.. This function is equivalent to the operator syntax x & y.If more than two arguments are given, the logical ...
This entry is part 8 of 11 in the series GNU Octave. To control the flow operations in an algorithm, one must be able to verify some conditions and act accordingly. Other-times it might be required to perform same task repeatedly until certain conditions are met. Conditional execution are the backbone of any algorithm.
The if statement is Octave's decision-making statement. There are three basic forms of an if statement. ... If the value of the conditional expression in an if statement is a vector or a matrix, it is considered true only if it is non-empty and all of the elements are nonzero. The conceptually equivalent code when condition is a matrix is ...
The functions any and all are useful for determining whether any or all of the elements of a matrix satisfy some condition. The find function is also useful in determining which elements of a matrix meet a specified condition. : tf = any (x) ¶. : tf = any (x, dim) ¶. For a vector argument, return true (logical 1) if any element of the vector ...
10 Statements ¶. Statements may be a simple constant expression or a complicated list of nested loops and conditional statements. Control statements such as if, while, and so on control the flow of execution in Octave programs.All the control statements start with special keywords such as if and while, to distinguish them from simple expressions.Many control statements contain other ...
Small Medium Large. @kagecl
An assignment is an expression that stores a new value into a variable. For example, the following expression assigns the value 1 to the variable z: z = 1. After this expression is executed, the variable z has the value 1. Whatever old value z had before the assignment is forgotten. The ' = ' sign is called an assignment operator.
4. Your "Code with ternary operation" is valid in Octave and MATLAB. It's not a true ternary operator, but it produces equivalent results. The advantage of that code of the if / else code is that it will work with arrays, not only with scalar values. - Cris Luengo.
1. I = "yes" assigns the string "yes" to the variable I. To make a comparison, use == like this: I == "yes". But this comparison will only work if the two strings are the same length, and will return an array, not a single equality value. Use strcmp to compare strings: if strcmp(I, "yes"). The input function parses what the user types, so that ...