Software Architecture Patterns by
Get full access to Software Architecture Patterns and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.

Chapter 1. Layered Architecture
The most common architecture pattern is the layered architecture pattern, otherwise known as the n-tier architecture pattern. This pattern is the de facto standard for most Java EE applications and therefore is widely known by most architects, designers, and developers. The layered architecture pattern closely matches the traditional IT communication and organizational structures found in most companies, making it a natural choice for most business application development efforts.
Pattern Description
Components within the layered architecture pattern are organized into horizontal layers, each layer performing a specific role within the application (e.g., presentation logic or business logic). Although the layered architecture pattern does not specify the number and types of layers that must exist in the pattern, most layered architectures consist of four standard layers: presentation, business, persistence, and database ( Figure 1-1 ). In some cases, the business layer and persistence layer are combined into a single business layer, particularly when the persistence logic (e.g., SQL or HSQL) is embedded within the business layer components. Thus, smaller applications may have only three layers, whereas larger and more complex business applications may contain five or more layers.
Each layer of the layered architecture pattern has a specific role and responsibility within the application. For example, a presentation layer would be responsible for handling all user interface and browser communication logic, whereas a business layer would be responsible for executing specific business rules associated with the request. Each layer in the architecture forms an abstraction around the work that needs to be done to satisfy a particular business request. For example, the presentation layer doesn’t need to know or worry about how to get customer data; it only needs to display that information on a screen in particular format. Similarly, the business layer doesn’t need to be concerned about how to format customer data for display on a screen or even where the customer data is coming from; it only needs to get the data from the persistence layer, perform business logic against the data (e.g., calculate values or aggregate data), and pass that information up to the presentation layer.
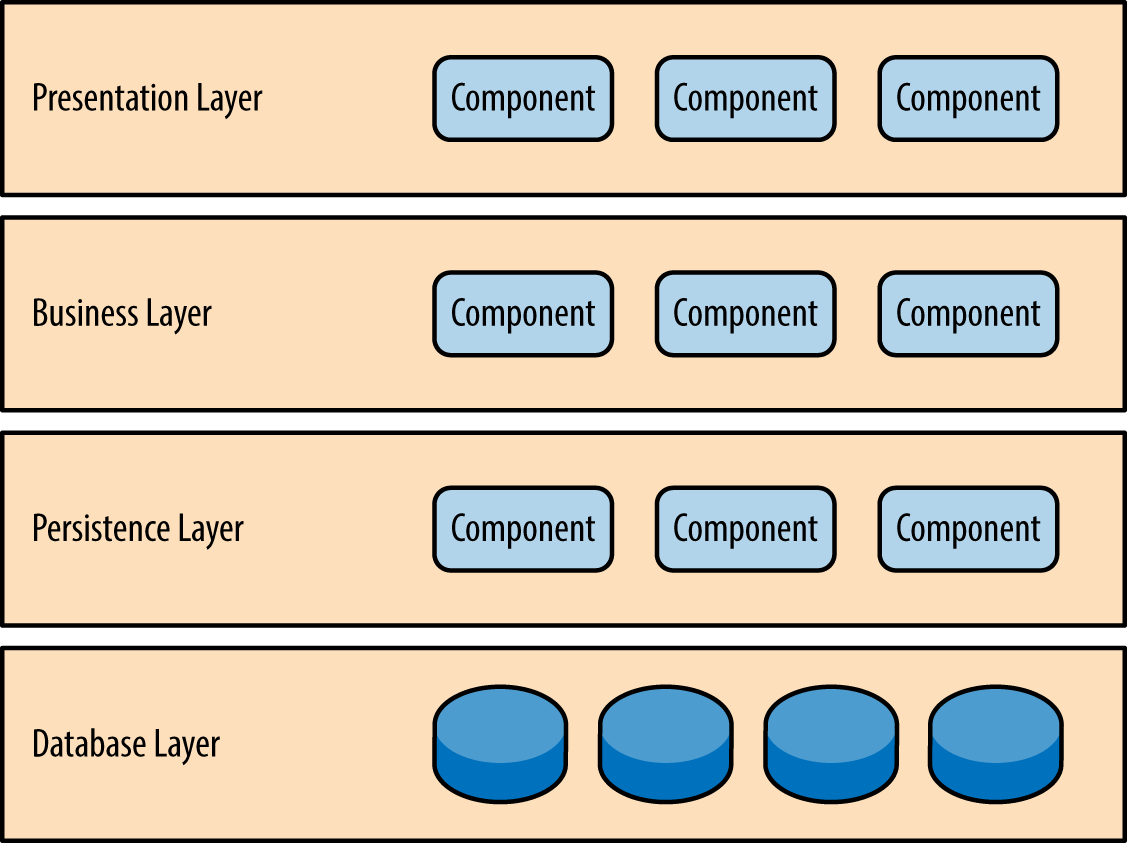
Figure 1-1. Layered architecture pattern
One of the powerful features of the layered architecture pattern is the separation of concerns among components. Components within a specific layer deal only with logic that pertains to that layer. For example, components in the presentation layer deal only with presentation logic, whereas components residing in the business layer deal only with business logic. This type of component classification makes it easy to build effective roles and responsibility models into your architecture, and also makes it easy to develop, test, govern, and maintain applications using this architecture pattern due to well-defined component interfaces and limited component scope.
Key Concepts
Notice in Figure 1-2 that each of the layers in the architecture is marked as being closed . This is a very important concept in the layered architecture pattern. A closed layer means that as a request moves from layer to layer, it must go through the layer right below it to get to the next layer below that one. For example, a request originating from the presentation layer must first go through the business layer and then to the persistence layer before finally hitting the database layer.

Figure 1-2. Closed layers and request access
So why not allow the presentation layer direct access to either the persistence layer or database layer? After all, direct database access from the presentation layer is much faster than going through a bunch of unnecessary layers just to retrieve or save database information. The answer to this question lies in a key concept known as layers of isolation .
The layers of isolation concept means that changes made in one layer of the architecture generally don’t impact or affect components in other layers: the change is isolated to the components within that layer, and possibly another associated layer (such as a persistence layer containing SQL). If you allow the presentation layer direct access to the persistence layer, then changes made to SQL within the persistence layer would impact both the business layer and the presentation layer, thereby producing a very tightly coupled application with lots of interdependencies between components. This type of architecture then becomes very hard and expensive to change.
The layers of isolation concept also means that each layer is independent of the other layers, thereby having little or no knowledge of the inner workings of other layers in the architecture. To understand the power and importance of this concept, consider a large refactoring effort to convert the presentation framework from JSP (Java Server Pages) to JSF (Java Server Faces). Assuming that the contracts (e.g., model) used between the presentation layer and the business layer remain the same, the business layer is not affected by the refactoring and remains completely independent of the type of user-interface framework used by the presentation layer.
While closed layers facilitate layers of isolation and therefore help isolate change within the architecture, there are times when it makes sense for certain layers to be open. For example, suppose you want to add a shared-services layer to an architecture containing common service components accessed by components within the business layer (e.g., data and string utility classes or auditing and logging classes). Creating a services layer is usually a good idea in this case because architecturally it restricts access to the shared services to the business layer (and not the presentation layer). Without a separate layer, there is nothing architecturally that restricts the presentation layer from accessing these common services, making it difficult to govern this access restriction.
In this example, the new services layer would likely reside below the business layer to indicate that components in this services layer are not accessible from the presentation layer. However, this presents a problem in that the business layer is now required to go through the services layer to get to the persistence layer, which makes no sense at all. This is an age-old problem with the layered architecture, and is solved by creating open layers within the architecture.
As illustrated in Figure 1-3 , the services layer in this case is marked as open, meaning requests are allowed to bypass this open layer and go directly to the layer below it. In the following example, since the services layer is open, the business layer is now allowed to bypass it and go directly to the persistence layer, which makes perfect sense.
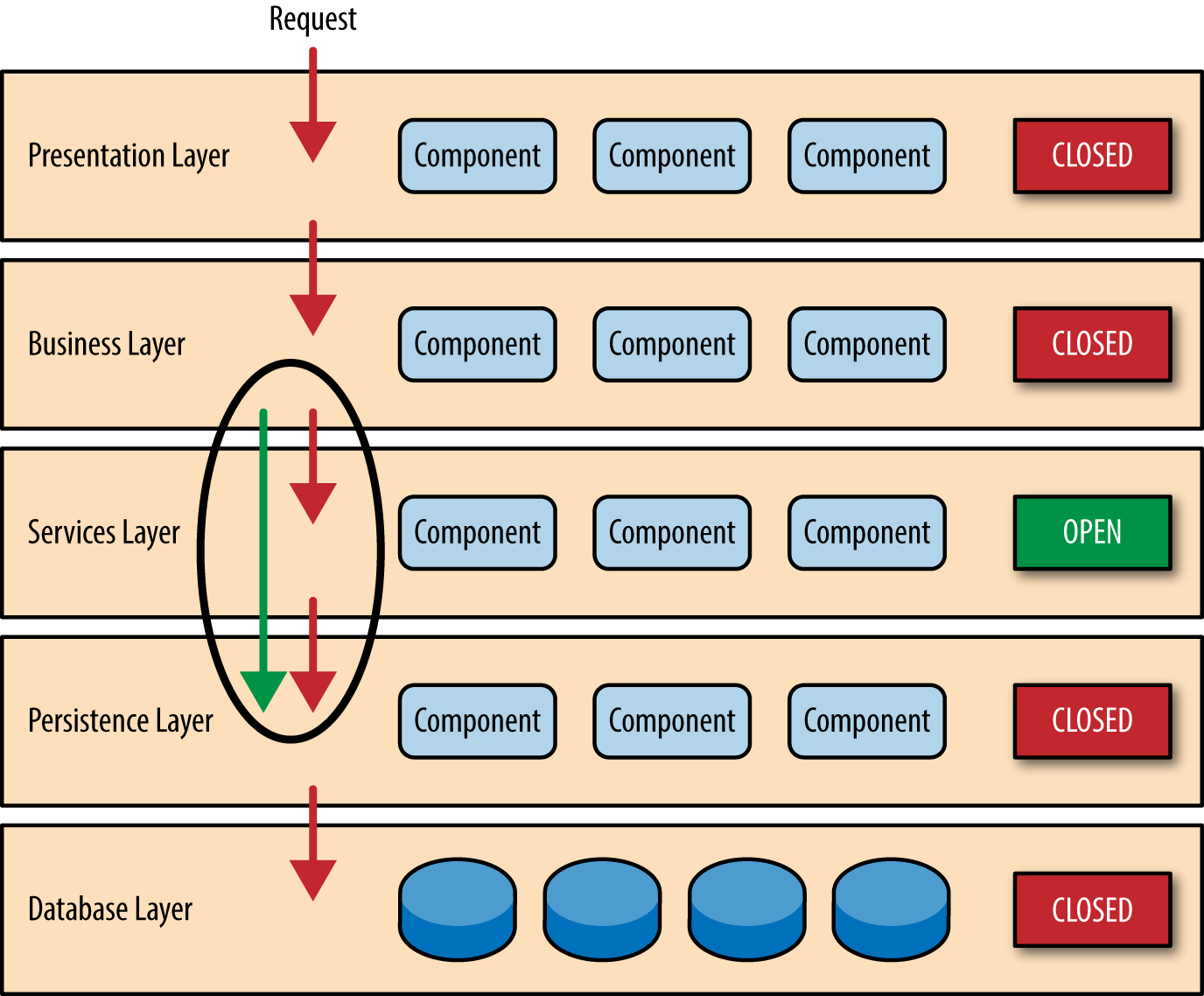
Figure 1-3. Open layers and request flow
Leveraging the concept of open and closed layers helps define the relationship between architecture layers and request flows and also provides designers and developers with the necessary information to understand the various layer access restrictions within the architecture. Failure to document or properly communicate which layers in the architecture are open and closed (and why) usually results in tightly coupled and brittle architectures that are very difficult to test, maintain, and deploy.
Pattern Example
To illustrate how the layered architecture works, consider a request from a business user to retrieve customer information for a particular individual as illustrated in Figure 1-4 . The black arrows show the request flowing down to the database to retrieve the customer data, and the red arrows show the response flowing back up to the screen to display the data. In this example, the customer information consists of both customer data and order data (orders placed by the customer).
The customer screen is responsible for accepting the request and displaying the customer information. It does not know where the data is, how it is retrieved, or how many database tables must be queries to get the data. Once the customer screen receives a request to get customer information for a particular individual, it then forwards that request onto the customer delegate module. This module is responsible for knowing which modules in the business layer can process that request and also how to get to that module and what data it needs (the contract). The customer object in the business layer is responsible for aggregating all of the information needed by the business request (in this case to get customer information). This module calls out to the customer dao (data access object) module in the persistence layer to get customer data, and also the order dao module to get order information. These modules in turn execute SQL statements to retrieve the corresponding data and pass it back up to the customer object in the business layer. Once the customer object receives the data, it aggregates the data and passes that information back up to the customer delegate, which then passes that data to the customer screen to be presented to the user.

Figure 1-4. Layered architecture example
From a technology perspective, there are literally dozens of ways these modules can be implemented. For example, in the Java platform, the customer screen can be a (JSF) Java Server Faces screen coupled with the customer delegate as the managed bean component. The customer object in the business layer can be a local Spring bean or a remote EJB3 bean. The data access objects illustrated in the previous example can be implemented as simple POJO’s (Plain Old Java Objects), MyBatis XML Mapper files, or even objects encapsulating raw JDBC calls or Hibernate queries. From a Microsoft platform perspective, the customer screen can be an ASP (active server pages) module using the .NET framework to access C# modules in the business layer, with the customer and order data access modules implemented as ADO (ActiveX Data Objects).
Considerations
The layered architecture pattern is a solid general-purpose pattern, making it a good starting point for most applications, particularly when you are not sure what architecture pattern is best suited for your application. However, there are a couple of things to consider from an architecture standpoint when choosing this pattern.
The first thing to watch out for is what is known as the architecture sinkhole anti-pattern . This anti-pattern describes the situation where requests flow through multiple layers of the architecture as simple pass-through processing with little or no logic performed within each layer. For example, assume the presentation layer responds to a request from the user to retrieve customer data. The presentation layer passes the request to the business layer, which simply passes the request to the persistence layer, which then makes a simple SQL call to the database layer to retrieve the customer data. The data is then passed all the way back up the stack with no additional processing or logic to aggregate, calculate, or transform the data.
Every layered architecture will have at least some scenarios that fall into the architecture sinkhole anti-pattern. The key, however, is to analyze the percentage of requests that fall into this category. The 80-20 rule is usually a good practice to follow to determine whether or not you are experiencing the architecture sinkhole anti-pattern. It is typical to have around 20 percent of the requests as simple pass-through processing and 80 percent of the requests having some business logic associated with the request. However, if you find that this ratio is reversed and a majority of your requests are simple pass-through processing, you might want to consider making some of the architecture layers open, keeping in mind that it will be more difficult to control change due to the lack of layer isolation.
Another consideration with the layered architecture pattern is that it tends to lend itself toward monolithic applications, even if you split the presentation layer and business layers into separate deployable units. While this may not be a concern for some applications, it does pose some potential issues in terms of deployment, general robustness and reliability, performance, and scalability.
Pattern Analysis
The following table contains a rating and analysis of the common architecture characteristics for the layered architecture pattern. The rating for each characteristic is based on the natural tendency for that characteristic as a capability based on a typical implementation of the pattern, as well as what the pattern is generally known for. For a side-by-side comparison of how this pattern relates to other patterns in this report, please refer to Appendix A at the end of this report.
Get Software Architecture Patterns now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
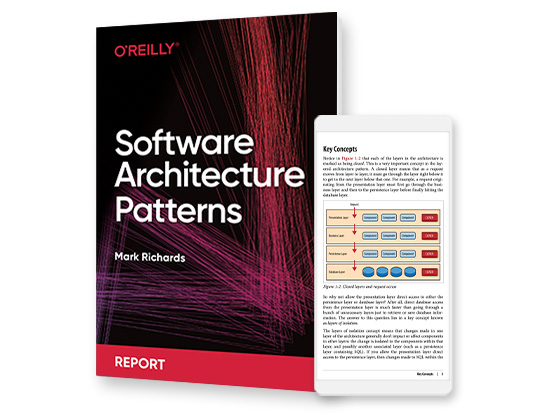
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
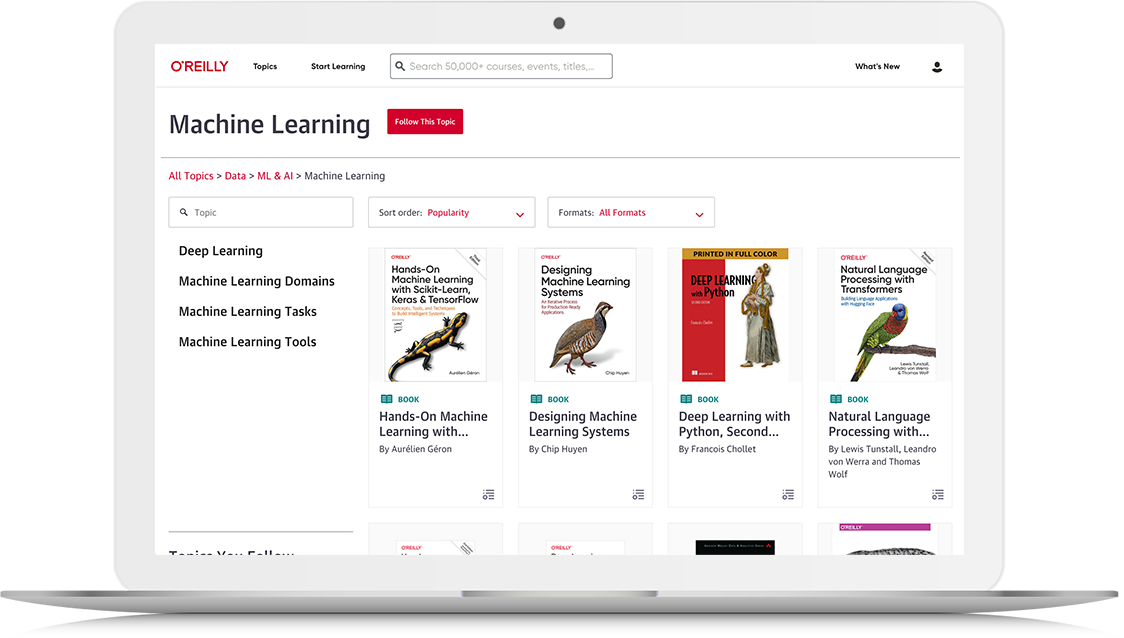
- Discover Architecture
- Prevent Architectural Drift
- Increase Application Resiliency
- Manage and Remediate Technical Debt
- Monolith to Microservices
- Partners Overview
- Cloud Providers
- Global System Integrators
- System Integrators
- Become a Partner
- Awards & Recognition
- Upcoming Events
- Analyst Reports
- Case Studies
- Frequently Asked Questions
- Refactor This
- Request a Demo
Download Gartner® Report on Cool Vendors™ in AI-Augmented Development and Testing for Software Engineering
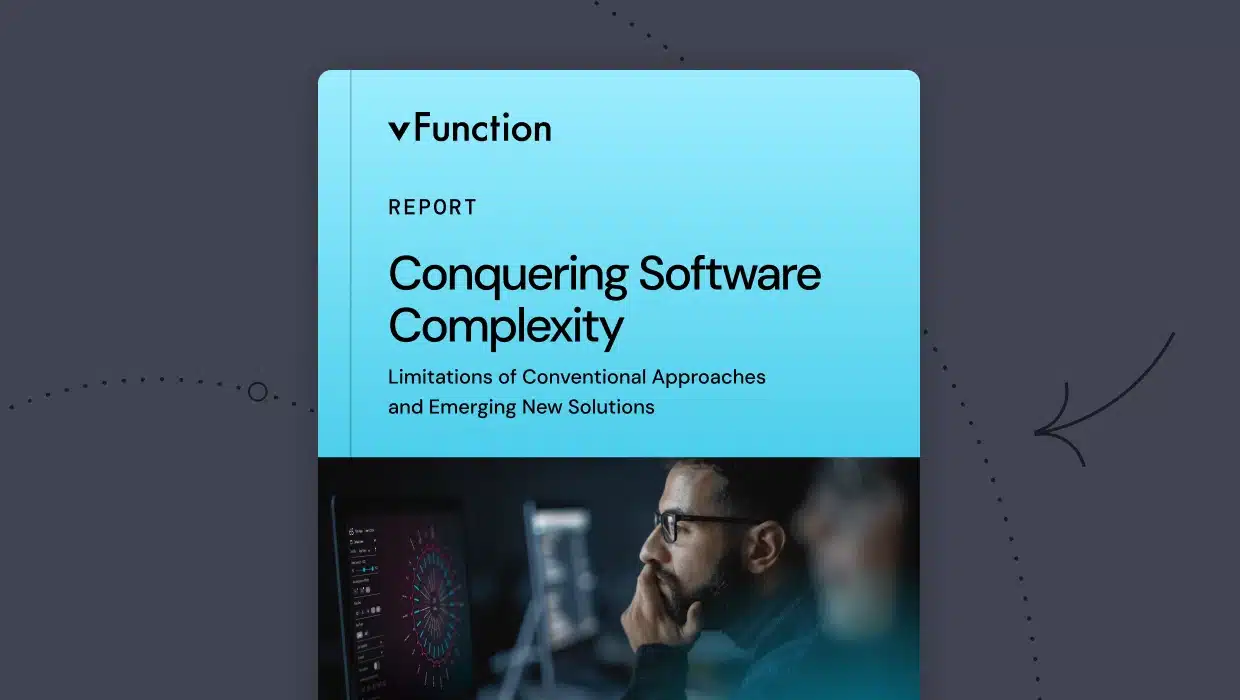
Conquering Software Complexity: Conventional Approaches and Emerging Solutions
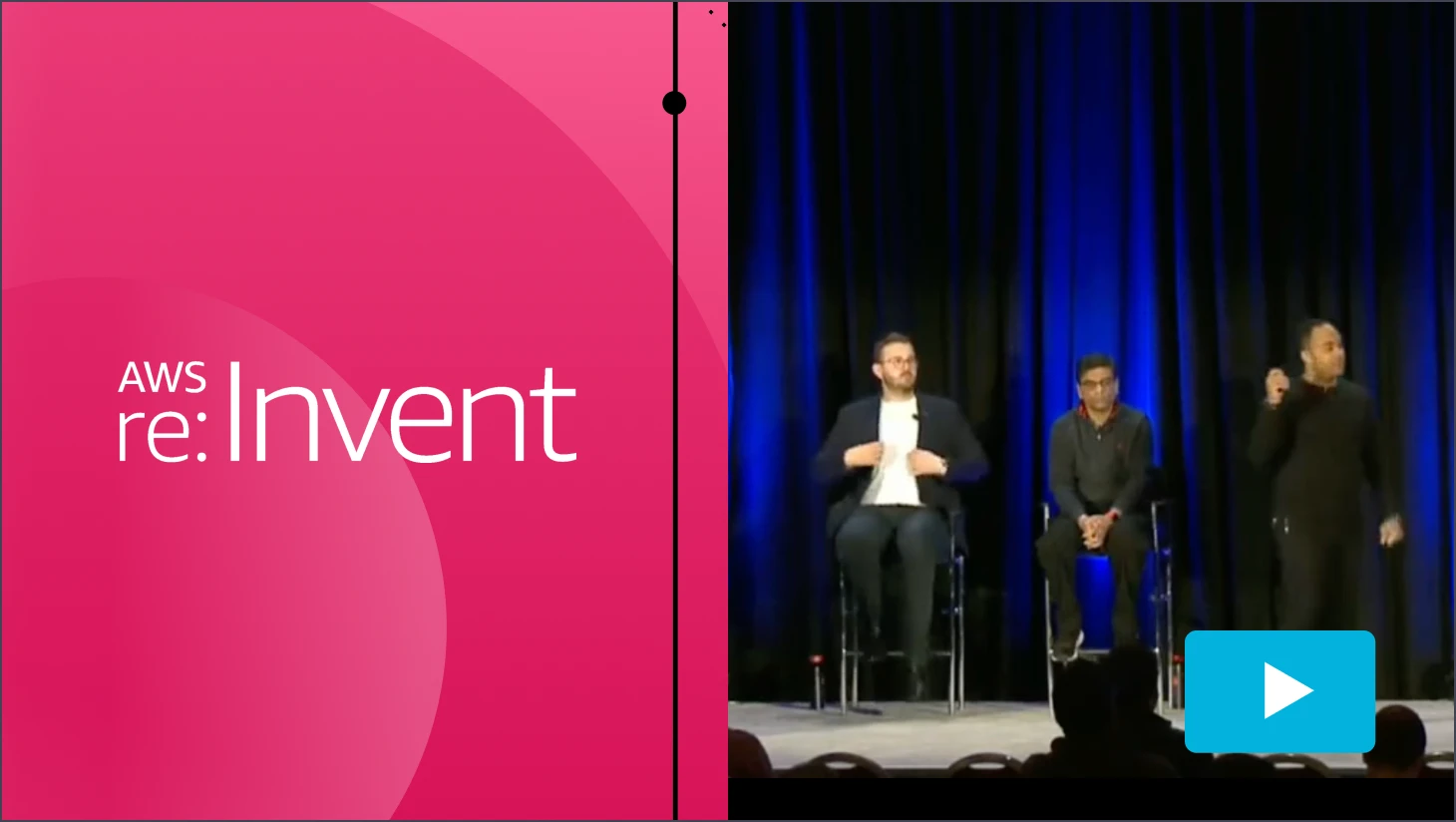
AWS re:Invent 2023: Evolution from migration to modernization
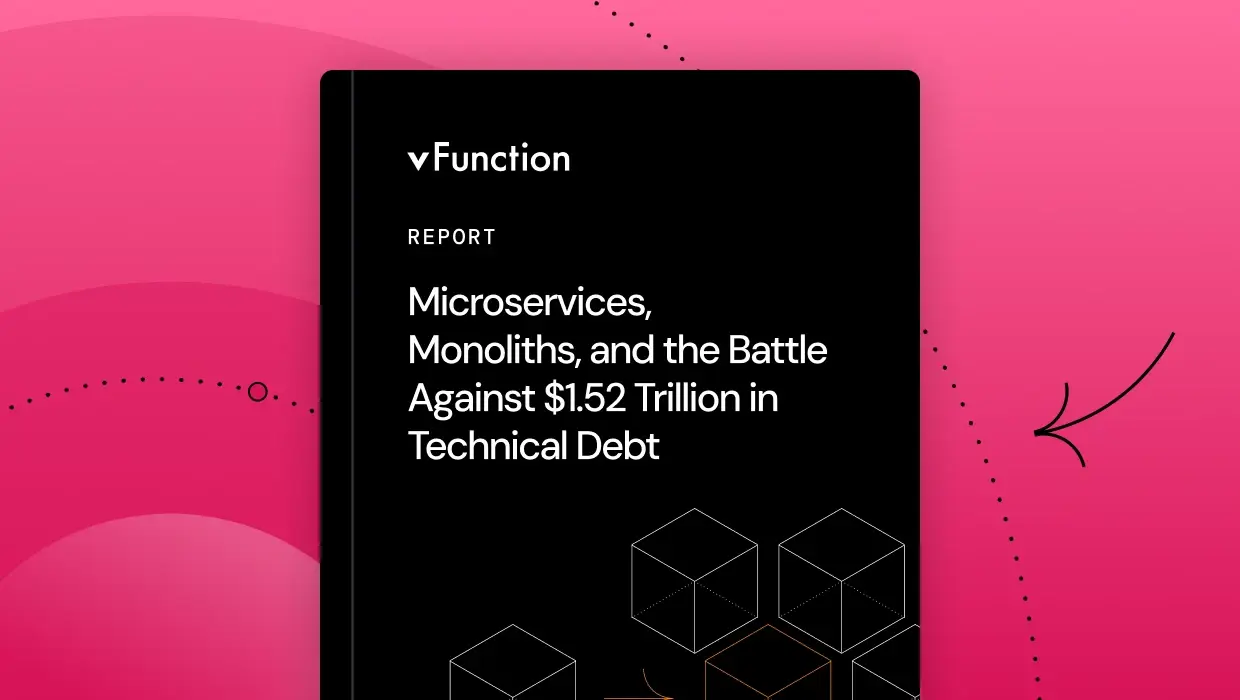
Microservices, Monoliths, and the Battle Against $1.52 Trillion in Technical Debt
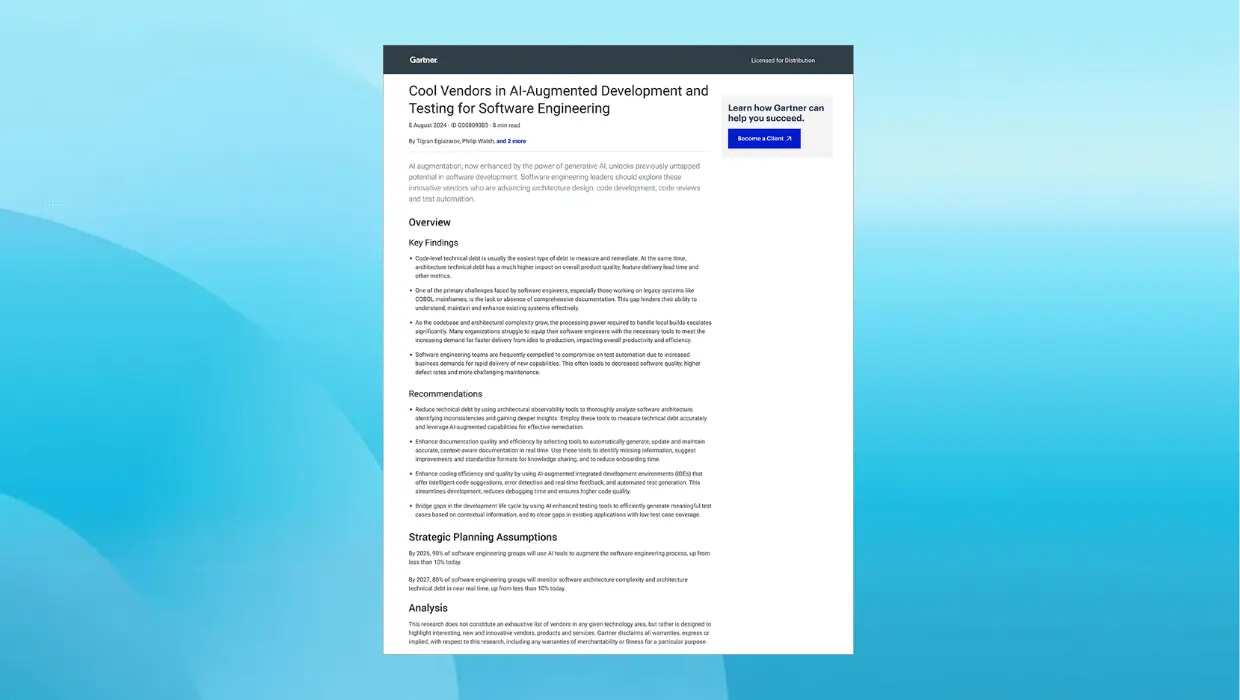
Cool Vendors™ in AI-Augmented Development and Testing for Software Engineering
What is a 3-tier application architecture? Definition and Examples
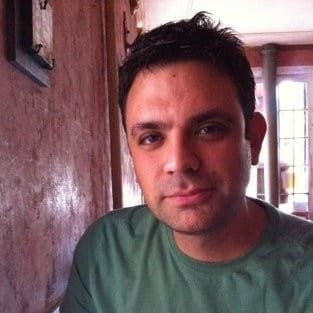
Michael Chiaramonte
May 13, 2024
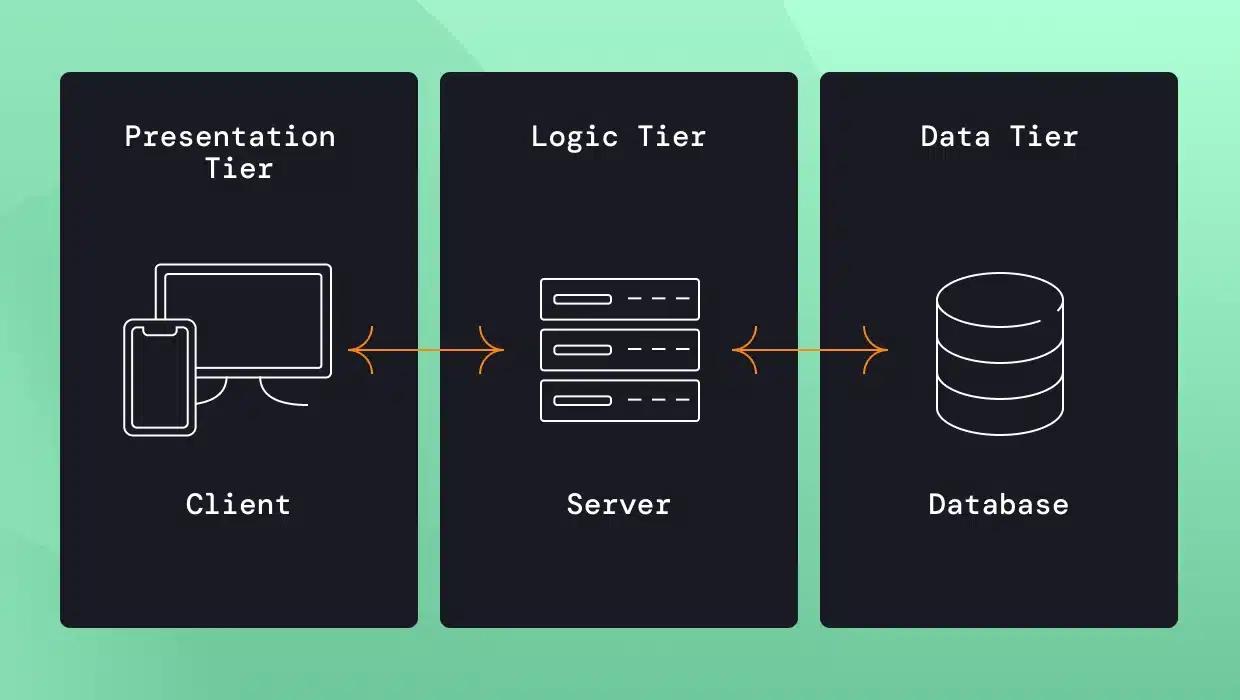
In software development, it’s very common to see applications built with a specific architectural paradigm in mind. One of the most prevalent patterns seen in modern software architecture is the 3-tier (or three-tier) architecture. This model structures an application into three distinct tiers: presentation (user interface), logic(business logic), and data (data storage).
The fundamental advantage of 3-tier architecture lies in the clear separation of concerns. Each tier operates independently, allowing developers to focus on specific aspects of the application without affecting other layers. This enhances maintainability, as updates or changes can be made to a single tier with minimal impact on the others. 3-tier applications are also highly scalable since each tier can be scaled horizontally or vertically to handle increased demand as usage grows.
This post delves into the fundamentals of 3-tier applications. In it, We’ll cover:
- The concept of 3-tier architecture: What it is and why it’s important.
- The role of each tier: Detailed explanations of the presentation, application, and data tiers.
- How the three tiers interact: The flow of data and communication within a 3-tier application.
- Real-world examples: Practical illustrations of how 3-tier architecture is used.
- Benefits of this approach: Advantages for developers, architects, and end-users.
With the agenda set, let’s precisely define the three tiers of the architecture in greater detail.
What is a 3-tier application architecture?
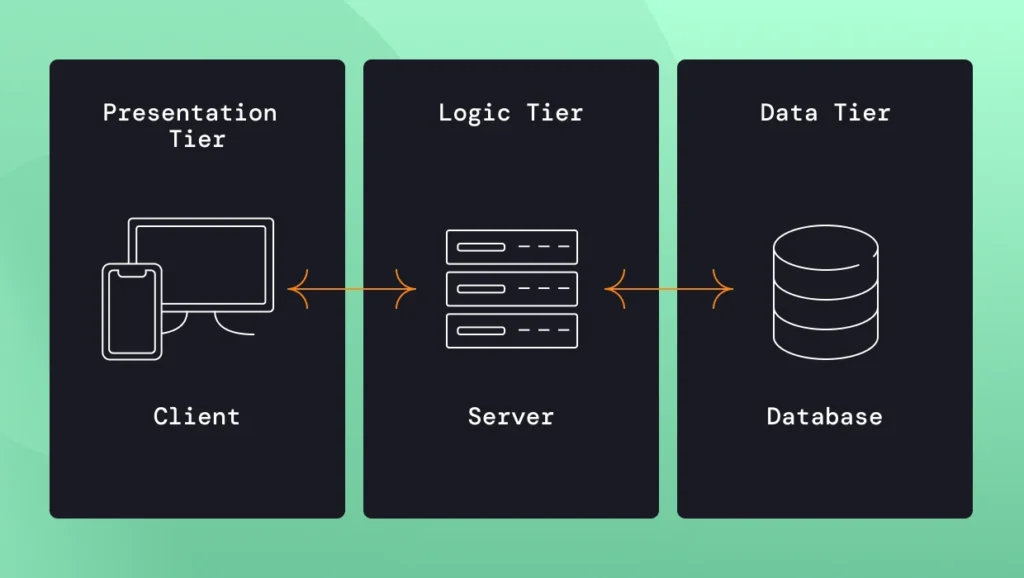
A 3-tier application is a model that divides an application into three interconnected layers:
- Presentation Tier: The user interface where the end-user interacts with the system (e.g., a web browser or a mobile app).
- Logic Tier: The middle tier of the architecture, also known as the logic tier, handles the application’s core processing, business rules, and calculations.
- Data Tier: Manages the storage, retrieval, and manipulation of the application’s data, typically utilizing a database.
This layered separation offers several key advantages that we will explore in more depth later in the post, but first, let’s examine them at a high level.
First, it allows for scalability since each tier can be scaled independently to meet changing performance demands. Second, 3-tier applications are highly flexible; tiers can be updated or replaced with newer technologies without disrupting the entire application. Third, maintainability is enhanced, as modifications to one tier often have minimal or no effect on other tiers. Finally, a layered architecture allows for improved security, as multiple layers of protection can be implemented to safeguard sensitive data and business logic.
How does a 3-tier application architecture work?
The fundamental principle of a 3-tier application is the flow of information and requests through the tiers. Depending on the technologies you use, each layer has mechanisms that allow each part of the architecture to communicate with the other adjacent layer. Here’s a simplified breakdown:
- User Interaction: The user interacts with the presentation tier (e.g., enters data into a web form or clicks a button on a mobile app).
- Request Processing: The presentation tier sends the user’s request to the application tier.
- Business Logic: The logic tier executes the relevant business logic, processes the data, and potentially interacts with the data tier to retrieve or store information.
- Data Access: If necessary, the application tier communicates with the data tier to access the database, either reading data to be processed or writing data for storage.
- Response: The logic tier formulates a response based on the processed data and business rules and packages it into the expected format the presentation tier requires.
- Display: The presentation tier receives the response from the application tier and displays the information to the user (e.g., updates a webpage or renders a result in a mobile app).
The important part is that the user never directly interacts with the logic or data tiers. All user interactions with the application occur through the presentation tier. The same goes for each adjacent layer in the 3-tier application. For example, the presentation layer communicates with the logic layer but never directly with the data layer. To understand how this compares to other n-tier architectural styles, let’s take a look at a brief comparison.
1-tier vs 2-tier vs 3-tier applications
While 3-tier architecture is a popular and well-structured approach, it’s not the only way to build applications. As time has passed, architecture has evolved to contain more layers. Some approaches are still used, especially in legacy applications. Here’s a brief comparison of 1-tier, 2-tier, and 3-tier architectures:
- All application components (presentation, logic, and data) reside within a single program or unit.
- Simpler to develop initially, particularly for small-scale applications.
- It becomes increasingly difficult to maintain and scale as complexity grows.
- Divides the application into two parts: the client (presentation/graphical user interface) and a server, which typically handles both logic and data.
- Offers some modularity and improved scalability compared to 1-tier.
- Can still face scalability challenges for complex systems, as the server tier combines business logic and data access, potentially creating a bottleneck.
- Separates the application into presentation, application (business logic), and data tiers.
- Provides the greatest level of separation, promoting scalability, maintainability, and flexibility.
- Typically requires more development overhead compared to simpler architectures.
The choice of architecture and physical computing tiers that your architecture uses depends on your application’s size, complexity, and scalability requirements. Using a multi-tier architecture tends to be the most popular approach, whether client-server architecture or 3-tier. That being said, monolithic applications still exist and have their place.
The logical tiers of a 3-tier application architecture
The three tiers at the heart of a 3-tier architecture are not simply physical divisions; they also represent a separation in technologies used. Let’s look at each tier in closer detail:
1. Presentation tier
- Focus: User interaction and display of information.
- Role: This is the interface that users see and interact with. It gathers input, formats and sanitizes data, and displays the results returned from the other tiers.
- Web Development: HTML, CSS/SCSS/Sass, TypeScript/JavaScript, front-end frameworks (React, Angular, Vue.js), a web server.
- Mobile Development: Platform-specific technologies (Swift, Kotlin, etc.).
- Desktop Applications: Platform-specific UI libraries or third-party cross-platform development tools.
2. Logic tier
- Focus: Core functionality and business logic.
- Role: This tier is the brain of the application. It processes data, implements business rules and logic, further validates input, and coordinates interactions between the presentation and data tiers.
- Programming Languages: Java, Python, JavaScript, C#, Ruby, etc.
- Web Frameworks: Spring, Django, Ruby on Rails, etc.
- App Server/Web Server
3. Data tier
- Focus: Persistent storage and management of data.
- Role: This tier reliably stores the application’s data and handles all access requests. It protects data integrity and ensures consistency.
- Database servers: Relational (MySQL, PostgreSQL, Microsoft SQL Server) or NoSQL (MongoDB, Cassandra).
- Database Management Systems: Provide tools to create, access, and manage data.
- Storage providers (AWS S3, Azure Blobs, etc)
Separating concerns among these tiers enhances the software’s modularity. This makes updating, maintaining, or replacing specific components easier without breaking the whole application.
3-tier application examples
Whether a desktop or web app, 3-tier applications come in many forms across almost every industry. Here are a few relatable examples of how a 3-tier architecture can be used and a breakdown of what each layer would be responsible for within the system.
E-commerce websites
- Presentation Layer: The online storefront with product catalogs, shopping carts, and checkout interfaces.
- Logic Layer: Handles searching, order processing, inventory management, interfacing with 3rd-party payment vendors, and business rules like discounts and promotions.
- Data Layer: Stores product information, customer data, order history, and financial transactions in a database.
Content management systems (CMS)
- Presentation Layer: The administrative dashboard and the public-facing website.
- LogicLayer: Manages content creation, editing, publishing, and the website’s structure and logic based on rules, permissions, schedules, and configuration
- Data Layer: Stores articles, media files, user information, and website settings.
Customer relationship management (CRM) systems
- Presentation Layer: Web or mobile interfaces for sales and support teams.
- Logic Layer: Processes customer data, tracks interactions, manages sales pipelines, and automates marketing campaigns.
- Data Layer: Maintains a database server with data for customers, contacts, sales opportunities, and support cases.
Online booking platforms (e.g., hotels, flights, appointments)
- Presentation Layer: Search features, promotional materials, and reservation interfaces.
- Logic Layer: Handles availability checks, real-time pricing, booking logic, and payment processing to 3rd-party payment vendors.
- Data Layer: Stores schedules, reservations, inventory information, and customer details.
Of course, these are just a few simplified examples of a 3-tier architecture in action. Many of the applications we use daily will use a 3-tier architecture (or potentially more tiers for a modern web-based application), so finding further examples is generally not much of a stretch. The examples above demonstrate how application functionality can be divided into one of the three tiers.
Benefits of a 3-tier app architecture
One of the benefits of the 3-tier architecture is it’s usually quite apparent why using it would be advantageous over other options, such as a two-tier architecture. However, let’s briefly summarize the advantages and benefits for developers, architects, and end-users who will build or utilize the 3-tier architecture pattern.
Scalability
Each tier can be independently scaled to handle increased load or demand. For example, you can add more servers to the logic tier to improve processing capabilities without affecting the user experience or add more database servers to improve query performance.
Maintainability
Changes to one tier often have minimal impact on the others, making it easier to modify, update, or debug specific application components. As long as contracts between the layers (such as API definitions or data mappings) don’t change, developers can benefit from shorter development cycles and reduced risk.
Flexibility
You can upgrade or replace technologies within individual tiers without overhauling the entire system. This allows for greater adaptability as requirements evolve. For example, if the technology you are using within your data tier does not support a particular feature you need, you can replace that technology while leaving the application and presentation layers untouched, as long as contracts between the layers don’t change (just as above).
Improved Security
Multiple layers of security can be implemented across tiers. This also isolates the sensitive data layer behind the logic layer, reducing potential attack surfaces. For instance, you can have the logic layer enforce field-level validation on a form and sanitize the data that comes through. This allows for two checks on the data, preventing security issues such as SQL injection and others listed in the OWASP Top 10 .
Reusability
Components within the logic tier can sometimes be reused in other applications, promoting efficiency and code standardization. For example, a mobile application, a web application, and a desktop application may all leverage the same application layer and corresponding data layer. If the logic layer is exposed externally through a REST API or similar technology, it also opens up the possibility of leveraging this functionality for third-party developers to take advantage of the API and the underlying functionality.
Developer specialization
Teams can specialize in specific tiers (e.g., front-end, back-end, database), optimizing their skills and improving development efficiency. Although many developers these days focus on full-stack development, larger organizations still divide teams based on frontend and backend technologies. Implementing a 3-tier architecture fits well with this paradigm of splitting up responsibilities.
The benefits listed above cover multiple angles, from staffing and infrastructure to security and beyond. The potential upside of leveraging 3-tier architectures is wide-reaching and broadly applicable. It leaves no question as to why 3-tier architectures have become the standard for almost all modern applications. That being said, many times, the current implementation of an application can be improved, and if an application is currently undergoing modernization, how do you ensure that it will meet your target and future state architecture roadmap? This is where vFunction can swoop in and help.
How vFunction can help with modernizing 3-tier applications
vFunction offers powerful tools to aid architects and developers in streamlining the modernization of 3-tier applications and addressing their potential weaknesses. Here’s how it empowers architects and developers:
Architectural observability
vFunction provides deep insights into your application’s architecture, tracking critical events like new dependencies, domain changes, and increasing complexity over time. This visibility allows you to pinpoint areas for proactive optimization and the creation of modular business domains as you continue to work on the application.
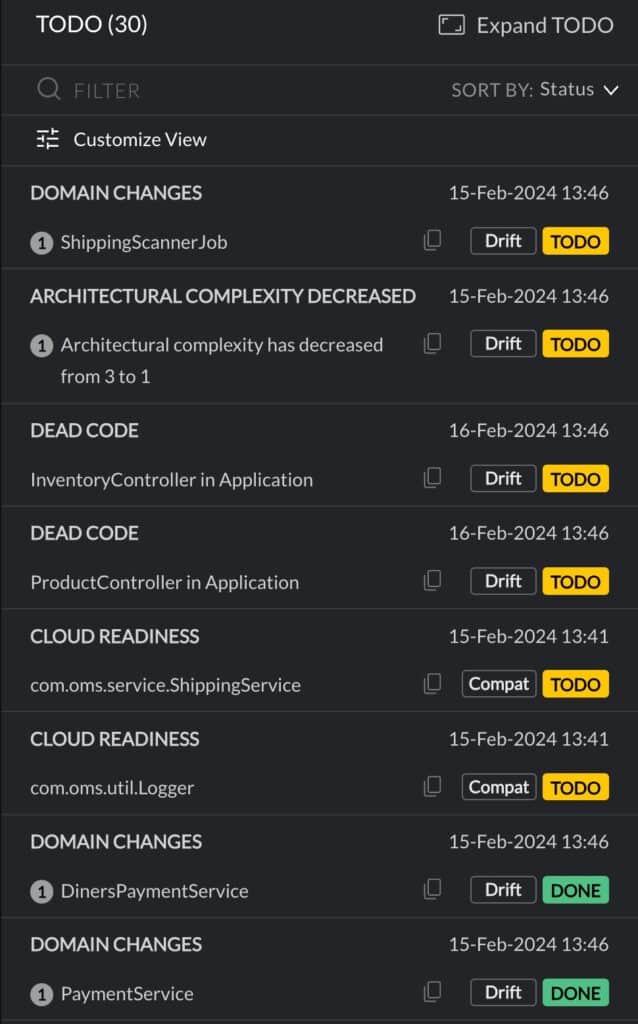
Resiliency enhancement
vFunction helps you identify potential architectural risks that might affect application resiliency . It generates prioritized recommendations and actions to strengthen your architecture and minimize the impact of downtime.
Targeted optimization
vFunction’s analysis pinpoints technical debt and bottlenecks within your applications. This lets you focus modernization efforts where they matter most, promoting engineering velocity, scalability, and performance.
Informed decision-making
vFunction’s comprehensive architectural views support data-driven architecture decisions on refactoring, migrating components to the cloud, or optimizing within the existing structure.
By empowering you with deep architectural insights and actionable recommendations, vFunction accelerates modernization architectural improvement processes, ensuring your 3-tier applications remain adaptable, resilient, and performant as they evolve.
In this post, we looked at how a 3-tier architecture can provide a proven foundation for building scalable, maintainable, and secure applications. By understanding its core principles, the role of each tier, and its real-world applications, developers can leverage this pattern to tackle complex software projects more effectively.
Key takeaways from our deep dive into 3-tier applications include:
- Separation of Concerns: A 3-tier architecture promotes clear modularity, making applications easier to develop, update, and debug.
- Scalability: Its ability to scale tiers independently allows applications to adapt to changing performance demands.
- Flexibility: Technologies within tiers can be updated or replaced without disrupting the entire application.
- Security: The layered design enables enhanced security measures and isolation of sensitive data.
As applications grow in complexity, tools like vFunction become invaluable. vFunction’s focus on architectural observability, analysis, and proactive recommendations means that architects and developers can modernize their applications strategically, with complete visibility of how every change affects the overall system architecture. This allows them to optimize performance, enhance resiliency, and make informed decisions about their architecture’s evolution.
If you’re looking to build modern and resilient software, considering the 3-tier architecture or (a topic for another post) microservices as a starting point, combined with tools like vFunction for managing long-term evolution, can be a recipe for success. Contact us today to learn more about how vFunction can help you modernize and build better software with architectural observability.
Principal Architect
Starting from a young age, Michael has loved building things in code and working with tech. His career has spanned many industries, platforms, and projects over the 20+ years he has worked as a software engineer, architect, and leader.
Other Related Resources
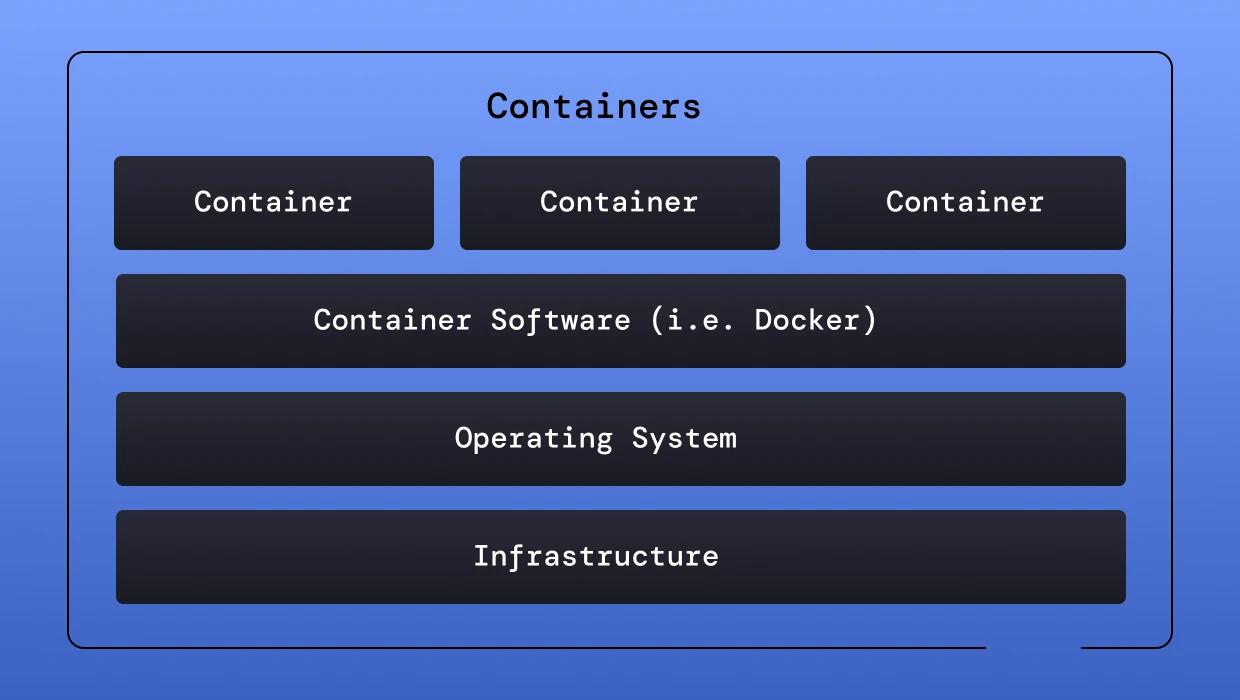
What is Containerization Software? Definition and benefits.
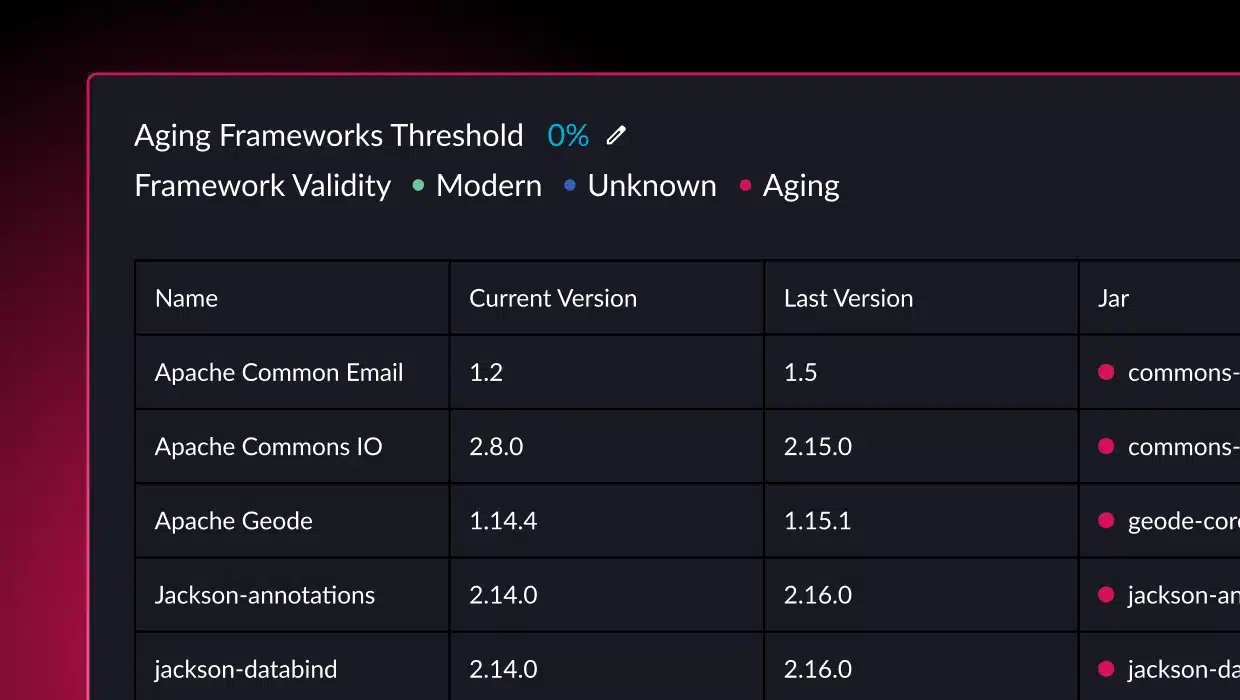
What Is a Monolithic Application? Everything You Need to Know
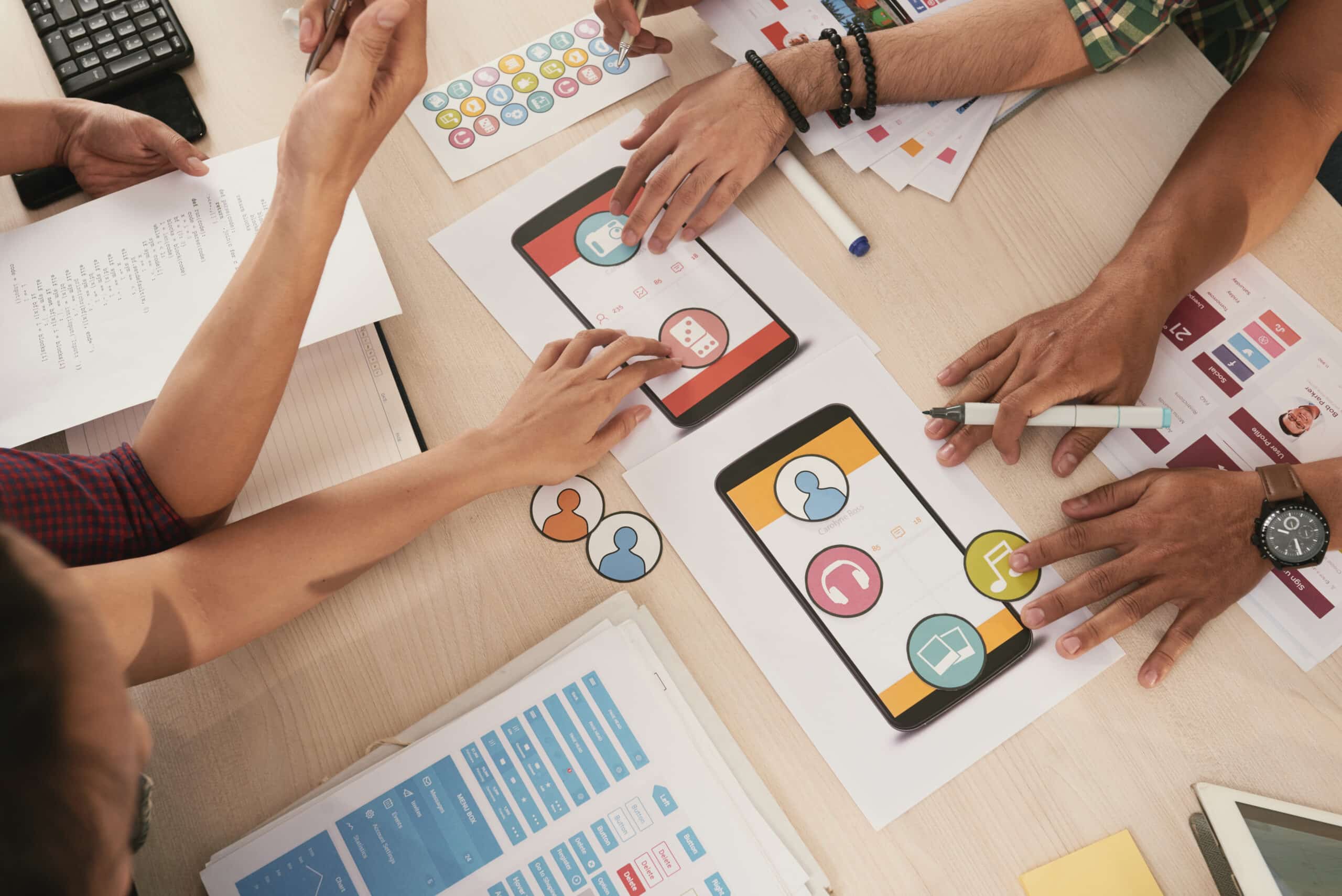
The benefits of a three-layered application architecture
Get started with vfunction.
See how vFunction can accelerate engineering velocity and increase application resiliency and scalability at your organization.
What is Presentation Logic? Complete Guide
Logic is the foundation of all web applications . It is the backbone of all business applications, from websites to mobile apps . In addition to being a crucial component of a web application, presentation logic is also crucial for the development of interactive experiences. Essentially, presentation logic is a way to present information to users in the most efficient manner possible. Using the proper presentation logic, an application can improve its user experience and improve sales results.
While business logic consists of the rules of a company, presentation logic refers to how the details of an application are displayed. Both are important but must be considered separately. A business application can’t operate without either. It must adhere to both of these standards to keep users happy. In addition, it should also be easy to use. There are many types of presentation logic. Some are easier to use than others, but they are both important in any application.
The two most common types of presentation logic are business logic and presentation logic. The latter is the most general type of presentation logic. It outlines the steps necessary to complete an action. Similarly, presentation logically explains how the business objects are presented to the user. When it is properly implemented, it will ensure that users retain the information. It will also ensure that the audience is able to understand the content of the application.
In simple terms , presentation logic is the processing required to create a print-out. It is also called business logic. It is an important part of an application, as it can define the business rules and actions that should be performed. It is also known as user interface. This layer is the foundation of the entire system. It’s where the business rules are implemented. Usually, presentation logic is implemented in the client side of a web-based application .
Presentation logic is the core of the application. The business logic layer provides a foundation for the user to interact with the application. It also contains all the business rules for the server. By contrast, presentation logic is the underlying code that determines how the data is presented. In contrast to business rules, presentation logic is the “rules” part. Instead of business rules, the logic layer implements the data stored on the server.
Presentation Layer
The presentation layer is a component of the software architecture that handles the user interface (UI) of an application. It is the layer that the end-users interact with, and its primary function is to present information to the user in a way that is understandable and easy to use.
The Presentation Layer has an essential role in software architecture. It is the layer that creates the first impression of the application for the users, and it affects the usability and user experience of the software. A well-designed presentation layer can make a significant difference in the success of the application.
Presentation Logic
Presentation Logic is the part of software logic responsible for determining how the user interface components of an application should behave and interact with each other. It defines how the user interface will be presented to the user and how it will respond to user input.
Features of Presentation Logic
Presentation Logic has several features that are essential to ensure its effectiveness in software development. These features include separation of concerns, reusability, testability, and maintainability.
Separation of Concerns
Reusability.
Presentation Logic should be designed to be reusable across different parts of the application. It allows for code reduction, faster development, and a consistent user experience. Reusable Presentation Logic components, such as form validation or navigation, can be shared across different screens or pages of the application, resulting in code optimization and reducing the time and effort required for development.
Testability
Presentation Logic should be designed to be easily testable to ensure its reliability and quality. Testability allows for quick and efficient debugging and helps to identify issues early in the development process. The Presentation Logic should be separated from the user interface components, allowing for the use of automated testing tools and frameworks to test its functionality.
Maintainability
Benefits of presentation logic.
Presentation Logic plays a crucial role in software development and provides several benefits to developers and end-users. These benefits include increased user experience, improved performance, reduced development time, and easier maintenance.
Increased User Experience
Improved performance, reduced development time.
Presentation Logic can significantly reduce the development time required for an application. By using reusable components, developers can quickly develop and integrate new features into their applications. By separating Presentation Logic from business logic and data access logic, developers can work on each layer independently, allowing for parallel development and faster iteration.
Easier Maintenance
Best practices for implementing presentation logic.
To implement Presentation Logic effectively, developers should follow certain best practices to ensure that the code is efficient, maintainable, and scalable. The following are some of the best practices for implementing Presentation Logic.
Follow the Separation of Concerns Principle
One of the most important best practices for implementing Presentation Logic is to follow the Separation of Concerns principle. This principle involves separating different concerns of an application into distinct modules or layers. By separating Presentation Logic from business logic and data access logic, developers can reduce the complexity of the codebase and make it easier to maintain and modify.
Use a Design Pattern
Use data binding.
Data binding is a technique that allows developers to bind data properties directly to user interface elements. This technique makes it easier to update the user interface elements when the underlying data changes. Using data binding can significantly reduce the amount of code required to implement Presentation Logic.
Use Reusable Components
Developers should aim to create reusable components for Presentation Logic wherever possible. This approach allows developers to use the same components across multiple screens or pages, reducing the amount of code required and promoting consistency in the user interface. Reusable components also make it easier to modify or update the Presentation Logic in the future.
Follow Coding Best Practices
Use automated testing.
Automated testing is an essential practice for implementing Presentation Logic effectively. Developers should write automated tests for their Presentation Logic to ensure that it is functioning as expected and to catch bugs and errors early in the development process. Automated testing can also help to reduce the amount of time and effort required for manual testing.
Frequently asked questions
What is presentation logic vs application logic.
Presentation logic and application logic are both important components of a software system, but they serve different purposes.
Application logic, on the other hand, refers to the code that controls the underlying functionality of the application. This includes the algorithms and business logic that handle data processing, calculations, and other tasks that are essential to the application’s core functionality. The application logic is responsible for ensuring that the application functions correctly and efficiently.
To summarize, presentation logic is concerned with how the application looks and behaves, while application logic is concerned with what the application does. Both are important components of a software system, and they work together to create a seamless user experience.
What is the presentation logic components?
What is the importance of presentation logic.
The presentation logic of an application is important for several reasons:
In conclusion, Presentation Logic is a critical aspect of software development that can significantly impact the user experience, performance, and maintainability of an application. By implementing Presentation Logic effectively and following best practices, developers can create efficient, scalable, and user-friendly applications that meet the needs of their users.
Leave a Reply Cancel reply
Related posts, the future of transportation and logistics: innovations on the horizon, what is cloud migration and what are its benefits, exploring the world of streaming: tips for aspiring streamers, leveraging machine learning and data visualization for enhanced wildlife conservation, technology in sports journalism, exploring the design and applications of conductive inks in printed electronics.
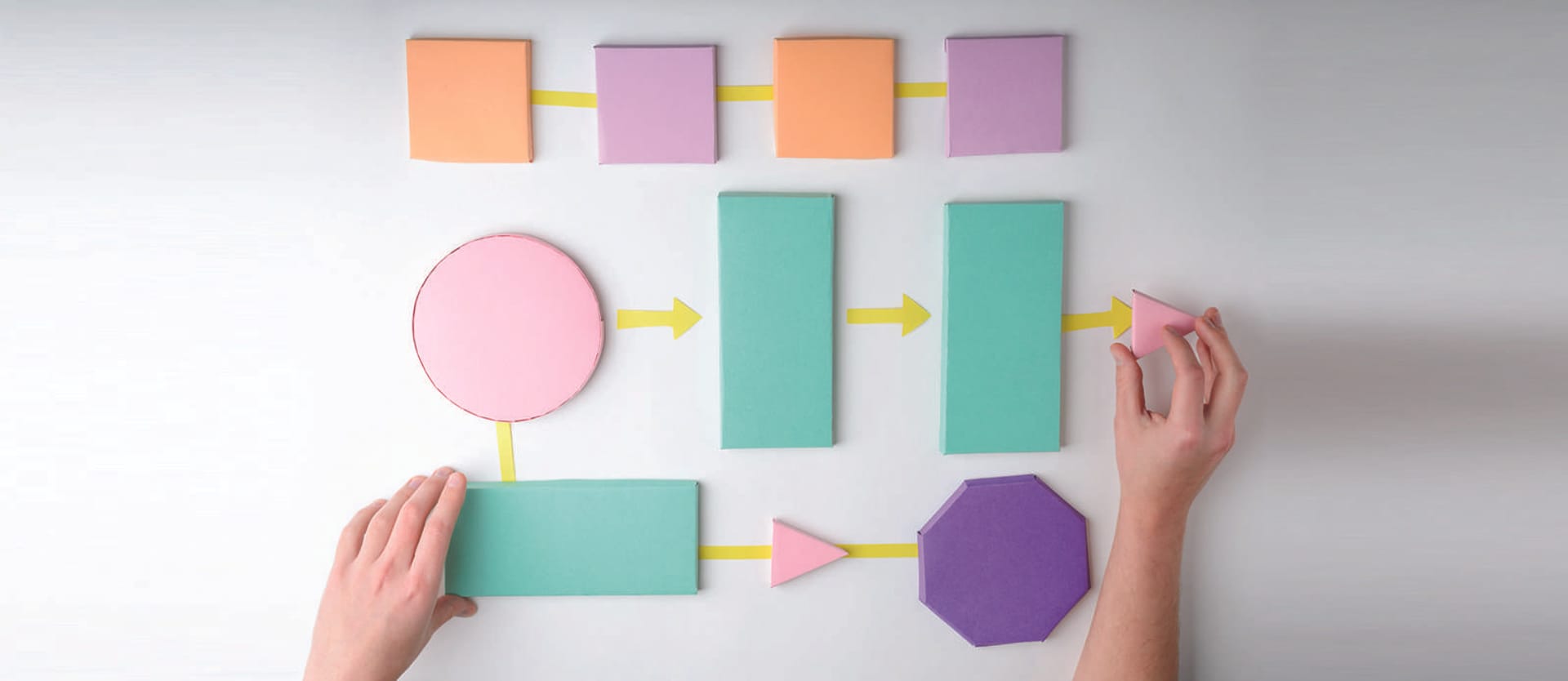
Web Application Architecture: How the Web Works
- Engineering
- Published: 25 Jul, 2019
- No comments Share
When building a web application, there are three main principles to bear in mind. From a customer’s point of view, the application should be simple, aesthetically pleasing, and address most of their problems. From the business aspect, a web application should stay aligned with its product/market fit . From a software engineer’s perspective, a web application should be scalable, functional, and able to withstand high traffic loads. All these issues are addressed in the web application’s architecture. We’ll cover the basic concepts of any modern web application and explain how the architecture patterns may differ depending on the application you’re building.
What is Web Application Architecture?
So, what is a web application, and how is it different from a website? The basic definition of a web application is a program that runs on a browser. It’s not a website, but the line between the two is fuzzy. To differentiate a web application from a website, remember these three formal characteristics. A web application:
- addresses a particular problem, even if it’s simply finding some information
- is as interactive as a desktop application
- has a Content Management System
A website is traditionally understood to simply be a combination of static pages. But today, most websites consist of both static and dynamic pages, which makes almost all modern websites - you guessed it! - web applications. In this article, we will use the terms interchangeably. Your computer, or smartphone, or any other device you’re browsing with is called a client. The other half of the web equation is called a server because it serves you the data you request. Their communication is called a client-server model, whose main concern is receiving your request and delivering the response back. Web application architecture is a mechanism that determines how application components communicate with each other. Or, in other words, the way the client and the server are connected is established by web application architecture. Web applications of different sizes and complexity levels all follow the same architectural principle, but details may differ. We will further explain how a basic request-response process works and what components comprise the architecture.
How does the web request work?
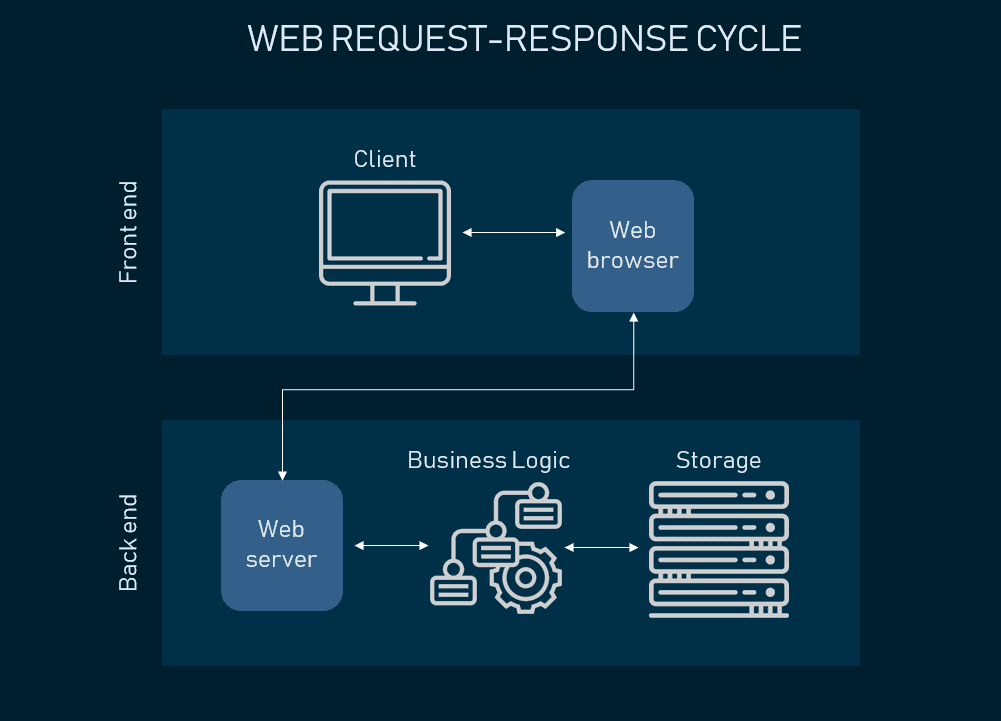
Web request-response cycle
Let’s look at Amazon.com to illustrate our explanation. First, you visit amazon.com . You type in the URL and as you hit Enter, your browser prepares to recognize this URL, because it needs to know the address of the server where the page is located. So it sends your request to the Domain Name Center (DNS), a repository of domain names and their IP addresses. If you've already visited Amazon from the same browser, it will pull the address from the cache. Then, a browser sends the request to the found IP address using the HTTPS protocol. Second, the web server processes the request . The web server where Amazon.com is located catches the request and sends it to the storage area to locate the page and all data that follows with it. But its route is held via Business Logic (also called Domain Logic and Application Logic). BL manages how each piece of data is accessed and determines this workflow specifically for each application . As BL processes the request, it sends it to storage to locate the looked-for data. Third, you receive your data . Your response travels back to you and you see the content of the web page on your display. The graphical interface you see when scrolling Amazon's or any other website is called the front end of an application - it depicts all UX and UI components so that a user can access the information they came looking for.
Web application architecture components and Three-Tier Architecture
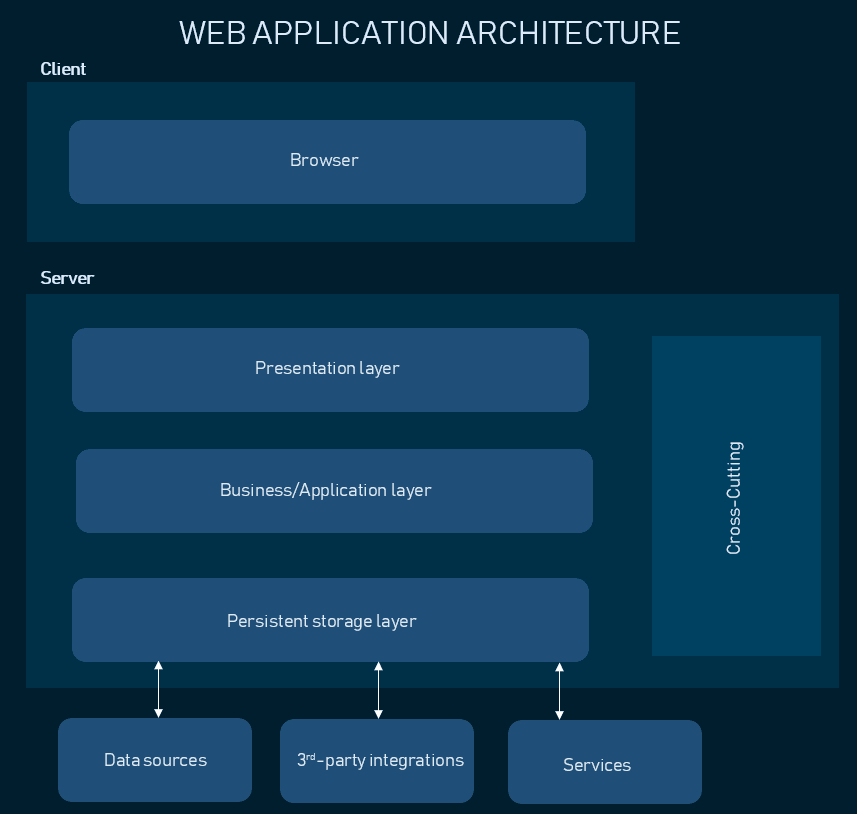
Web application architecture following the three-tier pattern
Presentation layer
The presentation layer is accessible to users via a browser and consists of user interface components and UI process components that support interaction with the system. It’s developed using three core technologies: HTML, CSS, and JavaScript. While HTML is the code that determines what your website will contain, CSS controls how it will look. JavaScript and its frameworks make your website interactive - responsive to a user’s actions. Developers use JavaScript frameworks such as Angular and React to make the content on the page dynamic.
Business layer
This layer, also called Business Logic or Domain Logic or Application Layer, accepts user requests from the browser, processes them, and determines the routes through which the data will be accessed. The workflows by which the data and requests travel through the back end are encoded in a business layer. For example, if your application is a hotel booking website, business logic will be responsible for the sequence of events a traveler will go through when booking a room. Although business rules can be a manifestation of the business logic, they are not the same. Sometimes business rules are extracted and managed separately, using a Business Rules Management System, as we discussed in our article on back office systems .
Persistence layer
Also called the storage or data access layer, the persistance layer is a centralized location that receives all data calls and provides access to the persistent storage of an application. The persistence layer is closely connected to the business layer, so the logic knows which database to talk to and the data retrieving process is more optimized. The data storage infrastructure includes a server and a Database Management System , software to communicate with the database itself, applications, and user interfaces to obtain data and parse it. Typically you can store your data either in owned hardware servers or in the cloud - meaning, that you purchase data center management and maintenance services while accessing your storage virtually. Using the services of cloud technology providers such as Amazon, Google, or Microsoft, you can utilize Infrastructure-as-a-Service, Platform-as-a-Service, or serverless approaches to cloud management. There are also components that usually exist in all web applications but are separated from the main layers: Cross-cutting code. This component handles other application concerns such as communications, operational management, and security. It affects all parts of the system but should never mix with them. Third-party integrations . Payment gateways, social logins, GDSs in travel websites are all integrations connected to the application’s back end via pieces of code called APIs. They allow your software to source data from other software and widen your functionality without coding it from scratch. Read how APIs work in our dedicated article. Let’s see how the three-tier architecture is implemented in different types of web applications.
Example #1. Dynamic web pages, SPAs, and MPAs
The application’s front end can serve either static or dynamic content. In most cases, it’s a combination of both. Static Web Pages exist on a server as they are and contain information that doesn’t change. Dynamic Web Pages change information every day or in response to a user’s request - think of any news website or your Twitter feed. The combination of dynamic and static content makes up a web application. The simplest example of a web application with dynamic content is a Single Page Application.
Single Page Applications
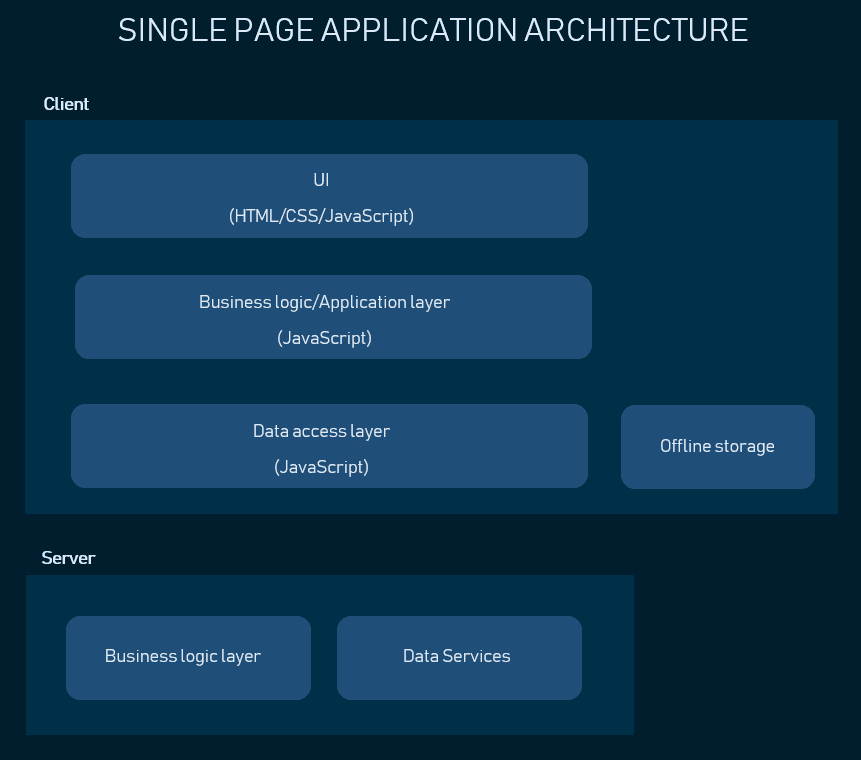
Single Page Application architecture
When the role of the server is reduced to data services, this is sometimes called thin server architecture. We can’t talk about SPAs without mentioning the more traditional model - Multi-Page Applications.
Multi-Page Applications
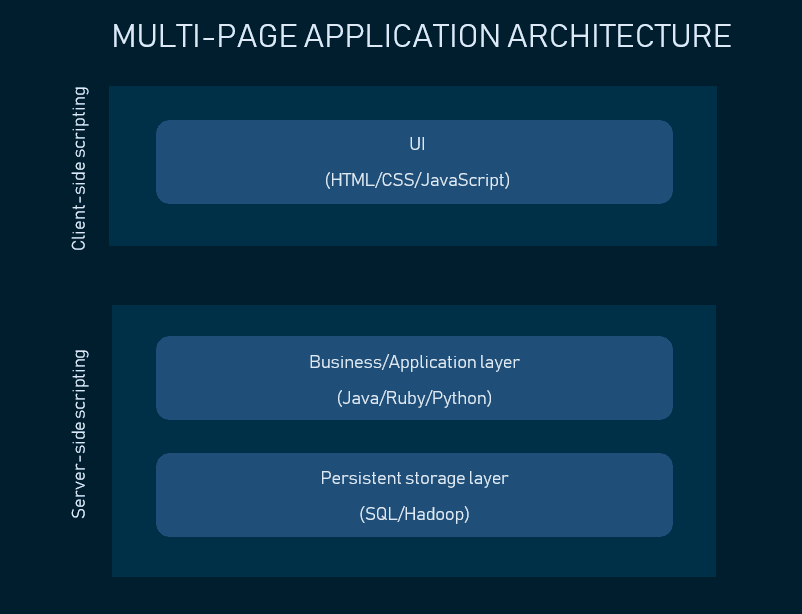
MPA architecture
As opposed to the SPA’s client-side scripting, traditional applications are written using both client- and server-side languages. Server-side scripting means that all operations are performed on the server’s end, so when you request content, it processes the script, retrieves data from the storage and chooses the content to display. Server-scripting languages you should be familiar with include PHP, Java , Python, Ruby, C#, and more.
Example #2. Enterprise applications
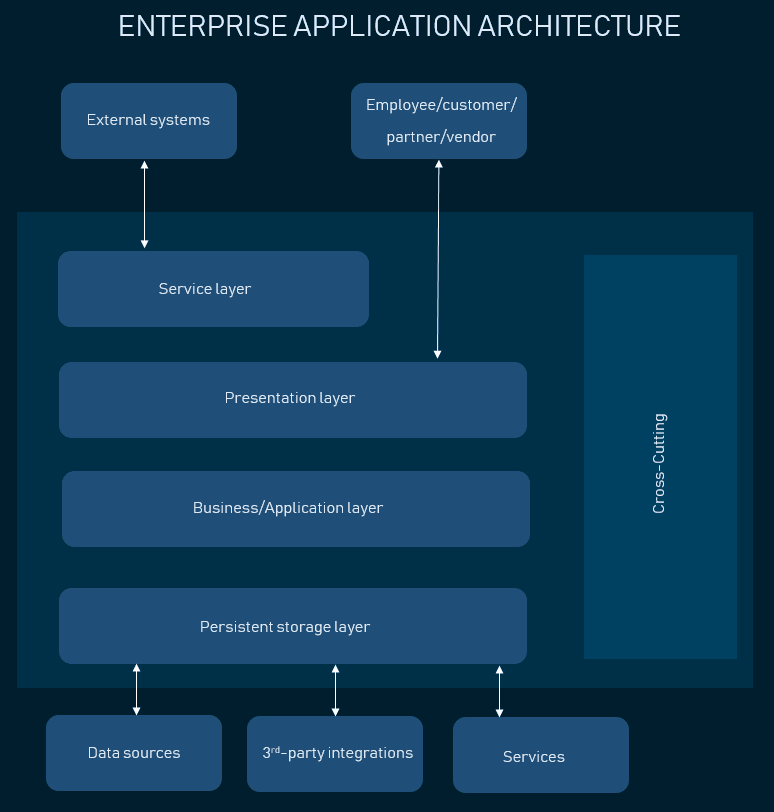
Enterprise application architecture
Apart from an extra layer, enterprise applications have access to data sources from other applications in an organization, making it a network of software solutions, connected by APIs. Besides, there are more groups of users who have access to different functional components - they can be business partners, clients, admins, and several groups of staff. Sometimes the presentation tiers are separate for all of them, so you can deploy the application as intranet or extranet.
To conclude
This, hopefully, sheds some light on the backstage of building modern websites. In this article, we dipped our toes into the complicated subject of software engineering. If this wasn’t enough for you, feel free to roam around our blog a bit more and specifically explore the following articles. The Good and the Bad of JavaScript Full Stack Development Technical Documentation in Software Development: Types, Best Practices, and Tools How to Use Open Source Software: Features, Main Software Types, and Selection Advice
Application Architecture Guide - Chapter 10 - Presentation Layer Guidelines
Note - The patterns & practices Microsoft Application Architecture Guide, 2nd Edition is now live at http://msdn.microsoft.com/en-us/library/dd673617.aspx .
- J.D. Meier, Alex Homer, David Hill, Jason Taylor, Prashant Bansode, Lonnie Wall, Rob Boucher Jr, Akshay Bogawat
- 1 Objectives
- 3 Presentation Layer Components
- 5 Design Considerations
- 6 Presentation Layer Frame
- 8 Composition
- 9 Exception Management
- 12 Navigation
- 13 Presentation Entities
- 14 Request Processing
- 15 User Experience
- 16 UI Components
- 17 UI Process Components
- 18 Validation
- 19 Pattern Map
- 20 Pattern Descriptions
- 21.1 Mobile Applications
- 21.2 Rich Client Applications
- 21.3 Rich Internet Applications (RIA)
- 21.4 Web Applications
- 22 patterns & practices Solution Assets
- 23 Additional Resources
- Understand how the presentation layer fits into typical application architecture.
- Understand the components of the presentation layer.
- Learn the steps for designing the presentation layer.
- Learn the common issues faced while designing the presentation layer.
- Learn the key guidelines for designing the presentation layer.
- Learn the key patterns and technology considerations for designing the presentation layer.
The presentation layer contains the components that implement and display the user interface and manage user interaction. This layer includes controls for user input and display, in addition to components that organize user interaction. Figure 1 shows how the presentation layer fits into a common application architecture.
Figure 1 A typical application showing the presentation layer and the components it may contain
Presentation Layer Components
- User interface (UI) components . User interface components provide a way for users to interact with the application. They render and format data for users. They also acquire and validate data input by the user.
- User process components . User process components synchronize and orchestrate user interactions. Separate user process components may be useful if you have a complicated UI. Implementing common user interaction patterns as separate user process components allows you to reuse them in multiple UIs.
The following steps describe the process you should adopt when designing the presentation layer for your application. This approach will ensure that you consider all of the relevant factors as you develop your architecture:
- Identify your client type . Choose a client type that satisfies your requirements and adheres to the infrastructure and deployment constraints of your organization. For instance, if your users are on mobile devices and will be intermittently connected to the network, a mobile rich client is probably your best choice.
- Determine how you will present data . Choose the data format for your presentation layer and decide how you will present the data in your UI.
- Determine your data-validation strategy . Use data-validation techniques to protect your system from untrusted input.
- Determine your business logic strategy . Factor out your business logic to decouple it from your presentation layer code.
- Determine your strategy for communication with other layers . If your application has multiple layers, such as a data access layer and a business layer, determine a strategy for communication between your presentation layer and other layers.
Design Considerations
There are several key factors that you should consider when designing your presentation layer. Use the following principles to ensure that your design meets the requirements for your application, and follows best practices:
- Choose the appropriate UI technology. Determine if you will implement a rich (smart) client, a Web client, or a rich Internet application (RIA). Base your decision on application requirements, and on organizational and infrastructure constraints.
- Use the relevant patterns. Review the presentation layer patterns for proven solutions to common presentation problems.
- Design for separation of concerns. Use dedicated UI components that focus on rendering and display. Use dedicated presentation entities to manage the data required to present your views. Use dedicated UI process components to manage the processing of user interaction.
- Consider human interface guidelines. Review your organization’s guidelines for UI design. Review established UI guidelines based on the client type and technologies that you have chosen.
- Adhere to user-driven design principles. Before designing your presentation layer, understand your customer. Use surveys, usability studies, and interviews to determine the best presentation design to meet your customer’s requirements.

Presentation Layer Frame
There are several common issues that you must consider as your develop your design. These issues can be categorized into specific areas of the design. The following table lists the common issues for each category where mistakes are most often made.
Table 1 Presentation Layer Frame
* Caching volatile data. | |
* Failing to consider use of patterns and libraries that support dynamic layout and injection of views and presentation at runtime. | |
* Failing to catch unhandled exceptions. | |
* Failing to design for intuitive use, or implementing overly complex interfaces. | |
* Using an inappropriate layout style for Web pages. | |
* Inconsistent navigation. | |
* Defining entities that are not necessary. | |
* Blocking the UI during long-running requests. | |
* Displaying unhelpful error messages. | |
* Creating custom components that are not necessary. | |
* Implementing UI process components when not necessary. | |
* Failing to validate all input. |
Caching is one of the best mechanisms you can use to improve application performance and UI responsiveness. Use data caching to optimize data lookups and avoid network round trips. Cache the results of expensive or repetitive processes to avoid unnecessary duplicate processing.
Consider the following guidelines when designing your caching strategy:
- Do not cache volatile data.
- Consider using ready-to-use cache data when working with an in-memory cache. For example, use a specific object instead of caching raw database data.
- Do not cache sensitive data unless you encrypt it.
- If your application is deployed in Web farm, avoid using local caches that need to be synchronized; instead, consider using a transactional resource manager such as Microsoft SQL Server® or a product that supports distributed caching.
- Do not depend on data still being in your cache. It may have been removed.
Composition
Consider whether your application will be easier to develop and maintain if the presentation layer uses independent modules and views that are easily composed at run time. Composition patterns support the creation of views and the presentation layout at run time. These patterns also help to minimize code and library dependencies that would otherwise force recompilation and redeployment of a module when the dependencies change. Composition patterns help you to implement sharing, reuse, and replacement of presentation logic and views.
Consider the following guidelines when designing your composition strategy:
- Avoid using dynamic layouts. They can be difficult to load and maintain.
- Be careful with dependencies between components. For example, use abstraction patterns when possible to avoid issues with maintainability.
- Consider creating templates with placeholders. For example, use the Template View pattern to compose dynamic Web pages in order to ensure reuse and consistency.
- Consider composing views from reusable modular parts. For example, use the Composite View pattern to build a view from modular, atomic component parts.
- If you need to allow communication between presentation components, consider implementing the Publish/Subscribe pattern. This will lower the coupling between the components and improve testability.
Exception Management
Design a centralized exception-management mechanism for your application that catches and throws exceptions consistently. Pay particular attention to exceptions that propagate across layer or tier boundaries, as well as exceptions that cross trust boundaries. Design for unhandled exceptions so they do not impact application reliability or expose sensitive information.
Consider the following guidelines when designing your exception management strategy:
- Use user-friendly error messages to notify users of errors in the application.
- Avoid exposing sensitive data in error pages, error messages, log files, and audit files.
- Design a global exception handler that displays a global error page or an error message for all unhandled exceptions.
- Differentiate between system exceptions and business errors. In the case of business errors, display a user-friendly error message and allow the user to retry the operation. In the case of system exceptions, check to see if the exception was caused by issues such as system or database failure, display a user-friendly error message, and log the error message, which will help in troubleshooting.
- Avoid using exceptions to control application logic.
Design a user input strategy based on your application input requirements. For maximum usability, follow the established guidelines defined in your organization, and the many established industry usability guidelines based on years of user research into input design and mechanisms.
Consider the following guidelines when designing your input collection strategy:
- Use forms-based input controls for normal data-collection tasks.
- Use a document-based input mechanism for collecting input in Microsoft Office–style documents.
- Implement a wizard-based approach for more complex data collection tasks, or for input that requires a workflow.
- Design to support localization by avoiding hard-coded strings and using external resources for text and layout.
- Consider accessibility in your design. You should consider users with disabilities when designing your input strategy; for example, implement text-to-speech software for blind users, or enlarge text and images for users with poor sight. Support keyboard-only scenarios where possible for users who cannot manipulate a pointing device.
Design your UI layout so that the layout mechanism itself is separate from the individual UI components and UI process components. When choosing a layout strategy, consider whether you will have a separate team of designers building the layout, or whether the development team will create the UI. If designers will be creating the UI, choose a layout approach that does not require code or the use of development-focused tools.
Consider the following guidelines when designing your layout strategy:
- Use templates to provide a common look and feel to all of the UI screens.
- Use a common look and feel for all elements of your UI to maximize accessibility and ease of use.
- Consider device-dependent input, such as touch screens, ink, or speech, in your layout. For example, with touch-screen input you will typically use larger buttons with more spacing between them than you would with mouse or keyboard inputs.
- When building a Web application, consider using Cascading Style Sheets (CSS) for layout. This will improve rendering performance and maintainability.
- Use design patterns, such as Model-View-Presenter (MVP), to separate the layout design from interface processing.
Design your navigation strategy so that users can navigate easily through your screens or pages, and so that you can separate navigation from presentation and UI processing. Ensure that you display navigation links and controls in a consistent way throughout your application to reduce user confusion and hide application complexity.
Consider the following guidelines when designing your navigation strategy:
- Use well-known design patterns to decouple the UI from the navigation logic where this logic is complex.
- Design toolbars and menus to help users find functionality provided by the UI.
- Consider using wizards to implement navigation between forms in a predictable way.
- Determine how you will preserve navigation state if the application must preserve this state between sessions.
- Consider using the Command Pattern to handle common actions from multiple sources.
Presentation Entities
Use presentation entities to store the data you will use in your presentation layer to manage your views. Presentation entities are not always necessary; use them only if your datasets are sufficiently large and complex to require separate storage from the UI controls.
Consider the following guidelines when designing presentation entities:
- Determine if you require presentation entities. Typically, you may require presentation entities only if the data or the format to be displayed is specific to the presentation layer.
- If you are working with data-bound controls, consider using custom objects, collections, or datasets as your presentation entity format.
- If you want to map data directly to business entities, use a custom class for your presentation entities.
- Do not add business logic to presentation entities.
- If you need to perform data type validation, consider adding it in your presentation entities.
Request Processing
Design your request processing with user responsiveness in mind, as well as code maintainability and testability.
Consider the following guidelines when designing request processing:
- Use asynchronous operations or worker threads to avoid blocking the UI for long-running actions.
- Avoid mixing your UI processing and rendering logic.
- Consider using the Passive View pattern (a variant of MVP) for interfaces that do not manage a lot of data.
- Consider using the Supervising Controller pattern (a variant of MVP) for interfaces that manage large amounts of data.
User Experience
Good user experience can make the difference between a usable and unusable application. Carry out usability studies, surveys, and interviews to understand what users require and expect from your application, and design with these results in mind.
Consider the following guidelines when designing for user experience:
- When developing a rich Internet application (RIA), avoid synchronous processing where possible.
- When developing a Web application, consider using Asynchronous JavaScript and XML (AJAX) to improve responsiveness and to reduce post backs and page reloads.
- Do not design overloaded or overly complex interfaces. Provide a clear path through the application for each key user scenario.
- Design to support user personalization, localization, and accessibility.
- Design for user empowerment. Allow the user to control how he or she interacts with the application, and how it displays data to them.
UI Components
UI components are the controls and components used to display information to the user and accept user input. Be careful not to create custom controls unless it is necessary for specialized display or data collection.
Consider the following guidelines when designing UI components:
- Take advantage of the data-binding features of the controls you use in the UI.
- Create custom controls or use third-party controls only for specialized display and data-collection tasks.
- When creating custom controls, extend existing controls if possible instead of creating a new control.
- Consider implementing designer support for custom controls to make it easier to develop with them.
- Consider maintaining the state of controls as the user interacts with the application instead of reloading controls with each action.
UI Process Components
UI process components synchronize and orchestrate user interactions. UI processing components are not always necessary; create them only if you need to perform significant processing in the presentation layer that must be separated from the UI controls. Be careful not to mix business and display logic within the process components; they should be focused on organizing user interactions with your UI.
Consider the following guidelines when designing UI processing components:
- Do not create UI process components unless you need them.
- If your UI requires complex processing or needs to talk to other layers, use UI process components to decouple this processing from the UI.
- Consider dividing UI processing into three distinct roles: Model, View, and Controller/Presenter, by using the MVC or MVP pattern.
- Avoid business rules, with the exception of input and data validation, in UI processing components.
- Consider using abstraction patterns, such as dependency inversion, when UI processing behavior needs to change based on the run-time environment.
- Where the UI requires complex workflow support, create separate workflow components that use a workflow system such as Windows Workflow or a custom mechanism.
Designing an effective input and data-validation strategy is critical to the security of your application. Determine the validation rules for user input as well as for business rules that exist in the presentation layer.
Consider the following guidelines when designing your input and data validation strategy:
- Validate all input data on the client side where possible to improve interactivity and reduce errors caused by invalid data.
- Do not rely on client-side validation only. Always use server-side validation to constrain input for security purposes and to make security-related decisions.
- Design your validation strategy to constrain, reject, and sanitize malicious input.
- Use the built-in validation controls where possible, when working with .NET Framework.
- In Web applications, consider using AJAX to provide real-time validation.
Pattern Map
Key patterns are organized by key categories, as detailed in the Presentation Layer Frame in the following table. Consider using these patterns when making design decisions for each category.
Table 2 Pattern Map
* Cache Dependency | |
* Composite View | |
* Exception Shielding | |
* Template View | |
* Front Controller | |
* Entity Translator | |
* Asynchronous Callback | |
* Model-View-Controller (MVC) |
- For more information on the Page Cache pattern, see “Enterprise Solution Patterns Using Microsoft .NET” at http://msdn.microsoft.com/en-us/library/ms998469.aspx
- For more information on the Model-View-Controller (MVC), Page Controller, Front Controller, Template View, Transform View, and Two-Step View patterns, see “Patterns of Enterprise Application Architecture (P of EAA)” at http://martinfowler.com/eaaCatalog/
- For more information on the Composite View, Supervising Controller, and Presentation Model patterns, see “Patterns in the Composite Application Library” at http://msdn.microsoft.com/en-us/library/cc707841.aspx
- For more information on the Chain of responsibility and Command pattern, see “data & object factory” at http://www.dofactory.com/Patterns/Patterns.aspx
- For more information on the Asynchronous Callback pattern, see “Creating a Simplified Asynchronous Call Pattern for Windows Forms Applications” at http://msdn.microsoft.com/en-us/library/ms996483.aspx
- For more information on the Exception Shielding and Entity Translator patterns, see “Useful Patterns for Services” at http://msdn.microsoft.com/en-us/library/cc304800.aspx
Pattern Descriptions
- Asynchronous Callback. Execute long-running tasks on a separate thread that executes in the background, and provide a function for the thread to call back into when the task is complete.
- Cache Dependency. Use external information to determine the state of data stored in a cache.
- Chain of Responsibility. Avoid coupling the sender of a request to its receiver by giving more than one object a chance to handle the request.
- Composite View . Combine individual views into a composite representation.
- Command Pattern. Encapsulate request processing in a separate command object with a common execution interface.
- Entity Translator. An object that transforms message data types into business types for requests, and reverses the transformation for responses.
- Exception Shielding. Prevent a service from exposing information about its internal implementation when an exception occurs.
- Front Controller . Consolidate request handling by channeling all requests through a single handler object, which can be modified at run time with decorators.
- Model-View-Controller . Separate the UI code into three separate units: Model (data), View (interface), and Presenter (processing logic), with a focus on the View. Two variations on this pattern include Passive View and Supervising Controller, which define how the View interacts with the Model.
- Page Cache. Improve the response time for dynamic Web pages that are accessed frequently but change less often and consume a large amount of system resources to construct.
- Page Controller . Accept input from the request and handle it for a specific page or action on a Web site.
- Passive View . Reduce the view to the absolute minimum by allowing the controller to process user input and maintain the responsibility for updating the view.
- Presentation Model . Move all view logic and state out of the view, and render the view through data-binding and templates.
- Supervising Controller . A variation of the MVC pattern in which the controller handles complex logic, in particular coordinating between views, but the view is responsible for simple view-specific logic.
- Template View . Implement a common template view, and derive or construct views using this template view.
- Transform View . Transform the data passed to the presentation tier into HTML for display in the UI.
- Two-Step View . Transform the model data into a logical presentation without any specific formatting, and then convert that logical presentation to add the actual formatting required.
Technology Considerations
The following guidelines will help you to choose an appropriate implementation technology. The guidelines also contain suggestions for common patterns that are useful for specific types of application and technology.
Mobile Applications
Consider the following guidelines when designing a mobile application:
- If you want to build full-featured connected, occasionally connected, and disconnected executable applications that run on a wide range of Microsoft Windows®–based devices, consider using the Microsoft Windows Compact Framework.
- If you want to build connected applications that require Wireless Application Protocol (WAP), compact HTML (cHTML), or similar rendering formats, consider using ASP.NET Mobile Forms and Mobile Controls.
- If you want to build applications that support rich media and interactivity, consider using Microsoft Silverlight® for Mobile.
Rich Client Applications
Consider the following guidelines when designing a rich client application:
- If you want to build applications with good performance and interactivity, and have design support in Microsoft Visual Studio®, consider using Windows Forms.
- If you want to build applications that fully support rich media and graphics, consider using Windows Presentation Foundation (WPF).
- If you want to build applications that are downloaded from a Web server and then execute on the client, consider using XAML Browser Applications (XBAP).
- If you want to build applications that are predominantly document-based, or are used for reporting, consider designing a Microsoft Office Business Application.
- If you decide to use Windows Forms and you are designing composite interfaces, consider using the Smart Client Software Factory.
- If you decide to use WPF and you are designing composite interfaces, consider using the Composite Application Guidance for WPF.
- If you decide to use WPF, consider using the Presentation Model (Model-View-ViewModel) pattern.
- If you decide to use WPF, consider using WPF Commands to communicate between your View and your Presenter or ViewModel.
- If you decide to use WPF, consider implementing the Presentation Model pattern by using DataTemplates over User Controls to give designers more control.
Rich Internet Applications (RIA)
Consider the following guidelines when designing an RIA:
- If you want to build browser-based, connected applications that have broad cross-platform reach, are highly graphical, and support rich media and presentation features, consider using Silverlight.
- If you decide to use Silverlight, consider using the Presentation Model (Model-View-ViewModel) pattern.
Web Applications
Consider the following guidelines when designing a Web application:
- If you want to build applications that are accessed through a Web browser or specialist user agent, consider using ASP.NET.
- If you want to build applications that provide increased interactivity and background processing, with fewer page reloads, consider using ASP.NET with AJAX.
- If you want to build applications that include islands of rich media content and interactivity, consider using ASP.NET with Silverlight controls.
- If you are using ASP.NET and want to implement a control-centric model with separate controllers and improved testability, consider using the ASP.NET MVC Framework.
- If you are using ASP.NET, consider using master pages to simplify development and implement a consistent UI across all pages.
patterns & practices Solution Assets
- Web Client Software Factory at http://msdn.microsoft.com/en-us/library/bb264518.aspx
- Smart Client Software Factory at http://msdn.microsoft.com/en-us/library/aa480482.aspx
- Composite Application Guidance for WPF at http://msdn.microsoft.com/en-us/library/cc707819.aspx
- Smart Client - Composite UI Application Block at http://msdn.microsoft.com/en-us/library/aa480450.aspx
Additional Resources
- For more information, see Microsoft Inductive User Interface Guidelines at http://msdn.microsoft.com/en-us/library/ms997506.aspx .
- For more information, see User Interface Control Guidelines at http://msdn.microsoft.com/en-us/library/bb158625.aspx .
- For more information, see User Interface Text Guidelines at http://msdn.microsoft.com/en-us/library/bb158574.aspx .
- For more information, see Design and Implementation Guidelines for Web Clients at http://msdn.microsoft.com/en-us/library/ms978631.aspx .
- For more information, see Web Presentation Patterns at http://msdn.microsoft.com/en-us/library/ms998516.aspx .
Navigation menu
Page actions.
- View source
Personal tools
- Community portal
- Current events
- Recent changes
- Random page
- What links here
- Related changes
- Special pages
- Printable version
- Permanent link
- Page information

- This page was last edited on 22 January 2010, at 07:50.
- Privacy policy
- About Guidance Share
- Disclaimers

Exploring the 3 layers of software interactions (APIs)
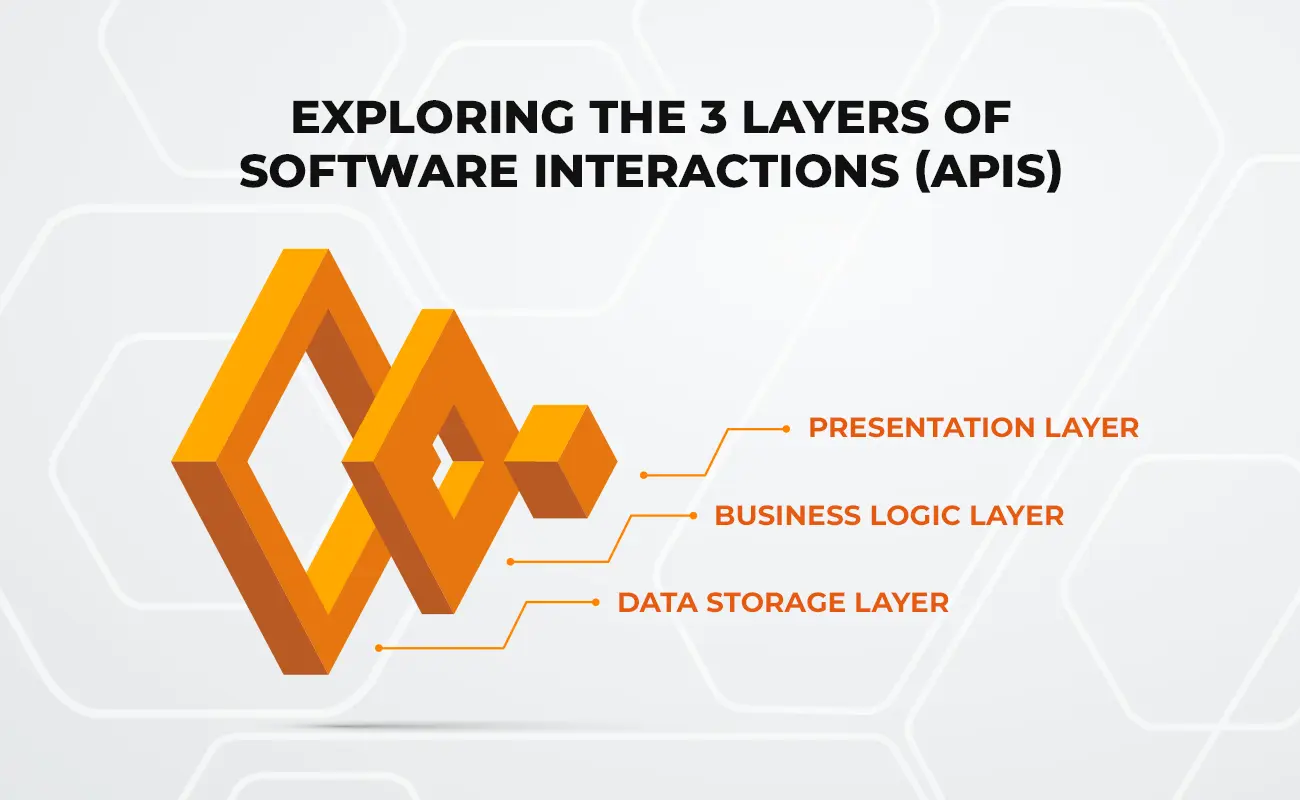
Application Programming Interfaces, commonly known as APIs, are a set of protocols, routines, and tools that enable software applications to interact with each other. They allow different software applications to exchange information seamlessly and efficiently.
APIs have become increasingly important in today's digital world, as they enable developers to build powerful applications that can connect with other services and platforms. APIs can be broken down into three different layers, each of which serves a different purpose.
In this blog post, we will explore the three layers of APIs and what they do.
1. Presentation Layer
The presentation layer, also known as the "API endpoint," is the layer that developers interact with most frequently. This layer provides a user-friendly interface for developers to access the functionality and data of the underlying service. It is responsible for processing incoming requests and returning responses in a format that developers can easily understand.
The presentation layer can take many different forms, depending on the requirements of the API. It could be a REST API that uses HTTP requests to exchange data or a SOAP API that uses XML messages to communicate. Regardless of the specific implementation, the presentation layer is the public-facing aspect of the API and is the first point of contact for developers.
2. Business Logic Layer
The business logic layer, also known as the "API middleware," is the layer that contains the core logic of the API. It is responsible for processing the requests received from the presentation layer and generating responses based on the requested actions.
The business logic layer is where the API's core functionality resides. It may perform data validation, authentication and authorization, database queries, or other complex operations. This layer is typically developed by the service provider and is not exposed directly to developers.
3. Data Storage Layer
The data storage layer, also known as the "API database," is the layer where all of the data used by the API is stored. This layer is responsible for managing data storage, retrieval, and modification. It may use a variety of database technologies such as SQL, NoSQL, or object-oriented databases.
The data storage layer is the most critical component of the API since it holds the data that the API relies on. If the data storage layer fails, the entire API may fail. Therefore, it is crucial to ensure that the data storage layer is designed and implemented correctly.
In conclusion, APIs consist of three layers: presentation layer, business logic layer, and data storage layer. Each layer plays a crucial role in the API's functionality and performance. Understanding the three layers of an API is essential for developers looking to build robust, reliable, and scalable APIs.
Related articles
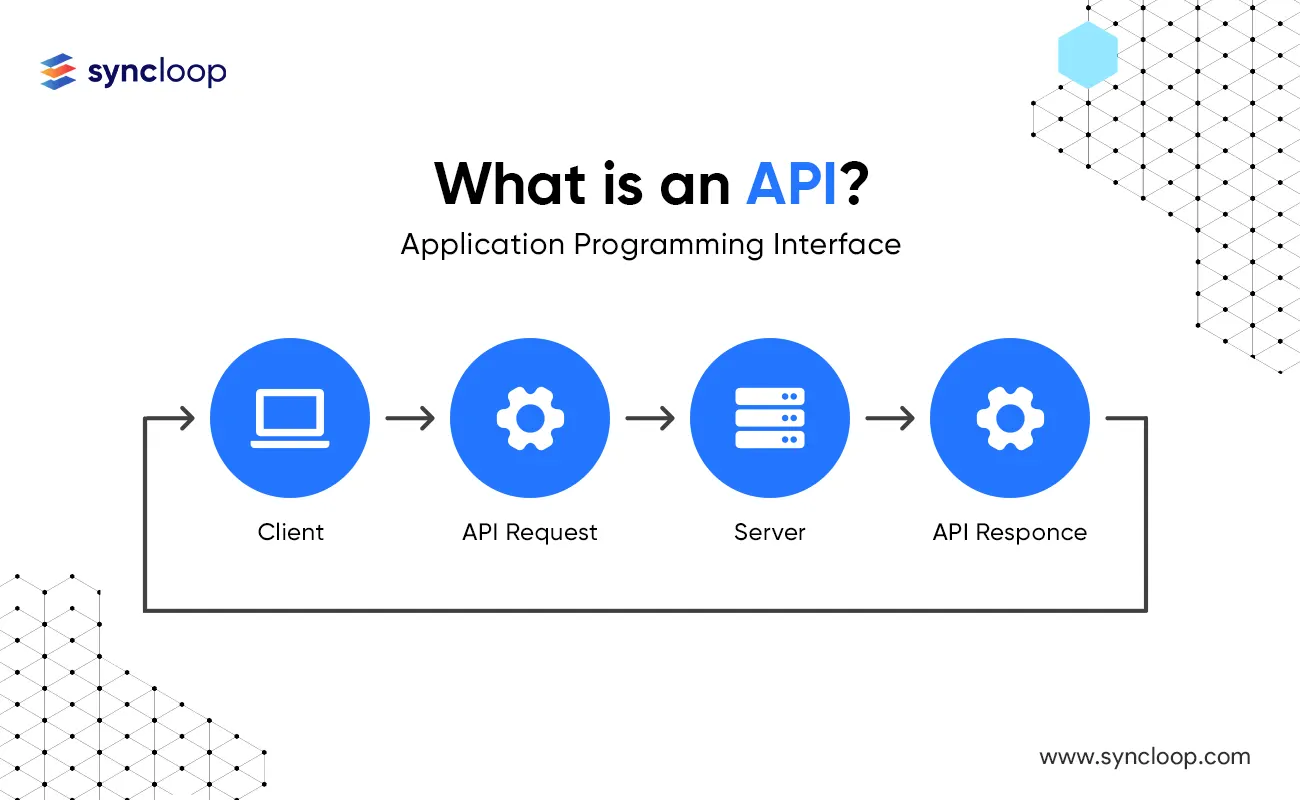
What is Application Programming Interface (API)?
An API is a set of rules and practices that allow two applications to communicate. This means that one can use one application to retrieve data from another application or send data to another application.

Three-tier architecture is a well-established software application architecture that organizes applications into three logical and physical computing tiers: the presentation tier, or user interface; the application tier, where data is processed; and the data tier, where application data is stored and managed.
The chief benefit of three-tier architecture is that because each tier runs on its own infrastructure, each tier can be developed simultaneously by a separate development team. And can be updated or scaled as needed without impacting the other tiers.
For decades three-tier architecture was the prevailing architecture for client-server applications. Today, most three-tier applications are targets for modernization that uses cloud-native technologies such as containers and microservices and for migration to the cloud.
Connect and integrate your systems to prepare your infrastructure for AI.
Register for the guide on app modernization
Presentation tier
The presentation tier is the user interface and communication layer of the application, where the end user interacts with the application. Its main purpose is to display information to and collect information from the user. This top-level tier can run on a web browser, as desktop application, or a graphical user interface (GUI), for example. Web presentation tiers are developed by using HTML, CSS, and JavaScript. Desktop applications can be written in various languages depending on the platform.
Application tier
The application tier, also known as the logic tier or middle tier, is the heart of the application. In this tier, information that is collected in the presentation tier is processed - sometimes against other information in the data tier - using business logic, a specific set of business rules. The application tier can also add, delete, or modify data in the data tier.
The application tier is typically developed by using Python, Java, Perl, PHP or Ruby, and communicates with the data tier by using API calls.
The data tier, sometimes called database tier, data access tier or back-end, is where the information that is processed by the application is stored and managed. This can be a relational database management system such as PostgreSQL , MySQL, MariaDB, Oracle, Db2, Informix or Microsoft SQL Server, or in a NoSQL Database server such as Cassandra, CouchDB , or MongoDB .
In a three-tier application, all communication goes through the application tier. The presentation tier and the data tier cannot communicate directly with one another.
Tier versus layer
In discussions of three-tier architecture, layer is often used interchangeably – and mistakenly – for tier , as in 'presentation layer' or 'business logic layer'.
They aren't the same. A 'layer' refers to a functional division of the software, but a 'tier' refers to a functional division of the software that runs on infrastructure separate from the other divisions. The Contacts app on your phone, for example, is a three - layer application, but a single-tier application, because all three layers run on your phone.
The difference is important because layers can't offer the same benefits as tiers.
Again, the chief benefit of three-tier architecture is its logical and physical separation of functionality. Each tier can run on a separate operating system and server platform - for example, web server, application server, database server - that best fits its functional requirements. And each tier runs on at least one dedicated server hardware or virtual server, so the services of each tier can be customized and optimized without impacting the other tiers.
Other benefits (compared to single- or two-tier architecture) include:
- Faster development : Because each tier can be developed simultaneously by different teams, an organization can bring the application to market faster. And programmers can use the latest and best languages and tools for each tier.
- Improved scalability : Any tier can be scaled independently of the others as needed.
- Improved reliability : An outage in one tier is less likely to impact the availability or performance of the other tiers.
- Improved security : Because the presentation tier and data tier can't communicate directly, a well-designed application tier can function as an internal firewall, preventing SQL injections and other malicious exploits.
In web development, the tiers have different names but perform similar functions:
- The web server is the presentation tier and provides the user interface. This is usually a web page or website, such as an ecommerce site where the user adds products to the shopping cart, adds payment details or creates an account. The content can be static or dynamic, and is developed using HTML, CSS, and JavaScript.
- The application server corresponds to the middle tier, housing the business logic that is used to process user inputs. To continue the ecommerce example, this is the tier that queries the inventory database to return product availability, or adds details to a customer's profile. This layer often developed using Python, Ruby, or PHP and runs a framework such as Django, Rails, Symphony, or ASP.NET.
- The database server is the data or backend tier of a web application. It runs on database management software, such as MySQL, Oracle, DB2, or PostgreSQL.
While three-tier architecture is easily the most widely adopted multitier application architecture, there are others that you might encounter in your work or your research.
Two-tier architecture
Two-tier architecture is the original client-server architecture, consisting of a presentation tier and a data tier; the business logic lives in the presentation tier, the data tier or both. In two-tier architecture the presentation tier - and therefore the end user - has direct access to the data tier, and the business logic is often limited. A simple contact management application, where users can enter and retrieve contact data, is an example of a two-tier application.
N-tier architecture
N-tier architecture - also called or multitier architecture - refers to any application architecture with more than one tier. But applications with more than three layers are rare because extra layers offer few benefits and can make the application slower, harder to manage and more expensive to run. As a result, n-tier architecture and multitier architecture are usually synonyms for three-tier architecture.
Move to cloud faster with IBM Cloud Pak solutions running on Red Hat OpenShift software—integrated, open, containerized solutions certified by IBM®.
Seamlessly modernize your VMware workloads and applications with IBM Cloud.
Modernize, build new apps, reduce costs, and maximize ROI.
IBM Consulting® application modernization services, which are powered by IBM Consulting Cloud Accelerator, offers skills, methods, tools, and initiatives that help determine the right strategy based on your portfolio. To modernize and containerize legacy system applications and accelerate the time-to-value of hybrid cloud environments.
Discover what application modernization is, the common benefits and challenges, and how to get started.
Learn about how relational databases work and how they compare to other data storage options.
Explore cloud native applications and how they drive innovation and speed within your enterprise.
Modernize your legacy three-tier applications on your journey to cloud. Whether you need assistance with strategy, processes, or capabilities—or want full-service attention—IBM can help. Start using containerized middleware that can run in any cloud—all bundled in IBM Cloud Paks.
Presentation Logic
- First Online: 05 September 2017
Cite this chapter
- Sten Vesterli 2
682 Accesses
ADF handles all the basic functionality of getting data from the database onto the web page, accepting changes, and storing data back. But when you want to implement your own special way of handling the user interface, you need to start writing code. This chapter describes how to add logic to the presentation layer, using prebuilt UI component validators (declarative), managed beans (server-side Java code), and custom client-side JavaScript code
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
Subscribe and save.
- Get 10 units per month
- Download Article/Chapter or eBook
- 1 Unit = 1 Article or 1 Chapter
- Cancel anytime
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
- Compact, lightweight edition
- Dispatched in 3 to 5 business days
- Free shipping worldwide - see info
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Author information
Authors and affiliations.
Værløse, Denmark
Sten Vesterli
You can also search for this author in PubMed Google Scholar
Rights and permissions
Reprints and permissions
Copyright information
© 2017 Sten Vesterli
About this chapter
Vesterli, S. (2017). Presentation Logic. In: Oracle ADF Survival Guide. Apress, Berkeley, CA. https://doi.org/10.1007/978-1-4842-2820-3_5
Download citation
DOI : https://doi.org/10.1007/978-1-4842-2820-3_5
Published : 05 September 2017
Publisher Name : Apress, Berkeley, CA
Print ISBN : 978-1-4842-2819-7
Online ISBN : 978-1-4842-2820-3
eBook Packages : Professional and Applied Computing Apress Access Books Professional and Applied Computing (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
Moments Log
Blogging every moment of your life

Breaking Down the Layers: Understanding the Four-tier Software Architecture
Table of Contents
Introduction to four-tier software architecture, benefits and advantages of four-tier software architecture, key components and layers in four-tier software architecture, best practices for implementing four-tier software architecture.
Unveiling the Essence: Decoding Four-tier Software Architecture
Software architecture is a crucial aspect of any software development project. It provides a blueprint for designing and organizing the various components of a software system. One popular approach to software architecture is the four-tier architecture, which divides the system into four distinct layers . In this article, we will delve into the world of four-tier software architecture, exploring its components and benefits.
At its core, four-tier software architecture is a client-server model that separates the user interface, business logic, data storage, and data access layers. Each layer has a specific role and interacts with the other layers in a well-defined manner. This separation of concerns allows for better scalability, maintainability, and flexibility in software development.
The first layer of the four-tier architecture is the presentation layer, also known as the user interface layer. This layer is responsible for presenting information to the user and receiving user input. It includes components such as web pages, forms, and graphical user interfaces. The presentation layer communicates with the business logic layer to retrieve and display data to the user.
Moving down the architecture, we come to the business logic layer. This layer contains the core functionality of the software system. It processes user requests, performs calculations, and enforces business rules. The business logic layer acts as the intermediary between the presentation layer and the data access layer, ensuring that data is retrieved and manipulated correctly.
Next, we have the data access layer. This layer is responsible for interacting with the database or any other data storage mechanism. It handles tasks such as retrieving, updating, and deleting data. The data access layer abstracts the underlying data storage technology, allowing the other layers to work with data in a consistent manner. It provides a level of separation between the business logic layer and the data storage layer, making it easier to switch databases or make changes to the data storage mechanism.
Finally, we reach the data storage layer, which is where the actual data is stored. This layer can consist of a relational database, a file system, or any other data storage technology. The data storage layer is responsible for ensuring data integrity, security, and availability. It is accessed by the data access layer to retrieve and store data.
Now that we have a basic understanding of the four-tier software architecture, let’s explore its benefits. One of the main advantages of this architecture is its modularity. Each layer can be developed and maintained independently, allowing for easier testing, debugging, and updates. Changes made to one layer do not necessarily affect the other layers, reducing the risk of unintended consequences.
Another benefit is scalability. The four-tier architecture allows for horizontal scaling, meaning that additional servers can be added to handle increased user load. This scalability is achieved by distributing the workload across multiple servers, with each server responsible for a specific layer. This distributed approach improves performance and ensures that the system can handle a growing number of users.
Additionally, the four-tier architecture promotes reusability. By separating the different layers, developers can reuse components across multiple projects. For example, the business logic layer can be reused in different user interfaces or with different data storage mechanisms. This reusability saves time and effort in software development, leading to faster delivery and reduced costs.
In conclusion, the four-tier software architecture provides a structured approach to software development, dividing the system into four distinct layers. Each layer has a specific role and interacts with the other layers in a well-defined manner. This architecture offers benefits such as modularity, scalability, and reusability. By understanding and implementing the four-tier architecture, software developers can create robust and flexible systems that meet the needs of their users.

One of the key advantages of four-tier software architecture is its ability to enhance scalability and flexibility. By separating the software into different layers, it becomes easier to modify or replace one layer without affecting the others. This modular approach allows for more efficient development and maintenance, as changes can be made to specific layers without disrupting the entire system. This flexibility is particularly valuable in large-scale software projects where multiple teams are working simultaneously on different components.
Another benefit of four-tier software architecture is improved performance and efficiency. By distributing the workload across multiple layers, the system can handle a higher volume of requests and process them more quickly. For example, the presentation layer, which is responsible for user interaction, can focus solely on rendering the user interface and handling user input, while the business logic layer handles the processing and manipulation of data. This division of labor ensures that each layer can perform its tasks efficiently, resulting in a more responsive and performant software system.
Furthermore, four-tier software architecture promotes reusability and maintainability. With clear separation between layers, developers can reuse components across different projects or even within the same project. This not only saves time and effort but also improves the overall quality of the software. Additionally, the modular nature of this architecture makes it easier to test and debug individual layers, simplifying the maintenance and troubleshooting process. This reduces the risk of introducing new bugs or issues when making changes to the software.
Security is another area where four-tier software architecture excels. By isolating sensitive data and logic in separate layers, it becomes easier to implement security measures at each level. For example, the data layer can enforce strict access controls and encryption mechanisms to protect the integrity and confidentiality of the data. Similarly, the business logic layer can implement authentication and authorization mechanisms to ensure that only authorized users can access certain functionalities. This layered approach to security minimizes the risk of unauthorized access or data breaches, making the software more robust and secure.
Lastly, four-tier software architecture promotes collaboration and teamwork. With clear boundaries between layers, different teams or individuals can work independently on their assigned layers, without stepping on each other’s toes. This promotes parallel development and allows for more efficient collaboration, as teams can focus on their specific areas of expertise. Moreover, the modular nature of this architecture facilitates integration with external systems or third-party components, enabling seamless collaboration with external stakeholders.
In conclusion, four-tier software architecture offers numerous benefits and advantages that make it a popular choice in the development of complex software systems. From enhanced scalability and flexibility to improved performance and efficiency, this architecture promotes reusability, maintainability, security, and collaboration. By understanding and leveraging the advantages of four-tier software architecture, developers can build robust, scalable, and maintainable software systems that meet the evolving needs of businesses and users alike.
In the world of software development, architects and developers often rely on different architectural patterns to design and build robust and scalable applications. One such pattern is the four-tier software architecture, which provides a structured approach to organizing the various components of a software system. Understanding the key components and layers in this architecture is crucial for developers looking to build efficient and maintainable applications.
At its core, the four-tier software architecture consists of four distinct layers: the presentation layer, the application layer, the business logic layer, and the data layer. Each layer has its own set of responsibilities and interacts with the layers above and below it in a well-defined manner.
The presentation layer, also known as the user interface layer, is the layer that users directly interact with. It is responsible for presenting information to the user and capturing user input. This layer typically includes components such as web pages, mobile app screens, and user input forms. Its primary goal is to provide a user-friendly interface that allows users to interact with the application easily.
Moving down the stack, we come to the application layer. This layer acts as a bridge between the presentation layer and the business logic layer. It handles user requests, processes them, and coordinates the flow of data between the presentation layer and the business logic layer. In simpler terms, it acts as a mediator, ensuring that the user’s actions are translated into meaningful operations that the business logic layer can understand.
The business logic layer, also known as the application layer, is where the core functionality of the software resides. It contains the business rules, algorithms, and workflows that define how the application operates. This layer is responsible for processing data, performing calculations, and enforcing business rules. It acts as the brain of the application, making decisions and orchestrating the overall behavior of the system.
Finally, we have the data layer, which is responsible for managing the storage and retrieval of data. This layer interacts with databases, file systems, or any other data storage mechanism used by the application. It handles tasks such as reading and writing data, querying databases, and ensuring data integrity. The data layer plays a critical role in ensuring that the application can store and retrieve data efficiently and reliably.
Understanding the interactions between these layers is crucial for building a well-structured and maintainable software system. Each layer has its own set of responsibilities, and they work together to create a cohesive and efficient application. For example, the presentation layer interacts with the application layer to handle user input and display information to the user. The application layer, in turn, interacts with the business logic layer to process user requests and enforce business rules. Finally, the business logic layer interacts with the data layer to store and retrieve data as needed.
By breaking down the software system into these distinct layers, developers can achieve a high degree of modularity and separation of concerns. Each layer can be developed and tested independently, making it easier to maintain and extend the application over time. Additionally, this architecture allows for scalability, as each layer can be scaled independently based on the specific needs of the application.
In conclusion, the four-tier software architecture provides a structured approach to organizing the components of a software system. Understanding the key components and layers in this architecture is crucial for developers looking to build efficient and maintainable applications. By breaking down the system into the presentation layer, application layer, business logic layer, and data layer, developers can achieve modularity, separation of concerns, and scalability. So, the next time you embark on a software development project, consider leveraging the power of the four-tier software architecture to build robust and scalable applications.
In today’s digital age, software architecture plays a crucial role in the development and implementation of complex applications. One popular approach to software architecture is the four-tier architecture, which provides a structured and scalable framework for building robust software systems. In this article, we will delve into the best practices for implementing four-tier software architecture, breaking down each layer and exploring its purpose and benefits.
The first layer of the four-tier architecture is the presentation layer. This layer is responsible for handling the user interface and interaction with the application. It focuses on providing a seamless and intuitive user experience, ensuring that the application is visually appealing and easy to navigate. By separating the presentation layer from the other layers, developers can make changes to the user interface without affecting the underlying business logic. This layer also enables the use of different presentation technologies, such as web or mobile, depending on the requirements of the application.
Moving on to the second layer, we have the application layer. This layer acts as the bridge between the presentation layer and the business logic layer. It handles the processing of user requests, validates input data, and coordinates the flow of information between the presentation and business logic layers. By encapsulating these responsibilities in a separate layer, developers can ensure that the application remains modular and maintainable. Additionally, the application layer enables the implementation of business rules and logic, making it easier to modify and extend the functionality of the application.
Next, we have the business logic layer, which forms the core of the application. This layer is responsible for implementing the business rules and processes that drive the application’s functionality. It handles complex calculations, data validation, and database operations. By separating the business logic from the other layers, developers can ensure that the application remains flexible and scalable. This layer also promotes code reusability, as the business logic can be shared across multiple applications or modules.
Finally, we have the data access layer, which is responsible for interacting with the underlying data storage systems, such as databases or external APIs. This layer handles the retrieval, storage, and manipulation of data, ensuring that the application has access to the necessary information. By separating the data access logic from the other layers, developers can easily switch between different data sources or make changes to the database schema without affecting the rest of the application. This layer also improves performance by optimizing data retrieval and minimizing network latency.
Implementing four-tier software architecture requires careful planning and adherence to best practices. One important aspect is the separation of concerns, where each layer focuses on a specific set of responsibilities. This separation enables modularity, maintainability, and scalability, making it easier to develop and evolve the application over time. Additionally, developers should strive for loose coupling between the layers, minimizing dependencies and promoting code reusability.
Another best practice is the use of well-defined interfaces between the layers. This allows for easy integration and testing, as each layer can be developed and tested independently. It also promotes interoperability, as different layers can be implemented using different technologies or programming languages. By adhering to these best practices, developers can ensure that the four-tier software architecture is implemented effectively and efficiently.
In conclusion, understanding the four-tier software architecture is essential for building robust and scalable applications. By breaking down each layer and exploring its purpose and benefits, developers can gain a deeper understanding of how the architecture works and how to implement it effectively. By following best practices such as separation of concerns and well-defined interfaces, developers can ensure that their applications are modular, maintainable, and scalable. So, whether you are a seasoned developer or just starting your journey in software architecture, the four-tier architecture is definitely worth exploring.
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
What's the difference between "Layers" and "Tiers"?
What's the difference between "Layers" and "Tiers"?
- architecture
- 7 Almost everyone I've met in software world uses these two terms interchangeably as if they are exactly the same. – RBT Commented Jun 3, 2016 at 9:08
- 2 "Layers refer to the inside of a cake, which can be anywhere between two and six layers of sponge, sandwiched together by buttercream before being decorated. Tiers refer to the number of cakes of increasing size that are stacked on top of each other." Yes, that's a real cooking definition, and pretty enlightening if you compare it to the selected answer: stackoverflow.com/a/120487/7389293 – carloswm85 Commented Oct 7, 2022 at 19:30
14 Answers 14
Logical layers are merely a way of organizing your code. Typical layers include Presentation, Business and Data – the same as the traditional 3-tier model. But when we’re talking about layers, we’re only talking about logical organization of code. In no way is it implied that these layers might run on different computers or in different processes on a single computer or even in a single process on a single computer. All we are doing is discussing a way of organizing a code into a set of layers defined by specific function. Physical tiers however, are only about where the code runs. Specifically, tiers are places where layers are deployed and where layers run. In other words, tiers are the physical deployment of layers.
Source: Rockford Lhotka, Should all apps be n-tier?
Read Scott Hanselman's post on the issue: A reminder on "Three/Multi Tier/Layer Architecture/Design" :
Remember though, that in "Scott World" (which is hopefully your world also :) ) a "Tier" is a unit of deployment, while a "Layer" is a logical separation of responsibility within code. You may say you have a "3-tier" system, but be running it on one laptop. You may say your have a "3-layer" system, but have only ASP.NET pages that talk to a database. There's power in precision, friends.
Layers refer to the logical separation of code. Logical layers help you organize your code better. For example, an application can have the following layers.
- Presentation Layer or UI Layer
- Business Layer or Business Logic Layer
- Data Access Layer or Data Layer
The above three layers reside in their own projects, maybe 3 projects or even more. When we compile the projects we get the respective layer DLL. So we have 3 DLLs now.
Depending upon how we deploy our application, we may have 1 to 3 tiers. As we now have 3 DLL's, if we deploy all the DLLs on the same machine, then we have only 1 physical tier but 3 logical layers.
If we choose to deploy each DLL on a separate machine, then we have 3 tiers and 3 layers.
So, Layers are a logical separation and Tiers are a physical separation. We can also say that tiers are the physical deployment of layers.
- What i understood from your answer that we can deploy 3 layers(DLL) on three different server. Right ? Can you please tell me how can i give reference of Business Logic layer on presentation layer ? – Mazhar Khan Commented Jan 9, 2013 at 7:22
- @MazharKhan You might want to use a service to expose the business layer functionality to the presentation layer – Amit Saxena Commented May 30, 2014 at 1:59
Why always trying to use complex words?
A layer = a part of your code , if your application is a cake, this is a slice.
A tier = a physical machine , a server.
A tier hosts one or more layers.
Example of layers:
- Presentation layer = usually all the code related to the User Interface
- Data Access layer = all the code related to your database access
Your code is hosted on a server = Your code is hosted on a tier.
Your code is hosted on 2 servers = Your code is hosted on 2 tiers.
For example, one machine hosting the Web Site itself (the Presentation layer), another machine more secured hosting all the more security sensitive code (real business code - business layer, database access layer, etc.).
There are so many benefits to implement a layered architecture. This is tricky and properly implementing a layered application takes time. If you have some, have a look at this post from Microsoft: http://msdn.microsoft.com/en-gb/library/ee658109.aspx
I've found a definition that says that Layers are a logical separation and tiers are a physical separation.
In plain english, the Tier refers to "each in a series of rows or levels of a structure placed one above the other" whereas the Layer refers to "a sheet, quantity, or thickness of material, typically one of several, covering a surface or body".
Tier is a physical unit , where the code / process runs. E.g.: client, application server, database server;
Layer is a logical unit , how to organize the code. E.g.: presentation (view), controller, models, repository, data access.
Tiers represent the physical separation of the presentation, business, services, and data functionality of your design across separate computers and systems.
Layers are the logical groupings of the software components that make up the application or service. They help to differentiate between the different kinds of tasks performed by the components, making it easier to create a design that supports reusability of components. Each logical layer contains a number of discrete component types grouped into sublayers, with each sublayer performing a specific type of task.
The two-tier pattern represents a client and a server.
In this scenario, the client and server may exist on the same machine, or may be located on two different machines. Figure below, illustrates a common Web application scenario where the client interacts with a Web server located in the client tier. This tier contains the presentation layer logic and any required business layer logic. The Web application communicates with a separate machine that hosts the database tier, which contains the data layer logic.

Advantages of Layers and Tiers:
Layering helps you to maximize maintainability of the code, optimize the way that the application works when deployed in different ways, and provide a clear delineation between locations where certain technology or design decisions must be made.
Placing your layers on separate physical tiers can help performance by distributing the load across multiple servers. It can also help with security by segregating more sensitive components and layers onto different networks or on the Internet versus an intranet.
A 1-Tier application could be a 3-Layer application.

Layers are logical separation of related-functional[code] within the application and Communication between the layers is explicit and loosely coupled. [ Presentation logic , Application logic , Data Access logic ]
Tiers are Physical separation of layers [which get hosted on Individual servers ] in an individual computer(process).

As shown in diagram:
n- Tier advantages: Better Security Scalability : As your organization grows You can scale up your DB-Tier with DB-Clustering with out touching other tiers. Maintainability : Web designer can change the View-code, with out touching the other layers on the other tiers. Easily Upgrade or Enhance [Ex: You can add Additional Application Code, Upgrade Storage Area, or even add Multiple presentation Layers for Separate devises like mobile, tablet, pc]

I like the below description from Microsoft Application Architecture Guide 2
Layers describe the logical groupings of the functionality and components in an application; whereas tiers describe the physical distribution of the functionality and components on separate servers, computers, networks, or remote locations. Although both layers and tiers use the same set of names (presentation, business, services, and data), remember that only tiers imply a physical separation.

Yes my dear friends said correctly. Layer is a logical partition of application whereas tier is physical partition of system tier partition is depends on layer partition. Just like an application execute on single machine but it follows 3 layered architecture, so we can say that layer architecture could be exist in a tier architecture. In simple term 3 layer architecture can implement in single machine then we can say that its is 1 tier architecture. If we implement each layer on separate machine then its called 3 tier architecture. A layer may also able to run several tier. In layer architecture related component to communicate to each other easily. Just like we follow given below architecture
- presentation layer
- business logic layer
- data access layer
A client could interact to "presentation layer", but they access public component of below layer's (like business logic layer's public component) to "business logic layer" due to security reason. Q * why we use layer architecture ? because if we implement layer architecture then we increase our applications efficiency like
==>security
==>manageability
==>scalability
other need like after developing application we need to change dbms or modify business logic etc. then it is necessary to all.
Q * why we use tier architecture?
because physically implementation of each layer gives a better efficiency ,without layer architecture we can not implement tier architecture. separate machine to implement separate tier and separate tier is implement one or more layer that's why we use it. it uses for the purposes of fault tolerance. ==>easy to maintain.
Simple example
Just like a bank open in a chamber, in which categories the employee:
- gate keeper
- a person for cash
- a person who is responsible to introduce banking scheme
they all are the related components of system.
If we going to bank for loan purpose then first a gate keeper open the door with smile after that we goes to near a person that introduce to all scheme of loan after that we goes to manager cabin and pass the loan. After that finally we goes to cashier's counter take loan. These are layer architecture of bank.
What about tier? A bank's branch open in a town, after that in another town, after that in another but what is the basic requirement of each branch
exactly the same concept of layer and tier.

- Great explanation dear – Dulaj Kulathunga Commented Jan 21, 2020 at 7:54
I use layers to describe the architect or technology stack within a component of my solutions. I use tiers to logically group those components typically when network or interprocess communication is involved.

Technically a Tier can be a kind of minimum environment required for the code to run.
E.g. hypothetically a 3-tier app can be running on
- 3 physical machines with no OS .
1 physical machine with 3 virtual machines with no OS.
(That was a 3-(hardware)tier app)
1 physical machine with 3 virtual machines with 3 different/same OSes
(That was a 3-(OS)tier app)
1 physical machine with 1 virtual machine with 1 OS but 3 AppServers
(That was a 3-(AppServer)tier app)
1 physical machine with 1 virtual machine with 1 OS with 1 AppServer but 3 DBMS
(That was a 3-(DBMS)tier app)
1 physical machine with 1 virtual machine with 1 OS with 1 AppServers and 1 DBMS but 3 Excel workbooks.
Excel workbook is the minimum required environment for VBA code to run.
Those 3 workbooks can sit on a single physical computer or multiple.
I have noticed that in practice people mean "OS Tier" when they say "Tier" in the app description context.
That is if an app runs on 3 separate OS then its a 3-Tier app.
So a pedantically correct way describing an app would be
"1-to-3-Tier capable, running on 2 Tiers" app.
Layers are just types of code in respect to the functional separation of duties withing the app (e.g. Presentation, Data , Security etc.)

When you talk about presentation, service, data, network layer, you are talking about layers. When you "deploy them separately", you talk about tiers.
Tiers is all about deployment. Take it this way: We have an application which has a frontend created in Angular, it has a backend as MongoDB and a middle layer which interacts between the frontend and the backend. So, when this frontend application, database application, and the middle layer is all deployed separately, we say it's a 3 tier application.
Benefit: If we need to scale our backend in the future, we only need to scale the backend independently and there's no need to scale up the frontend.
Layers are conceptual entities, and are used to separate the functionality of software system from a logical point of view; when you implement the system you organize these layers using different methods; in this condition we refer to them not as layers but as tiers.
IBM's Three-Tier Architecture article has a section dedicated to this topic:
In discussions of three-tier architecture, layer is often used interchangeably – and mistakenly – for tier, as in 'presentation layer' or 'business logic layer.' They aren't the same. A 'layer' refers to a functional division of the software, but a 'tier' refers to a functional division of the software that runs on infrastructure separate from the other divisions. The Contacts app on your phone, for example, is a three-layer application, but a single-tier application, because all three layers run on your phone. The difference is important, because layers can't offer the same benefits as tiers.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged architecture or ask your own question .
- The Overflow Blog
- Detecting errors in AI-generated code
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- Missing plot area in ContourPlot
- Enhancing my RSA implement in Python
- How can I have my paper reviewed?
- How can one be compensated for loss caused negligently by someone who dies in the process?
- Does AI use lots of water?
- Caculating Prices of Goods
- Would all disagreements vanish if everyone had access to the same information and followed the same reasoning process?
- Why is the "scan backwards" method of parsing right-associative operators considered to be anti-pattern? What alternatives are recommended?
- Using earphones as a dipole antenna
- how to make the latex support traditional-Chinese characters
- What purpose is the GND screw serving in this vanity lighting setup?
- Texture is not even on UV mapped material
- ぎおんまつり written with two different kanji for ぎ
- Why is thermal conductivity of thermal interface materials so low?
- Why does John 19:17 include the words, "which in Aramaic is called Golgotha"?
- Is ext4 and xfs only for usage with internal file systems?
- Did Microsoft actually release a “Critical Update Notification Tool”?
- Is Wild Shape affected by Moonbeam?
- How to implement a "scanner" effect on Linux to fix documents with varying darkness of background?
- How do cafes prepare matcha in a foodsafe way, if a bamboo whisk/chasen cannot be sanitized in a dishwasher?
- Can I still access the potato dimension?
- Do all languages distinguish between persons and non-persons?
- Could a civilisation develop spaceflight by artillery before developing orbit-capable rockets?
- Will running a dehumidifier in a basement impact room temperature?
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
What are the relations between the presentation/application/data logics, client and server, and MVC?
I appreciate if someone could clarify my confusions about the following quote from Distributed Systems , by Coulouris et al:
Consider the functional decomposition of a given application, as follows: • the presentation logic , which is concerned with handling user interaction and updating the view of the application as presented to the user; • the application logic , which is concerned with the detailed application-specific processing associated with the application (also referred to as the business logic); • the data logic , which is concerned with the persistent storage of the application, typically in a database management system. Consider the implementation of such an application using client-server technology and two-tier and three-tier solutions in Figure 2.8 (a) and (b). In the two-tier solution , the three aspects mentioned above must be partitioned into two processes, the client and the server. This is most commonly done by splitting the application logic , with some residing in the client and the remainder in the server (although other solutions are also possible). In the three-tier solution , there is a one-to-one mapping from logical elements to physical servers and hence, for example, the application logic is held in one place. Each tier also has a well-defined role; for example, the third tier is simply a database offering a (potentially standardized) relational service interface. The first tier can also be a simple user interface allowing intrinsic support for thin clients.
Do the figures specify the presentation/application/data logics? If so, how?
Do the figures specify the presentation logic exactly as "user view" and "control" (in both two tier and three tier cases)?
Do the figures specify the application logic exactly as "data manipulation" and "Application" (in the two tier case)?
Do the figures specify the data logic exactly as "data management" (in the two-tier case) and "database manager" (in the three-tier case)?
Do the figures specify the components of the MVC framework? If yes, how?
Do the figures specify "V" in MVC exactly as "user view" (in both two tier and three tier cases)?
Do the figures specify "C" in MVC exactly as "control" (in both two tier and three tier cases)?
Do the figures specify "M" in MVC exactly as "data manipulation" and "Application" (in the two tier case), and "data management" (in the two-tier case) and "database manager" (in the three-tier case)?
How do the presentation/application/data logics and MVC correspond to each other?
Does the presentation logic exactly consist of "V" and "C" in MVC?
Does "M" in MVC exactly consist of the application logic and the data logic?
- design-patterns
- web-applications

2 Answers 2
They don't specify them in any precise sense, but they do show a high level logical organization of the system. Presentation logic would be a part of the user-facing tiers (desktop GUIs, web pages, mobile device screens). Most of the application logic would be in what's on both pictures marked as "Tier 2".
"Tier" is a somewhat overloaded word, and people mean slightly different things by it, but these tiers may be just the logical organization of the source code, or how the code is packaged into libraries (e.g., DLLs), or they could represent different physical machines. My impression is that "tier" most often means "physical tier".
In real systems, things may not be organized so neatly. E.g., some of the application logic may need to be moved, or even duplicated on the user-facing tiers, to provide better user experience. Sometimes, parts of it may end up in the database tier, for various practical reasons (both good ones and bad ones).
Again, nothing is specified precisely here, but yes - presentation is concerned with displaying information and user interface, and this is what we call "Views". "Controls" here most likely refers to just the things that the user can do with the application, or it may even literally refer to GUI controls (buttons, textboxes, checkboxes, menus, things like that).
Roughly, yes. One way to think about this as application logic being separated by concerns of the two tiers (e.g. the fine-grained logic that can be done on, say, a phone, vs the more coarse-grained, resource-intensive logic that executes on some backend service).
I'll repeat the image here for easy reference:

The idea with having separate notions of presentation and application logic is that your application logic (the thing that your application actually does ) shouldn't depend on the way stuff is being shown to the user (the presentation logic), and on the intricacies of how exactly it's done. The image here is not really designed to show that; it's more focused on the high level organizational view of the system.
Yes; it just means that there's some kind of a database that stores the data and provides various capabilities that let you query it, etc. But, depending on the application, it could be something as simple as a file, and some code that governs how you read and write to it.
No. There's no notion of MVC in these pictures.
If MVC was shown here, the V would roughly correspond to "Views and Controls"
No. Here "controls" most likely just refers to either the on-screen widgets (buttons and such), or just the things that the user can do (in the same sense you'd use the word "controls" in a video game). In MVC, C stands for "Controller", which is a component that sort of coordinates the interaction between the Views and the Model (see below).
Not quite. The M in MVC is not about data storage. MVC is a GUI pattern, so it's concerned with the GUI and the presentation logic. In MVC, you can think of the Model as of a black box of sorts that encompass "the rest of the application"; from the presentation-related point of view, we don't really care (and we want to be isolated) from the internals of the rest of the application - we just want to know how to interact with it. A more useful way to think about it, though, is that the Model corresponds, on a very conceptual level, to the application logic, or to a part of the application logic that's relevant for this particular view; in contrast, the layered/tiered view is a high-level overview of the application as a whole.
That said, there's a lot of history and a lot of terminology here, and there are different variants of MVC itself, and related patterns like MVP, MVVM, etc. You'll encounter terms like "ViewModel" and "Presentation Model" - this is not the same as (or related to) Model (if anything, they are associated with the Controller, or Presenter in MVP). Then there's Web MVC where Views correspond to web pages (as in, HTML/CSS/JS source), or templates that generate such pages, rather then desktop widgets. There too you may encounter "view models", but these are often just data bags that serve as a way to pass data to the view, so again, it's a different notion.
Also, the original MVC (when first invented/described) was different than how we think of it today - back then, every little widget had it's own trio of MVC components (as in, every button would have its own View that could draw it, a Controller that knew how to deal with user input, and a little piece of application logic that was represented by its Model). That's not how we use the pattern today; typically, a View would be an entire screen, or maybe a panel within that screen.
Since MVC is a GUI pattern (some might call it GUI architecture, but it doesn't really matter), most of it is about the presentation layer, and the organization within it.
I'd say that's roughly correct; at least, V & C are concerned with the presentation logic (but as C also interacts with the Model, one might say that it handles additional concerns as well).

MVC (model-view-controller) is an architectural pattern, but it does not map directly into N-tier architecture: Those are more or less orthogonal, and in principle each layer can have their own (even multiple) MVCs. And of course N-tier architecture can use MVC somewhere or not.
For some simplified system, maybe one can somehow put model at some business domain layer, between application and persistence layers. But View and Controller will necessarily be in representation and application layers both. But I think this is a misleading: MVC and 2- or 3-tier are not exactly comparable, and actually Layers is an architectural pattern on it's own.
Application layer and business domain layer usually (but not necessarily) are at application tier, as well as persistence layer, which connects to the "Data(base) management" "tier".
As for multiple MVCs, one can imaging having it's very own MVC in the representation layer (for example, in-webbrowser frontend can be built with MVC pattern), in application layer (view, controller) + business domain (model). With some technologies it's even possible to span MVC across those three layers. Persistence layer can have it's own MVCs. And if we go into data management - it can also have it's very own MVCs.
That is, even with multi-tier architecture we can have logical layers and MVCs inside or across.
- Thanks. (1) "in principle each layer can have their own (even multiple)", do you mean "tier" by "layer"? (2) Could you elaborate how "each layer can have their own (even multiple)"? (3) Are "business domain layer, intermediating application and persistence layers" and "representation and application layers" synonyms for presentation, application (i.e. business) and data logics in the book mentioned in my post? – Tim Commented Jun 5, 2019 at 17:53
- (4) " View and Controller will necessarily be in representation and application layers both", do you mean "view" must be in both "representation and application layers", and "controller" must also be in both "representation and application layers" – Tim Commented Jun 5, 2019 at 18:24
- My answer in short: MVC relation to "tiers" or "layers" is in general arbitrary. It's basically a loop, which can be found inside or across tiers and layers. Here by tiers I understand software units, which can reside on different physical machines. And by layers - abstraction layers of the whole stack. – Roman Susi Commented Jun 5, 2019 at 18:48
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Software Engineering Stack Exchange. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged design-patterns web-applications mvc n-tier or ask your own question .
- The Overflow Blog
- Detecting errors in AI-generated code
- Featured on Meta
- User activation: Learnings and opportunities
- Preventing unauthorized automated access to the network
Hot Network Questions
- Replacing "shall not", "shall be", "shall" etc. with "must" or other more imperative words
- The 12th Amendment: what if the presidential and vice-presidential candidates are from the same state?
- Why doesn't reductio ad absurdum mean everything follows?
- How uncommon/problematic is a passport whose validity period (period between issue and expiry) is a non-whole number of years?
- How fast to play Rachmaninoff’s Études-Tableaux, Op. 33 No. 5 in G minor?
- Does rebuilding a clustered index offline require extra space for each non-clustered index?
- Could a civilisation develop spaceflight by artillery before developing orbit-capable rockets?
- Can I increase the wattage of my landcruiser plug?
- Texture is not even on UV mapped material
- Adjusting water quanity according to whether I soak rice before cooking
- Middle path of buddha
- SF story set in an isolated (extragalactic) star system
- Explorifying `\latex2e` code
- How do gravitational waves cause photons to be polarized?
- Expecting ad hominem criticism in a thesis defense: How to prepare well for this?
- What is the book/author about preserved heads/brains?
- Can artistic depictions of crime (especially violence) be used as evidence?
- He worked in the field during most of the day
- Caculating Prices of Goods
- Which tool has been used to make this puzzle?
- Using earphones as a dipole antenna
- How can one be compensated for loss caused negligently by someone who dies in the process?
- "immer noch" meaning "still"
- Pnemautic lift cabinet door won't stay open
Separated Presentation
Ensure that any code that manipulates presentation only manipulates presentation, pushing all domain and data source logic into clearly separated areas of the program.
29 June 2006
This is part of the Further Enterprise Application Architecture development writing that I was doing in the mid 2000’s. Sadly too many other things have claimed my attention since, so I haven’t had time to work on them further, nor do I see much time in the foreseeable future. As such this material is very much in draft form and I won’t be doing any corrections or updates until I’m able to find time to work on it again.
How it Works
This pattern is a form of layering, where we keep presentation code and domain code in separate layers with the domain code unaware of presentation code. This style came into vogue with Model-View-Controller architecture and is widely used.
To use it you begin by looking at all the data and behavior in a system and looking to see if that code is involved with the presentation. Presentation code would manipulate GUI widgets and structures in a rich client application, HTTP headers and HTML in a web application, or command line arguments and print statements in a command line application. We then divide the application into two logical modules with all the presentation code in one module and the rest in another module.
Further layering is often used to separate data source code from domain (business logic), and to separate the the domain using a Service Layer . For the purposes of Separated Presentation we can ignore these further layers, just referring to all of this as 'the domain layer'. Just bear in mind that further layering of the domain layer is likely.
The layers are a logical and not a physical construct. Certainly you may find a physical separation into different tiers but this is not required (and a bad idea if not necessary). You also may see a separation into different physical packaging units (eg Java jars or .NET assemblies), but again this isn't required. Certainly it is good to use any logical packaging mechanisms (Java packages, .NET namespaces) to separate the layers.
As well as a separation, there is also a strict visibility rule. The presentation is able to call the domain but not vice-versa. This can be checked as part of a build with dependency checking tools. The point here is that the domain should be utterly unaware of what presentations may be used with it. This both helps keep the concers separate and also supports using multiple presentations with the same domain code.
Although the domain cannot call the presentation it's often necessary for the domain to notify the presentation if any changes occur. Observer is the usual solution to this problem. The domain fires an event which is observed the by presentation, the presentation then re-reads data from the domain as needed.
A good mental test to use to check you are using Separated Presentation is to imagine a completely different user interface. If you are writing a GUI imagine writing a command line interface for the same application. Ask yourself if anything would be duplicated between the GUI and command line presentation code - if it is then it's a good candidate for moving to the domain.
When to Use It
Example: moving domain logic out of a window (java).
Most of examples you'll see from me follow Separated Presentation , simply because I find it such a fundamental design technique. Here's an example of how you can refactor a simple design that doesn't use Separated Presentation in order to use it.
The example comes from the running example of Ice Cream Atmospheric Monitor. The main task I use to illustrate this is calculating the variance between target and actual and coloring the field to indicate the amount of this variance. You can imagine this done in the assessment window object like this:
class AssessmentWindow...
As you can see this routine mixes the domain problem of calculating the variance with the behavior of updating the the variance text field. The Reading object, that holds the actual and target data, is here a data class - an anemic collection of fields and accessors. Since this is the object that has the data, it should be the one to calculate the variance.
To start this I can use Replace Temp with Query on the variance calculation itself, to yield this.
With the calculation now in its own method, I can safely move it to the Reading object.
I can do the same thing with the varianceRatio, I'll just show the final result but again I do it to steps (creating the local method then moving it) as I'm less likely to mess it up that way - particularly with the refactoring editor (IntelliJ Idea) I'm using.
The calculations are looking better, but I'm still not too happy. The logic about when the color should be one color or another is domain logic, even though the choice of color (and the fact that text color is the presentation mechanism) is presentation logic. What I need to do is split the out the determination of which category of variance we have (and the logic for assigning these categories) from the coloring.
There's no formalized refactoring here, but what I need is a method like this on Reading.
That's better, I now have the domain decisions in the domain object. But things are a bit messy, the presentation shouldn't have to know that the variance is null if the actual reading is null. That kind of dependency should be encapsulated in the Reading class.
I can further encapsultate this by adding a null variance category, which also allows me to use a switch that I find easier to read.
As a last step, although not connected to Separated Presentation , I prefer to clean that switch up to remove the duplication.
This makes it obvious that what we have here is a simple table lookup. I could replace this by populating and indexing out of a hash. In some languages I might do that, but I find the switch here to be nice and readable in Java.
- Engineering Mathematics
- Discrete Mathematics
- Operating System
- Computer Networks
- Digital Logic and Design
- C Programming
- Data Structures
- Theory of Computation
- Compiler Design
- Computer Org and Architecture
Business-Logic Layer
In this article, we are going to learn about the Business Logic Layer in Database Management systems. The Business Logic Layer also known as BLL act as an intermediate between the Presentation Layer and the Data Access Layer (DAL). This layer handles the business logic, business rules as well as calculations. It tells how the data from the database can be used, what it can perform, and what can not within its application.
The Business-Logic Layer (BLL) is a component of a software architecture that is responsible for implementing the business logic of an application. It sits between the presentation layer (e.g., the user interface) and the data access layer (e.g., the database), and is responsible for processing and manipulating data before it is presented to the user or stored in the database.
The BLL is responsible for performing tasks such as: -Validating input data to ensure that it meets the necessary business rules and constraints. -Performing calculations and transformations on data, as required by the business logic. -Enforcing business rules and policies, such as access control and security. -Communicating with the data access layer to retrieve and store data. -Handling errors and exceptions.
The BLL is designed to be reusable and independent of the user interface and data storage implementation. This allows the application to be easily modified or extended without affecting the underlying business logic.
The BLL is also responsible for managing the workflows and the use cases of the application, by handling the communication between the different layers, and by implementing the rules and constraints of the business.
In summary, The Business-Logic Layer (BLL) is a component of a software architecture that is responsible for implementing the business logic of an application. It sits between the presentation layer and the data access layer, and is responsible for processing and manipulating data before it is presented to the user or stored in the database. It also manages the workflows and the use cases of the application, and it is designed to be reusable and independent of the user interface and data storage implementation.
- Presentation Layer: The layer at which users interact with the application and the final data will be visible to the users at this interface. It acts as an interface between the user and the application.
- Business Logic Layer: It acts as an intermediate between the Presentation and the Data Access Layer.
- Data Access Layer: The layer at which the data is managed.
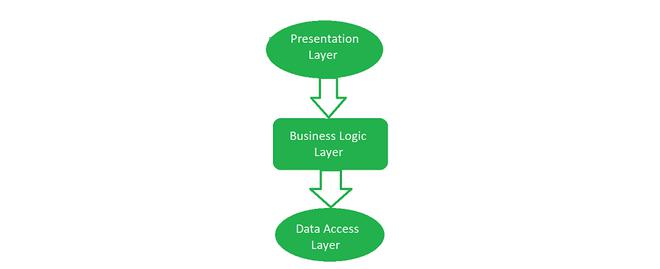
Business Logic Layer
- All the three layers above play an important role in building an application
- The business logic layer manages the communication between the database and the presentation layer.
Example: In an application, when the user access it or write queries in it with the help of a presentation or user interface layer the business logic layer helps the user to get a response to the asked queries by transferring it to the Data Access layer which further processes the query and give the suitable result to a business logic layer which is further transferred to the presentation layer which makes it visible to the user.
Due to the less clarity in defining the Business logic layer, some business domains like Microsoft and Apple excluded the BLL from their applications which leads to difficulty in code maintenance. A better approach is to build an application that supports multiple different user Interfaces.
Advantages of Business Logic Layer:
- Code Maintenance is easy: Maintaining the code will be easy when we are using the Business Logical Layer as it supports multitier architecture. By using this we can easily determine any kind of change in the code.
- Security: This architecture provides us the security as we can see that the Presentation layer doesn’t directly interact with the Data Access Layer which prevents it from any kind of data loss and ensures that the data is secure at the Data layer.
- Application releases: It makes application release rollout easy. Because the Business Logic Layer is the only one to be updated every time then we do not need other layers of architecture i.e. the Presentation layer and the Data Access Layer.
- Ease of learning: It is easy to learn because the learner has to specialize only in the presentation, data, and business layer to more quickly learn the specific parts of the application. The development time taken by the application will be small as all the layers can work together at the same time.
Disadvantages of Business Logic Layer:
- Expensive: It will be very difficult and expensive to install and maintain this layer in databases.
- Source control is very difficult to perform properly with existing procedures.
- It makes it difficult to use the code, again and again, such that it decreases code reusability .
Applications of Business Logic Layer:
- BLL has a major application in building multi-tier applications.
- It is most commonly used in creating component-based applications.
Please Login to comment...
Similar reads.
- Computer Subject
- How to Underline in Discord
- How to Block Someone on Discord
- How to Report Someone on Discord
- How to add Bots to Discord Servers
- 15 Most Important Aptitude Topics For Placements [2024]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Creating a Business Logic Layer (C#)
- 9 contributors
by Scott Mitchell
Download PDF
In this tutorial we'll see how to centralize your business rules into a Business Logic Layer (BLL) that serves as an intermediary for data exchange between the presentation layer and the DAL.
Introduction
The Data Access Layer (DAL) created in the first tutorial cleanly separates the data access logic from the presentation logic. However, while the DAL cleanly separates the data access details from the presentation layer, it does not enforce any business rules that may apply. For example, for our application we may want to disallow the CategoryID or SupplierID fields of the Products table to be modified when the Discontinued field is set to 1, or we might want to enforce seniority rules, prohibiting situations in which an employee is managed by someone who was hired after them. Another common scenario is authorization perhaps only users in a particular role can delete products or can change the UnitPrice value.
In this tutorial we'll see how to centralize these business rules into a Business Logic Layer (BLL) that serves as an intermediary for data exchange between the presentation layer and the DAL. In a real-world application, the BLL should be implemented as a separate Class Library project; however, for these tutorials we'll implement the BLL as a series of classes in our App_Code folder in order to simplify the project structure. Figure 1 illustrates the architectural relationships among the presentation layer, BLL, and DAL.

Figure 1 : The BLL Separates the Presentation Layer from the Data Access Layer and Imposes Business Rules
Step 1: Creating the BLL Classes
Our BLL will be composed of four classes, one for each TableAdapter in the DAL; each of these BLL classes will have methods for retrieving, inserting, updating, and deleting from the respective TableAdapter in the DAL, applying the appropriate business rules.
To more cleanly separate the DAL- and BLL-related classes, let's create two subfolders in the App_Code folder, DAL and BLL . Simply right-click on the App_Code folder in the Solution Explorer and choose New Folder. After creating these two folders, move the Typed DataSet created in the first tutorial into the DAL subfolder.
Next, create the four BLL class files in the BLL subfolder. To accomplish this, right-click on the BLL subfolder, choose Add a New Item, and choose the Class template. Name the four classes ProductsBLL , CategoriesBLL , SuppliersBLL , and EmployeesBLL .

Figure 2 : Add Four New Classes to the App_Code Folder
Next, let's add methods to each of the classes to simply wrap the methods defined for the TableAdapters from the first tutorial. For now, these methods will just call directly into the DAL; we'll return later to add any needed business logic.
If you are using Visual Studio Standard Edition or above (that is, you're not using Visual Web Developer), you can optionally design your classes visually using the Class Designer . Refer to the Class Designer Blog for more information on this new feature in Visual Studio.
For the ProductsBLL class we need to add a total of seven methods:
- GetProducts() returns all products
- GetProductByProductID(productID) returns the product with the specified product ID
- GetProductsByCategoryID(categoryID) returns all products from the specified category
- GetProductsBySupplier(supplierID) returns all products from the specified supplier
- AddProduct(productName, supplierID, categoryID, quantityPerUnit, unitPrice, unitsInStock, unitsOnOrder, reorderLevel, discontinued) inserts a new product into the database using the values passed-in; returns the ProductID value of the newly inserted record
- UpdateProduct(productName, supplierID, categoryID, quantityPerUnit, unitPrice, unitsInStock, unitsOnOrder, reorderLevel, discontinued, productID) updates an existing product in the database using the passed-in values; returns true if precisely one row was updated, false otherwise
- DeleteProduct(productID) deletes the specified product from the database
ProductsBLL.cs
The methods that simply return data GetProducts , GetProductByProductID , GetProductsByCategoryID , and GetProductBySuppliersID are fairly straightforward as they simply call down into the DAL. While in some scenarios there may be business rules that need to be implemented at this level (such as authorization rules based on the currently logged on user or the role to which the user belongs), we'll simply leave these methods as-is. For these methods, then, the BLL serves merely as a proxy through which the presentation layer accesses the underlying data from the Data Access Layer.
The AddProduct and UpdateProduct methods both take in as parameters the values for the various product fields and add a new product or update an existing one, respectively. Since many of the Product table's columns can accept NULL values ( CategoryID , SupplierID , and UnitPrice , to name a few), those input parameters for AddProduct and UpdateProduct that map to such columns use nullable types . Nullable types are new to .NET 2.0 and provide a technique for indicating whether a value type should, instead, be null . In C# you can flag a value type as a nullable type by adding ? after the type (like int? x; ). Refer to the Nullable Types section in the C# Programming Guide for more information.
All three methods return a Boolean value indicating whether a row was inserted, updated, or deleted since the operation may not result in an affected row. For example, if the page developer calls DeleteProduct passing in a ProductID for a non-existent product, the DELETE statement issued to the database will have no affect and therefore the DeleteProduct method will return false .
Note that when adding a new product or updating an existing one we take in the new or modified product's field values as a list of scalars as opposed to accepting a ProductsRow instance. This approach was chosen because the ProductsRow class derives from the ADO.NET DataRow class, which doesn't have a default parameterless constructor. In order to create a new ProductsRow instance, we must first create a ProductsDataTable instance and then invoke its NewProductRow() method (which we do in AddProduct ). This shortcoming rears its head when we turn to inserting and updating products using the ObjectDataSource. In short, the ObjectDataSource will try to create an instance of the input parameters. If the BLL method expects a ProductsRow instance, the ObjectDataSource will try to create one, but fail due to the lack of a default parameterless constructor. For more information on this problem, refer to the following two ASP.NET Forums posts: Updating ObjectDataSources with Strongly-Typed DataSets , and Problem With ObjectDataSource and Strongly-Typed DataSet .
Next, in both AddProduct and UpdateProduct , the code creates a ProductsRow instance and populates it with the values just passed in. When assigning values to DataColumns of a DataRow various field-level validation checks can occur. Therefore, manually putting the passed in values back into a DataRow helps ensure the validity of the data being passed to the BLL method. Unfortunately the strongly-typed DataRow classes generated by Visual Studio do not use nullable types. Rather, to indicate that a particular DataColumn in a DataRow should correspond to a NULL database value we must use the SetColumnNameNull() method.
In UpdateProduct we first load in the product to update using GetProductByProductID(productID) . While this may seem like an unnecessary trip to the database, this extra trip will prove worthwhile in future tutorials that explore optimistic concurrency. Optimistic concurrency is a technique to ensure that two users who are simultaneously working on the same data don't accidentally overwrite one another's changes. Grabbing the entire record also makes it easier to create update methods in the BLL that only modify a subset of the DataRow's columns. When we explore the SuppliersBLL class we'll see such an example.
Finally, note that the ProductsBLL class has the DataObject attribute applied to it (the [System.ComponentModel.DataObject] syntax right before the class statement near the top of the file) and the methods have DataObjectMethodAttribute attributes . The DataObject attribute marks the class as being an object suitable for binding to an ObjectDataSource control , whereas the DataObjectMethodAttribute indicates the purpose of the method. As we'll see in future tutorials, ASP.NET 2.0's ObjectDataSource makes it easy to declaratively access data from a class. To help filter the list of possible classes to bind to in the ObjectDataSource's wizard, by default only those classes marked as DataObjects are shown in the wizard's drop-down list. The ProductsBLL class will work just as well without these attributes, but adding them makes it easier to work with in the ObjectDataSource's wizard.
Adding the Other Classes
With the ProductsBLL class complete, we still need to add the classes for working with categories, suppliers, and employees. Take a moment to create the following classes and methods using the concepts from the example above:
CategoriesBLL.cs
- GetCategories()
- GetCategoryByCategoryID(categoryID)
SuppliersBLL.cs
- GetSuppliers()
- GetSupplierBySupplierID(supplierID)
- GetSuppliersByCountry(country)
- UpdateSupplierAddress(supplierID, address, city, country)
EmployeesBLL.cs
- GetEmployees()
- GetEmployeeByEmployeeID(employeeID)
- GetEmployeesByManager(managerID)
The one method worth noting is the SuppliersBLL class's UpdateSupplierAddress method. This method provides an interface for updating just the supplier's address information. Internally, this method reads in the SupplierDataRow object for the specified supplierID (using GetSupplierBySupplierID ), sets its address-related properties, and then calls down into the SupplierDataTable 's Update method. The UpdateSupplierAddress method follows:
Refer to this article's download for my complete implementation of the BLL classes.
Step 2: Accessing the Typed DataSets Through the BLL Classes
In the first tutorial we saw examples of working directly with the Typed DataSet programmatically, but with the addition of our BLL classes, the presentation tier should work against the BLL instead. In the AllProducts.aspx example from the first tutorial, the ProductsTableAdapter was used to bind the list of products to a GridView, as shown in the following code:
To use the new BLL classes, all that needs to be changed is the first line of code simply replace the ProductsTableAdapter object with a ProductBLL object:
The BLL classes can also be accessed declaratively (as can the Typed DataSet) by using the ObjectDataSource. We'll be discussing the ObjectDataSource in greater detail in the following tutorials.

Figure 3 : The List of Products is Displayed in a GridView ( Click to view full-size image )
Step 3: Adding Field-Level Validation to the DataRow Classes
Field-level validation are checks that pertains to the property values of the business objects when inserting or updating. Some field-level validation rules for products include:
- The ProductName field must be 40 characters or less in length
- The QuantityPerUnit field must be 20 characters or less in length
- The ProductID , ProductName , and Discontinued fields are required, but all other fields are optional
- The UnitPrice , UnitsInStock , UnitsOnOrder , and ReorderLevel fields must be greater than or equal to zero
These rules can and should be expressed at the database level. The character limit on the ProductName and QuantityPerUnit fields are captured by the data types of those columns in the Products table ( nvarchar(40) and nvarchar(20) , respectively). Whether fields are required and optional are expressed by if the database table column allows NULL s. Four check constraints exist that ensure that only values greater than or equal to zero can make it into the UnitPrice , UnitsInStock , UnitsOnOrder , or ReorderLevel columns.
In addition to enforcing these rules at the database they should also be enforced at the DataSet level. In fact, the field length and whether a value is required or optional are already captured for each DataTable's set of DataColumns. To see the existing field-level validation automatically provided, go to the DataSet Designer, select a field from one of the DataTables and then go to the Properties window. As Figure 4 shows, the QuantityPerUnit DataColumn in the ProductsDataTable has a maximum length of 20 characters and does allow NULL values. If we attempt to set the ProductsDataRow 's QuantityPerUnit property to a string value longer than 20 characters an ArgumentException will be thrown.

Figure 4 : The DataColumn Provides Basic Field-Level Validation ( Click to view full-size image )
Unfortunately, we can't specify bounds checks, such as the UnitPrice value must be greater than or equal to zero, through the Properties window. In order to provide this type of field-level validation we need to create an event handler for the DataTable's ColumnChanging event. As mentioned in the preceding tutorial , the DataSet, DataTables, and DataRow objects created by the Typed DataSet can be extended through the use of partial classes. Using this technique we can create a ColumnChanging event handler for the ProductsDataTable class. Start by creating a class in the App_Code folder named ProductsDataTable.ColumnChanging.cs .

Figure 5 : Add a New Class to the App_Code Folder ( Click to view full-size image )
Next, create an event handler for the ColumnChanging event that ensures that the UnitPrice , UnitsInStock , UnitsOnOrder , and ReorderLevel column values (if not NULL ) are greater than or equal to zero. If any such column is out of range, throw an ArgumentException .
ProductsDataTable.ColumnChanging.cs
Step 4: Adding Custom Business Rules to the BLL's Classes
In addition to field-level validation, there may be high-level custom business rules that involve different entities or concepts not expressible at the single column level, such as:
- If a product is discontinued, its UnitPrice cannot be updated
- An employee's country of residence must be the same as their manager's country of residence
- A product cannot be discontinued if it is the only product provided by the supplier
The BLL classes should contain checks to ensure adherence to the application's business rules. These checks can be added directly to the methods to which they apply.
Imagine that our business rules dictate that a product could not be marked discontinued if it was the only product from a given supplier. That is, if product X was the only product we purchased from supplier Y , we could not mark X as discontinued; if, however, supplier Y supplied us with three products, A , B , and C , then we could mark any and all of these as discontinued. An odd business rule, but business rules and common sense aren't always aligned!
To enforce this business rule in the UpdateProducts method we'd start by checking if Discontinued was set to true and, if so, we'd call GetProductsBySupplierID to determine how many products we purchased from this product's supplier. If only one product is purchased from this supplier, we throw an ApplicationException .
Responding to Validation Errors in the Presentation Tier
When calling the BLL from the presentation tier we can decide whether to attempt to handle any exceptions that might be raised or let them bubble up to ASP.NET (which will raise the HttpApplication 's Error event). To handle an exception when working with the BLL programmatically, we can use a try...catch block, as the following example shows:
As we'll see in future tutorials, handling exceptions that bubble up from the BLL when using a data Web control for inserting, updating, or deleting data can be handled directly in an event handler as opposed to having to wrap code in try...catch blocks.
A well architected application is crafted into distinct layers, each of which encapsulates a particular role. In the first tutorial of this article series we created a Data Access Layer using Typed DataSets; in this tutorial we built a Business Logic Layer as a series of classes in our application's App_Code folder that call down into our DAL. The BLL implements the field-level and business-level logic for our application. In addition to creating a separate BLL, as we did in this tutorial, another option is to extend the TableAdapters' methods through the use of partial classes. However, using this technique does not allow us to override existing methods nor does it separate our DAL and our BLL as cleanly as the approach we've taken in this article.
With the DAL and BLL complete, we're ready to start on our presentation layer. In the next tutorial we'll take a brief detour from data access topics and define a consistent page layout for use throughout the tutorials.
Happy Programming!
About the Author
Scott Mitchell, author of seven ASP/ASP.NET books and founder of 4GuysFromRolla.com , has been working with Microsoft Web technologies since 1998. Scott works as an independent consultant, trainer, and writer. His latest book is Sams Teach Yourself ASP.NET 2.0 in 24 Hours . He can be reached at [email protected]. or via his blog, which can be found at http://ScottOnWriting.NET .
Special Thanks To
This tutorial series was reviewed by many helpful reviewers. Lead reviewers for this tutorial were Liz Shulok, Dennis Patterson, Carlos Santos, and Hilton Giesenow. Interested in reviewing my upcoming MSDN articles? If so, drop me a line at [email protected].
Previous Next
Was this page helpful?
Additional resources

IMAGES
VIDEO
COMMENTS
Presentation logic. In software development, presentation logic is concerned with how business objects are displayed to users of the software, e.g. the choice between a pop-up screen and a drop-down menu. [1] The separation of business logic from presentation logic is an important concern for software development and an instance of the ...
Prerequisite : OSI Model. Introduction : Presentation Layer is the 6th layer in the Open System Interconnection (OSI) model. This layer is also known as Translation layer, as this layer serves as a data translator for the network. The data which this layer receives from the Application Layer is extracted and manipulated here as per the required ...
For example, components in the presentation layer deal only with presentation logic, whereas components residing in the business layer deal only with business logic. This type of component classification makes it easy to build effective roles and responsibility models into your architecture, and also makes it easy to develop, test, govern, and ...
The important part is that the user never directly interacts with the logic or data tiers. All user interactions with the application occur through the presentation tier. The same goes for each adjacent layer in the 3-tier application. For example, the presentation layer communicates with the logic layer but never directly with the data layer.
Presentation logic is the core of the application. The business logic layer provides a foundation for the user to interact with the application. It also contains all the business rules for the server. By contrast, presentation logic is the underlying code that determines how the data is presented. In contrast to business rules, presentation ...
The two main distinctions enterprise application architecture has from a regular web application is the addition of another layer to the classic pattern - the service layer. The service layer is another abstraction between Presentation and Business Logic. It's an integration gateway that allows other software to access your business logic and ...
In a logical multilayer architecture for an information system with an object-oriented design, the following four are the most common: . Presentation layer (a.k.a. UI layer, view layer, presentation tier in multitier architecture); Application layer (a.k.a. service layer [8] [9] or GRASP Controller Layer [10]); Business layer (a.k.a. business logic layer (BLL), domain logic layer)
web development. One of the most common ways to modularize an information-rich program is to separate it into three broad layers: presentation (UI), domain logic (aka business logic), and data access. So you often see web applications divided into a web layer that knows about handling HTTP requests and rendering HTML, a business logic layer ...
One of the most useful things you can do when designing any presentation layer is to enforce Separated Presentation. Once you've done this, the next step is to think about how the presentation logic itself is going to be organized. For a simple window, a single class may well suffice. But more complex logic leads to a wider range of breakdowns.
Overview. The presentation layer contains the components that implement and display the user interface and manage user interaction. This layer includes controls for user input and display, in addition to components that organize user interaction. Figure 1 shows how the presentation layer fits into a common application architecture.
2. Business Logic Layer. The business logic layer, also known as the "API middleware," is the layer that contains the core logic of the API. It is responsible for processing the requests received from the presentation layer and generating responses based on the requested actions. The business logic layer is where the API's core functionality ...
The presentation tier and the data tier cannot communicate directly with one another. Tier versus layer. In discussions of three-tier architecture, layer is often used interchangeably - and mistakenly - for tier, as in 'presentation layer' or 'business logic layer'. They aren't the same.
This chapter describes how to add logic to the presentation layer, using prebuilt UI component validators (declarative), managed beans (server-side Java code), and custom client-side JavaScript code. Download chapter PDF. As you saw in Chapter 1, ADF handles all the basic functionality of getting data from the database onto the web page ...
This layer is responsible for presenting information to the user and receiving user input. It includes components such as web pages, forms, and graphical user interfaces. The presentation layer communicates with the business logic layer to retrieve and display data to the user. Moving down the architecture, we come to the business logic layer.
A layer = a part of your code, if your application is a cake, this is a slice. A tier = a physical machine, a server. A tier hosts one or more layers. Example of layers: Presentation layer = usually all the code related to the User Interface. Data Access layer = all the code related to your database access.
Presentation logic would be a part of the user-facing tiers (desktop GUIs, web pages, mobile device screens). Most of the application logic would be in what's on both pictures marked as "Tier 2". ... Since MVC is a GUI pattern (some might call it GUI architecture, but it doesn't really matter), most of it is about the presentation layer, and ...
Further layering is often used to separate data source code from domain (business logic), and to separate the the domain using a Service Layer. For the purposes of Separated Presentation we can ignore these further layers, just referring to all of this as 'the domain layer'. Just bear in mind that further layering of the domain layer is likely.
The Business-Logic Layer (BLL) is a component of a software architecture that is responsible for implementing the business logic of an application. It sits between the presentation layer (e.g., the user interface) and the data access layer (e.g., the database), and is responsible for processing and manipulating data before it is presented to ...
Hi there! Today I'll try to explain you why the BLoC Architecture is the key to building a strong and stable app. So, we'll dive into the Presentation Layer,...
In this tutorial we'll see how to centralize your business rules into a Business Logic Layer (BLL) that serves as an intermediary for data exchange between the presentation layer and the DAL. Introduction. The Data Access Layer (DAL) created in the first tutorial cleanly separates the data access logic from the presentation logic. However ...
A Drupal theme is a collection of files that control the presentation layer, which defines how your site's content is structured and styled. Drupal supports custom themes and sub-themes (variations on an existing theme). The only required file for a theme is the .info.yml file, but most themes utilize additional files for styles, scripts, templates, and more. Location of themes Themes must be ...