

Python Conditional Assignment
When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator.
In this tutorial, we will look at different ways to assign values to a variable based on some condition.
1. Using Ternary Operator
The ternary operator is very special operator in Python, it is used to assign a value to a variable based on some condition.
It goes like this:
Here, the value of variable will be value_if_true if the condition is true, else it will be value_if_false .
Let's see a code snippet to understand it better.
You can see we have conditionally assigned a value to variable c based on the condition a > b .
2. Using if-else statement
if-else statements are the core part of any programming language, they are used to execute a block of code based on some condition.
Using an if-else statement, we can assign a value to a variable based on the condition we provide.
Here is an example of replacing the above code snippet with the if-else statement.
3. Using Logical Short Circuit Evaluation
Logical short circuit evaluation is another way using which you can assign a value to a variable conditionally.
The format of logical short circuit evaluation is:
It looks similar to ternary operator, but it is not. Here the condition and value_if_true performs logical AND operation, if both are true then the value of variable will be value_if_true , or else it will be value_if_false .
Let's see an example:
But if we make condition True but value_if_true False (or 0 or None), then the value of variable will be value_if_false .
So, you can see that the value of c is 20 even though the condition a < b is True .
So, you should be careful while using logical short circuit evaluation.
While working with lists , we often need to check if a list is empty or not, and if it is empty then we need to assign some default value to it.
Let's see how we can do it using conditional assignment.
Here, we have assigned a default value to my_list if it is empty.
Assign a value to a variable conditionally based on the presence of an element in a list.
Now you know 3 different ways to assign a value to a variable conditionally. Any of these methods can be used to assign a value when there is a condition.
The cleanest and fastest way to conditional value assignment is the ternary operator .
if-else statement is recommended to use when you have to execute a block of code based on some condition.
Happy coding! 😊
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Conditional Statements in Python
Understanding and mastering Python's conditional statements is fundamental for any programmer aspiring to write efficient and robust code. In this guide, we'll delve into the intricacies of conditional statements in Python, covering the basics, advanced techniques, and best practices.
- What are Conditional Statements?
Conditional Statements are statements in Python that provide a choice for the control flow based on a condition. It means that the control flow of the Python program will be decided based on the outcome of the condition. Now let us see how Conditional Statements are implemented in Python.
- Types of Conditional Statements in Python
1. If Conditional Statement in Python
2. if else conditional statements in python, 3. nested if..else conditional statements in python, 4. if-elif-else conditional statements in python, 5. ternary expression conditional statements in python, best practices for using conditional statements.
If the simple code of block is to be performed if the condition holds then the if statement is used. Here the condition mentioned holds then the code of the block runs otherwise not.
Syntax of If Statement :
In a conditional if Statement the additional block of code is merged as an else statement which is performed when if condition is false.
Syntax of Python If-Else :
Nested if..else means an if-else statement inside another if statement. Or in simple words first, there is an outer if statement, and inside it another if – else statement is present and such type of statement is known as nested if statement. We can use one if or else if statement inside another if or else if statements.
The if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the ladder is bypassed. If none of the conditions is true, then the final “else” statement will be executed.
The Python ternary Expression determines if a condition is true or false and then returns the appropriate value in accordance with the result. The ternary Expression is useful in cases where we need to assign a value to a variable based on a simple condition, and we want to keep our code more concise — all in just one line of code.
Syntax of Ternary Expression
- Keep conditions simple and expressive for better readability.
- Avoid deeply nested conditional blocks; refactor complex logic into smaller, more manageable functions.
- Comment on complex conditions to clarify their purpose.
- Prefer the ternary operator for simple conditional assignments.
- Advanced Techniques:
- Using short-circuit evaluation for efficiency in complex conditions.
- Leveraging the any() and all() functions with conditions applied to iterables.
- Employing conditional expressions within list comprehensions and generator expressions.
Conditional Statements in Python - FAQs
What are conditional statements in python.
Conditional statements in Python are used to execute a specific block of code based on the truth value of a condition. The most common conditional statements in Python are if , elif , and else . These allow the program to react differently depending on whether a condition (or a series of conditions) is true or false. x = 10 if x > 5: print("x is greater than 5") elif x == 5: print("x is equal to 5") else: print("x is less than 5")
What is a Conditional Expression in Python?
A conditional expression (also known as a ternary operator) in Python provides a method to simplify conditional statements. It allows for a quick definition of a condition and two possible outcomes (one if the condition is true, and one if the condition is false). The syntax is value_if_true if condition else value_if_false . # Conditional expression example x = 5 result = "High" if x > 10 else "Low" print(result) # Outputs: "Low"
What are Decision-Making Statements in Python?
Decision-making statements in Python help to control the flow of execution based on certain conditions. These include: if statement: Executes a block of code if a specified condition is true. if-else statement: Executes one block of code if the condition is true, and another block if the condition is false. elif (else if) statement: Checks multiple conditions in a sequence and executes a block of code as soon as one of the conditions evaluates to true. Nested if statements: You can nest if statements within another if or else block to create complex decision trees.
What are Conditional Selection Statements in Python?
Conditional selection statements refer to the use of if , elif , and else statements that select specific blocks of code to execute based on conditions. These statements are fundamental for branching in programming, allowing different outcomes depending on the input or state of the program.
What are the Conditional Loops in Python?
While Python supports loops that can incorporate conditions, the term "conditional loops" might specifically refer to loops that run based on a condition. Python provides two primary types of such loops: while loop: Continues to execute as long as a given condition is true. It checks the condition before executing the loop body. # while loop example x = 5 while x < 10: print(x) x += 1 for loop: Iterates over a sequence (like a list, tuple, or string) and executes the loop body for each item in the sequence. Although not traditionally a "conditional" loop, it can incorporate conditions using break and continue to control the loop execution dynamically. # for loop example with condition for i in range(10): if i == 5: break # Exit the loop when i is 5 print(i) These explanations outline how Python uses conditional statements and expressions to manage the flow of execution and make decisions within programs, crucial for creating dynamic and responsive applications
Similar Reads
Improve your coding skills with practice.
What kind of Experience do you want to share?
How to implement conditional assignment
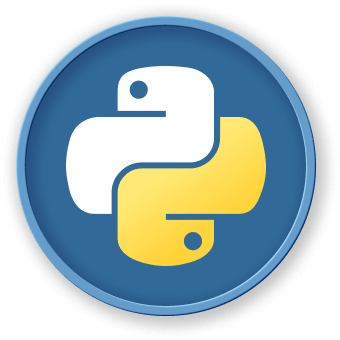
Introduction
Conditional assignment basics, python assignment techniques, real-world use cases.
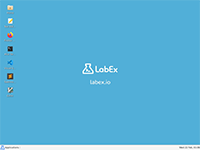
Conditional assignment is a powerful technique in Python programming that allows developers to assign values based on specific conditions. This tutorial explores various methods to implement conditional assignments efficiently, helping programmers write more concise and readable code with fewer lines of logic.
Skills Graph
Introduction to conditional assignment.
Conditional assignment is a powerful technique in Python that allows developers to assign values based on specific conditions. This approach provides a concise and readable way to handle variable assignments dynamically.
Basic Syntax and Methods
Python offers multiple ways to perform conditional assignments:
1. Ternary Operator
The ternary operator provides a compact way to assign values conditionally:

2. Logical AND/OR Operators
Python's logical operators can be used for conditional assignments:
Comparison of Conditional Assignment Techniques
Flow of conditional assignment, best practices.
- Use ternary operators for simple conditions
- Prefer explicit if-else statements for complex logic
- Ensure readability is not compromised
- Be cautious with short-circuit evaluation
LabEx Practical Tip
At LabEx, we recommend practicing conditional assignments to improve your Python programming skills and write more efficient code.
Advanced Conditional Assignment Methods
1. dictionary-based assignment.
Utilize dictionaries for complex conditional assignments:
2. Lambda Functions for Conditional Logic
Lambda functions provide flexible assignment strategies:
Conditional Assignment Patterns
3. unpacking with conditional logic.
Combine unpacking with conditional assignments:
Comparison of Assignment Techniques
Advanced conditional assignment strategies, nested conditional assignments, labex pro tip.
At LabEx, we emphasize mastering these advanced assignment techniques to write more expressive and concise Python code.
Key Takeaways
- Choose the right technique based on complexity
- Prioritize code readability
- Understand the performance implications
- Practice different conditional assignment methods
1. User Authentication and Access Control
Implement role-based access using conditional assignments:
2. E-commerce Pricing Strategy
Dynamic pricing with conditional assignments:
Conditional Assignment Flow
3. data validation and transformation.
Conditional data processing:
Practical Use Case Scenarios
4. configuration management.
Intelligent configuration selection:
LabEx Practical Insights
At LabEx, we recommend practicing these real-world conditional assignment techniques to develop robust and flexible Python applications.
- Use conditional assignments for complex logic
- Implement flexible and dynamic processing
- Prioritize code readability and maintainability
- Adapt techniques to specific use cases
By understanding and applying conditional assignment techniques in Python, developers can create more elegant and streamlined code. These methods not only improve code readability but also enhance performance by reducing complex conditional statements and providing more direct value assignments across different programming scenarios.
Other Python Tutorials you may like
- Practical Use of Inheritance
- Extensible Programs Through Inheritance
- ASCII Art Animation with OpenCV
- Calculating Laser Weapon Damage
- Factorial Calculation in Python
- Pandas DataFrame Keys Method
- Pandas DataFrame Join Method
- Pandas DataFrame Itertuples Method
- Pandas DataFrame Iterrows Method
- Pandas DataFrame Items Method

Quick Start with Python

The Advanced Python Mastery

Python Practice Labs
We use cookies for a number of reasons, such as keeping the website reliable and secure, to improve your experience on our website and to see how you interact with it. By accepting, you agree to our use of such cookies. Privacy Policy
Python One Line Conditional Assignment
Problem : How to perform one-line if conditional assignments in Python?
Example : Say, you start with the following code.
You want to set the value of x to 42 if boo is True , and do nothing otherwise.
Let’s dive into the different ways to accomplish this in Python. We start with an overview:
Exercise : Run the code. Are all outputs the same?
Next, you’ll dive into each of those methods and boost your one-liner superpower !
Method 1: Ternary Operator
The most basic ternary operator x if c else y returns expression x if the Boolean expression c evaluates to True . Otherwise, if the expression c evaluates to False , the ternary operator returns the alternative expression y .
Let’s go back to our example problem! You want to set the value of x to 42 if boo is True , and do nothing otherwise. Here’s how to do this in a single line:
While using the ternary operator works, you may wonder whether it’s possible to avoid the ...else x part for clarity of the code? In the next method, you’ll learn how!
If you need to improve your understanding of the ternary operator, watch the following video:
You can also read the related article:
- Python One Line Ternary
Method 2: Single-Line If Statement
Like in the previous method, you want to set the value of x to 42 if boo is True , and do nothing otherwise. But you don’t want to have a redundant else branch. How to do this in Python?
The solution to skip the else part of the ternary operator is surprisingly simple— use a standard if statement without else branch and write it into a single line of code :
To learn more about what you can pack into a single line, watch my tutorial video “If-Then-Else in One Line Python” :
Method 3: Ternary Tuple Syntax Hack
A shorthand form of the ternary operator is the following tuple syntax .
Syntax : You can use the tuple syntax (x, y)[c] consisting of a tuple (x, y) and a condition c enclosed in a square bracket. Here’s a more intuitive way to represent this tuple syntax.
In fact, the order of the <OnFalse> and <OnTrue> operands is just flipped when compared to the basic ternary operator. First, you have the branch that’s returned if the condition does NOT hold. Second, you run the branch that’s returned if the condition holds.
Clever! The condition boo holds so the return value passed into the x variable is the <OnTrue> branch 42 .
Don’t worry if this confuses you—you’re not alone. You can clarify the tuple syntax once and for all by studying my detailed blog article.
Related Article : Python Ternary — Tuple Syntax Hack
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills . You’ll learn about advanced Python features such as list comprehension , slicing , lambda functions , regular expressions , map and reduce functions, and slice assignments .
You’ll also learn how to:
- Leverage data structures to solve real-world problems , like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array , shape , axis , type , broadcasting , advanced indexing , slicing , sorting , searching , aggregating , and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups , negative lookaheads , escaped characters , whitespaces, character sets (and negative characters sets ), and greedy/nongreedy operators
- Understand a wide range of computer science topics , including anagrams , palindromes , supersets , permutations , factorials , prime numbers , Fibonacci numbers, obfuscation , searching , and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined , and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!

IMAGES
VIDEO
COMMENTS
There is conditional assignment in Python 2.5 and later - the syntax is not very obvious hence it's easy to miss. Here's how you do it: x = true_value if condition else false_value For further reference, check out the Python 2.5 docs.
Python Conditional Assignment. When you want to assign a value to a variable based on some condition, like if the condition is true then assign a value to the variable, else assign some other value to the variable, then you can use the conditional assignment operator. ... Let's see how we can do it using conditional assignment. my_list ...
The ternary operator in Python is a one-liner conditional expression that assigns a value based on a condition. It is written as value_if_true if condition else value_if_false. a = 5 ... A ternary is a concise way to perform a conditional assignment in a single line. In the context of programming, it typically refers to a ternary operator ...
Prefer the ternary operator for simple conditional assignments. Advanced Techniques: Using short-circuit evaluation for efficiency in complex conditions. ... the term "conditional loops" might specifically refer to loops that run based on a condition. Python provides two primary types of such loops: while loop: Continues to execute as long as a ...
Introduction to Conditional Assignment. Conditional assignment is a powerful technique in Python that allows developers to assign values based on specific conditions. This approach provides a concise and readable way to handle variable assignments dynamically. Basic Syntax and Methods. Python offers multiple ways to perform conditional ...
Problem: How to perform one-line if conditional assignments in Python?. Example: Say, you start with the following code.. x = 2 boo = True. You want to set the value of x to 42 if boo is True, and do nothing otherwise.. Let's dive into the different ways to accomplish this in Python. We start with an overview:
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
Python supports one additional decision-making entity called a conditional expression. (It is also referred to as a conditional operator or ternary operator in various places in the Python documentation.) Conditional expressions were proposed for addition to the language in PEP 308 and green-lighted by Guido in 2005.
One of the reasons why assignments are illegal in conditions is that it's easier to make a mistake and assign True or False: some_variable = 5 # This does not work # if True = some_variable: # do_something() # This only works in Python 2.x True = some_variable print True # returns 5 In Python 3 True and False are keywords, so no risk anymore.
Many programming languages have a ternary operator, which defines a conditional expression. The most common usage is to make a terse, simple dependent assignment statement. In other words, it offers a one-line code to evaluate the first expression if the condition is true; otherwise, it considers the second expression.