- Engineering Mathematics
- Discrete Mathematics
- Operating System
- Computer Networks
- Digital Logic and Design
- C Programming
- Data Structures
- Theory of Computation
- Compiler Design
- Computer Org and Architecture

Pointer Expressions in C with Examples
Prerequisite: Pointers in C Pointers are used to point to address the location of a variable. A pointer is declared by preceding the name of the pointer by an asterisk(*) . Syntax:
When we need to initialize a pointer with variable’s location, we use ampersand sign(&) before the variable name. Example:
The ampersand (&) is used to get the address of a variable. We can directly find the location of any identifier by just preceding it with an ampersand(&) sign. Example:
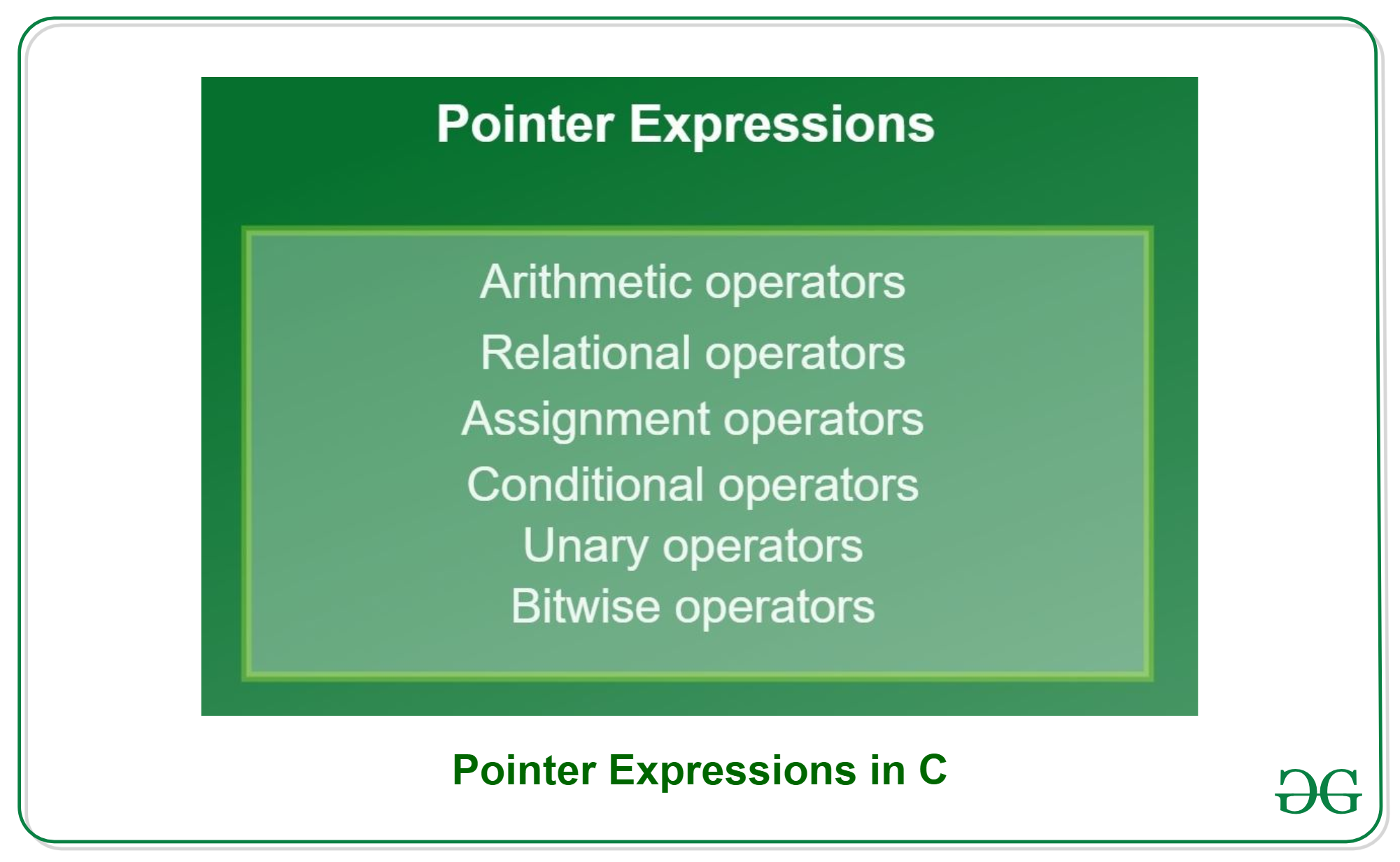
Arithmetic Operators
We can perform arithmetic operations to pointer variables using arithmetic operators. We can add an integer or subtract an integer using a pointer pointing to that integer variable. The given table shows the arithmetic operators that can be performed on pointer variables:
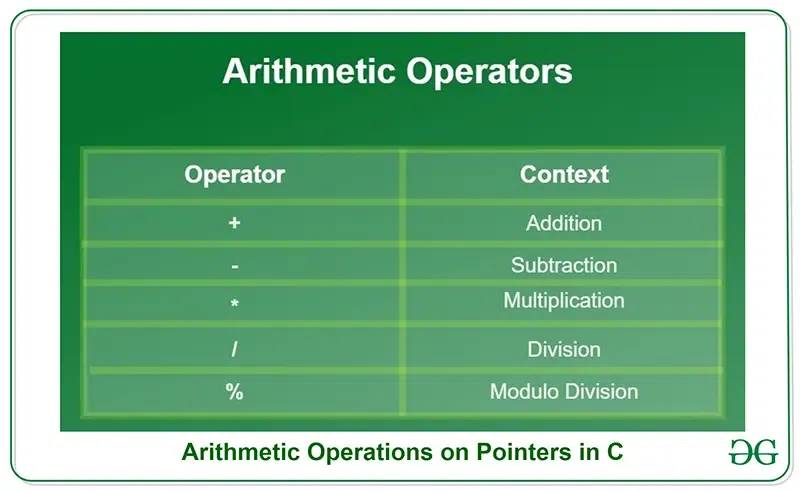
We can also directly perform arithmetic expressions on integers by dereferencing pointers. Let’s look at the example given below where p1 and p2 are pointers.
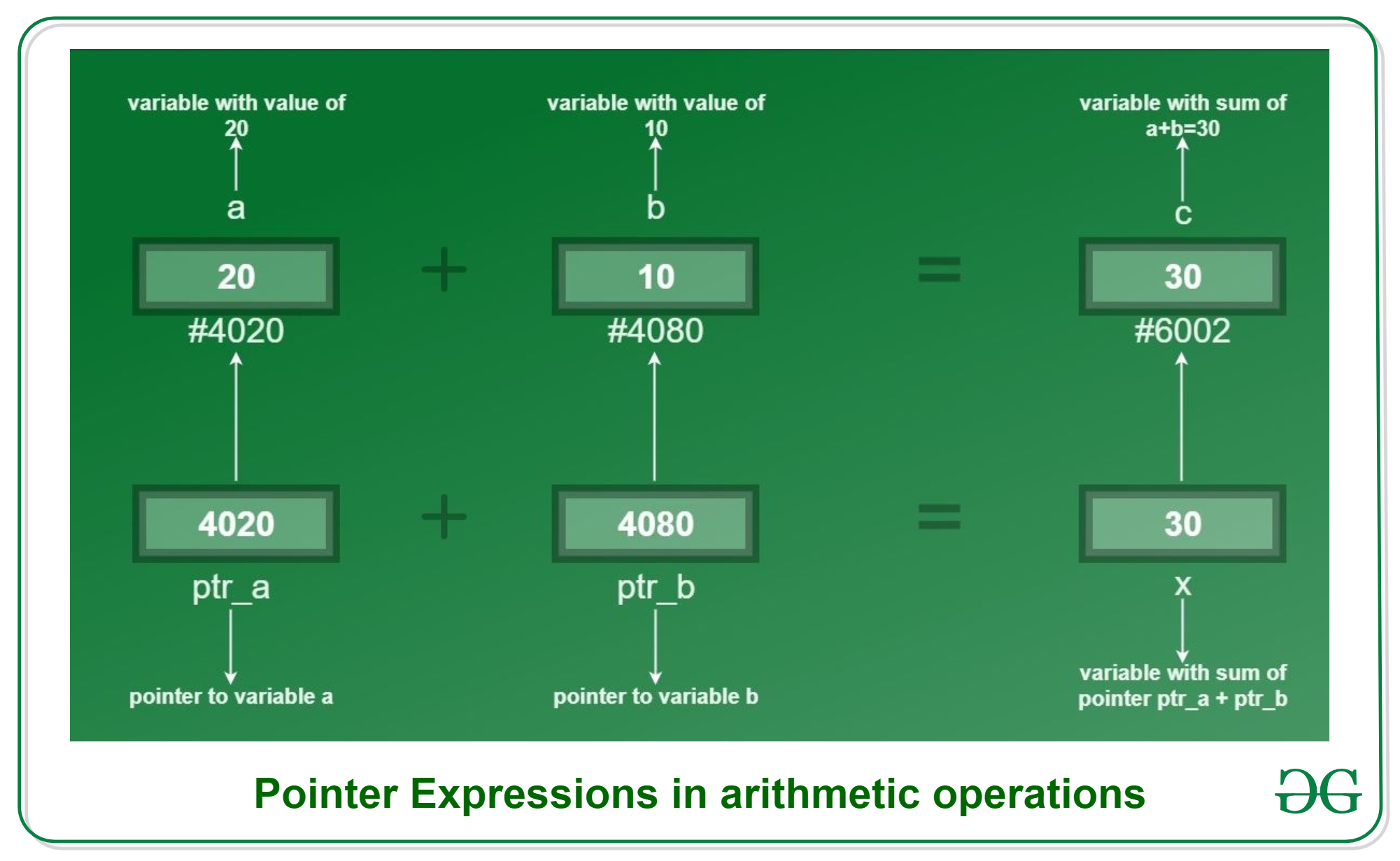
Note: While performing division, make sure you put a blank space between ‘/’ and ‘*’ of the pointer as together it would make a multi-line comment(‘/*’). Example:
Relational Operators
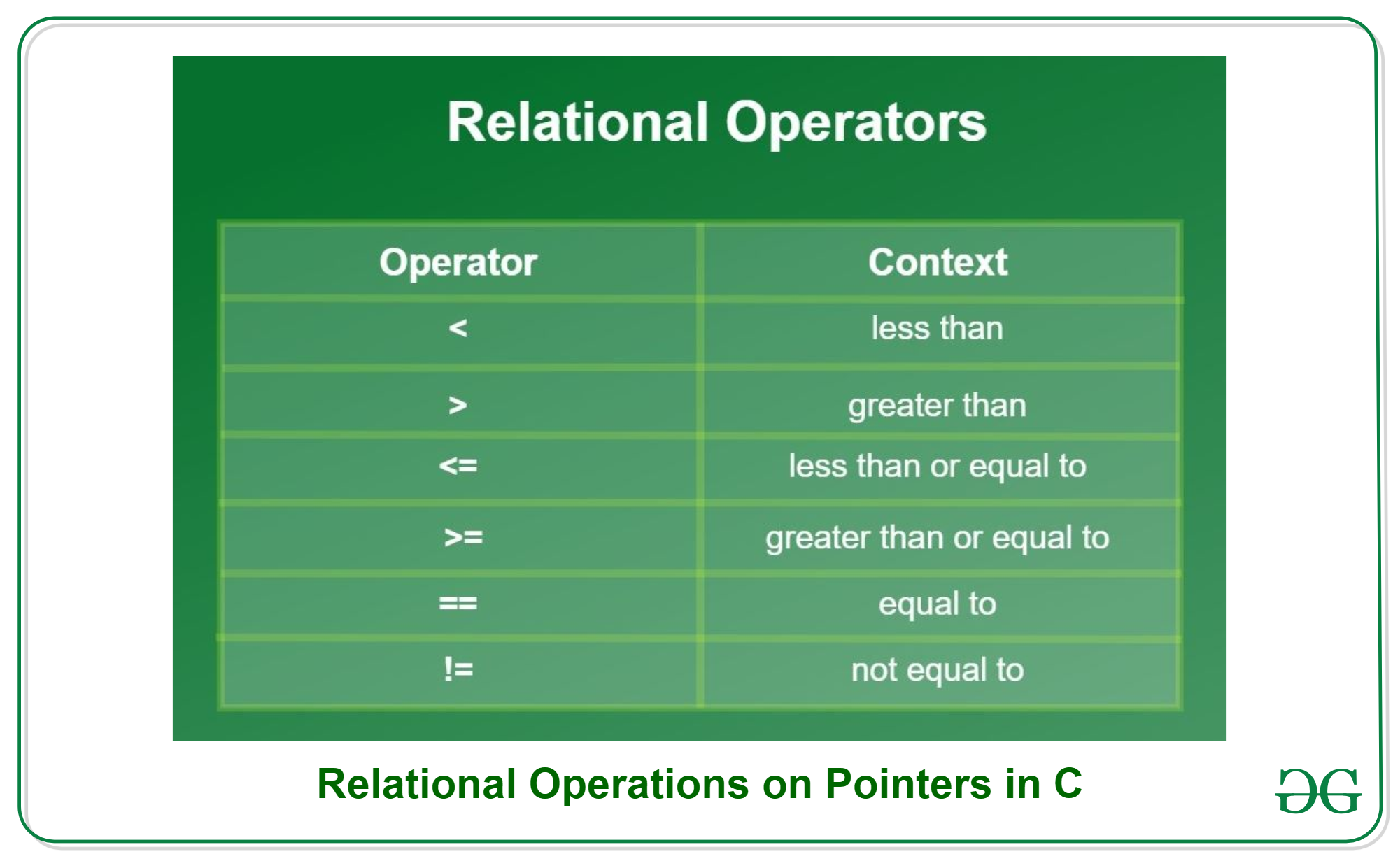
The value of the relational expression is either 0 or 1 that is false or true. The expression will return value 1 if the expression is true and it’ll return value 0 if false. Let us understand relational expression on pointer better with the code given below:
Assignment Operators
Assignment operators are used to assign values to the identifiers. There are multiple shorthand operations available. A table is given below showing the actual assignment statement with its shorthand statement.
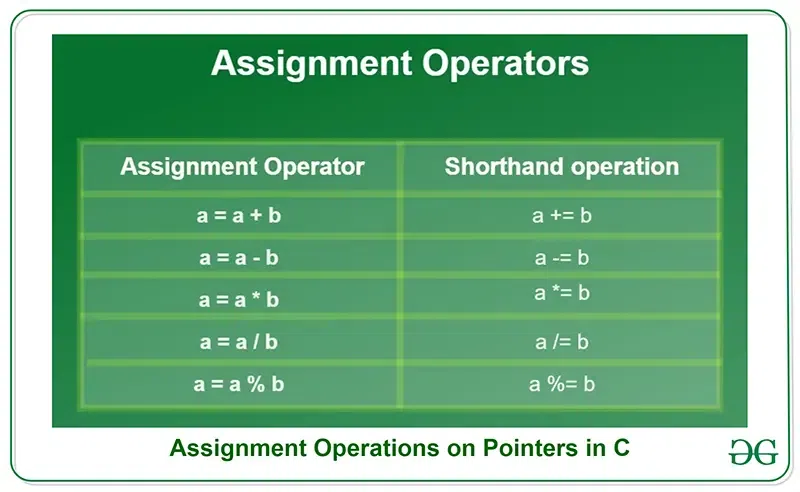
Let us understand assignment operator in better way with the help of code given below:
Conditional Operators
There is only one mostly used conditional operator in C known as Ternary operator. Ternary operator first checks the expression and depending on its return value returns true or false, which triggers/selects another expression. Syntax:
- As shown in example, assuming *ptr1=20 and *ptr2=10 then the condition here becomes true for the expression, so it’ll return value of true expression i.e. *ptr1, so variable ‘c’ will now contain value of 20.
- Considering same example, assume *ptr1=30 and *ptr2=50 then the condition is false for the expression, so it’ll return value of false expression i.e. *ptr2, so variable ‘c’ will now contain value 50.
Let us understand the concept through the given code:
Unary Operators
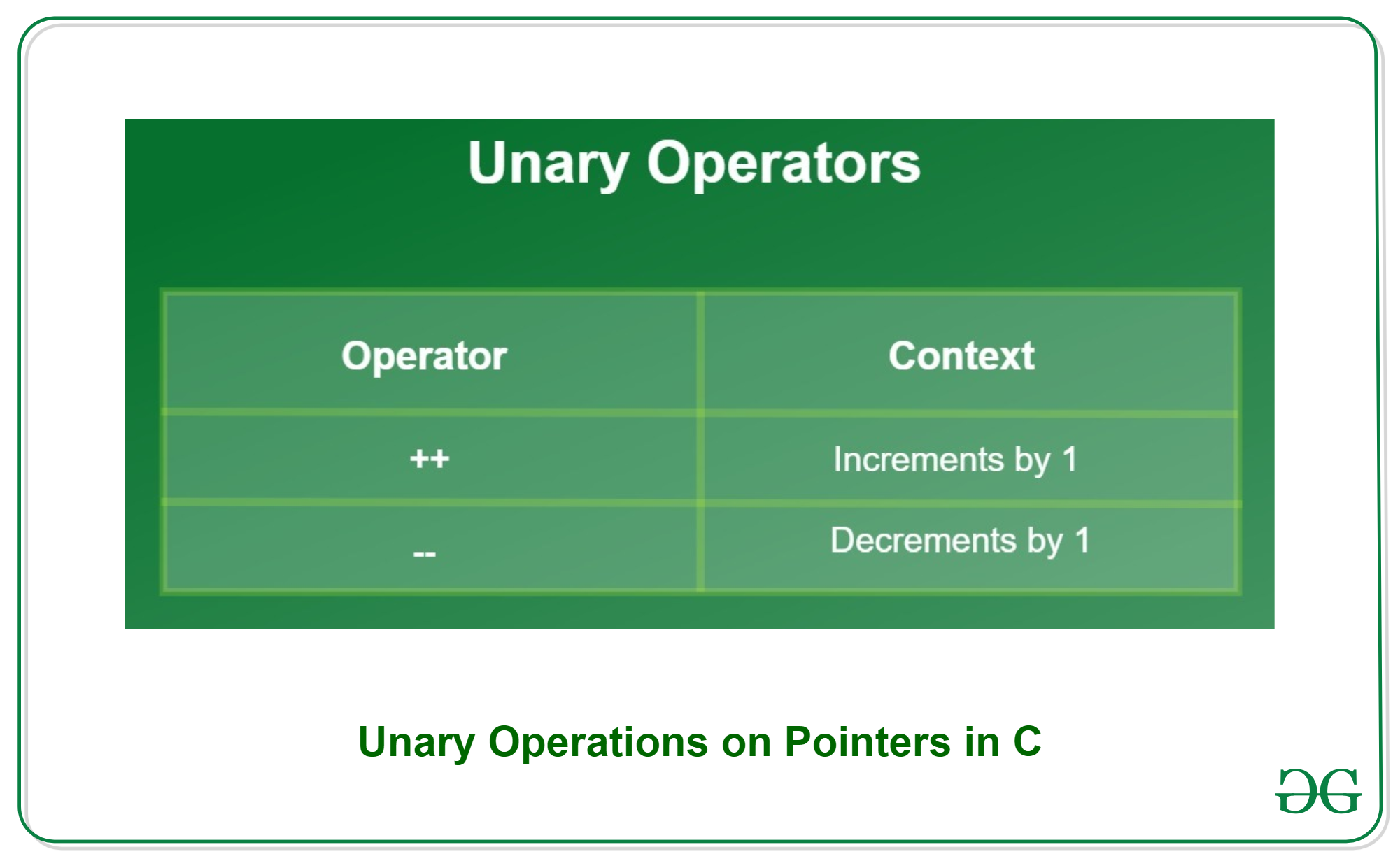
Let us understand the use of the unary operator through the given code:
Bitwise Operators
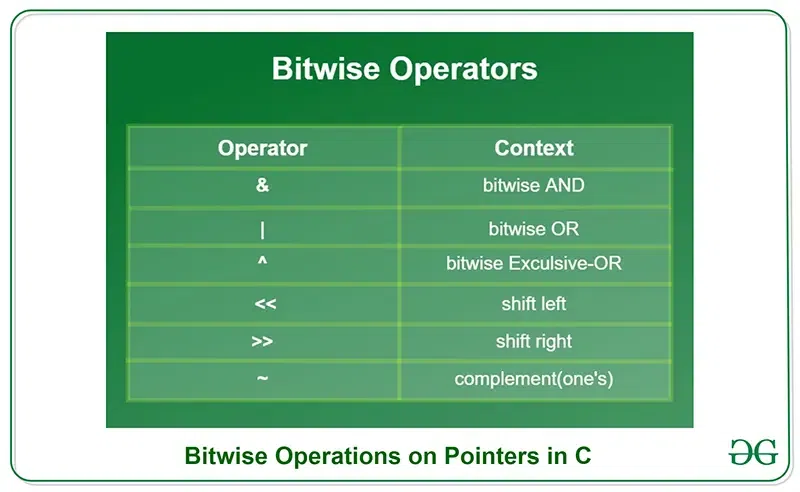
Similar Reads
Improve your coding skills with practice.
What kind of Experience do you want to share?
C Functions
C structures, c reference, creating pointers.
You learned from the previous chapter, that we can get the memory address of a variable with the reference operator & :
In the example above, &myAge is also known as a pointer .
A pointer is a variable that stores the memory address of another variable as its value.
A pointer variable points to a data type (like int ) of the same type, and is created with the * operator.
The address of the variable you are working with is assigned to the pointer:
Example explained
Create a pointer variable with the name ptr , that points to an int variable ( myAge ). Note that the type of the pointer has to match the type of the variable you're working with ( int in our example).
Use the & operator to store the memory address of the myAge variable, and assign it to the pointer.
Now, ptr holds the value of myAge 's memory address.

Dereference
In the example above, we used the pointer variable to get the memory address of a variable (used together with the & reference operator).
You can also get the value of the variable the pointer points to, by using the * operator (the dereference operator):
Note that the * sign can be confusing here, as it does two different things in our code:
- When used in declaration ( int* ptr ), it creates a pointer variable .
- When not used in declaration, it act as a dereference operator .
Good To Know: There are two ways to declare pointer variables in C:
Notes on Pointers
Pointers are one of the things that make C stand out from other programming languages, like Python and Java .
They are important in C, because they allow us to manipulate the data in the computer's memory. This can reduce the code and improve the performance. If you are familiar with data structures like lists, trees and graphs, you should know that pointers are especially useful for implementing those. And sometimes you even have to use pointers, for example when working with files and memory management .
But be careful ; pointers must be handled with care, since it is possible to damage data stored in other memory addresses.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

IMAGES
VIDEO